8.13 LAB: Library book sorting Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in Vector class (VectorLibrary vectorLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and VectorLibrary InsertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each InsertSorted() returns the number of operations the computer performs. Ex: If the input is: The Catcher in the Rye 9787543321724 J.D. Salinger the output is: Number of linked list operations: 1 Number of vector operations: 1 Which list do you think will require the most operations? Why? Main.cpp: #include "LinkedListLibrary.h" #include "VectorLibrary.h" #include "BookNode.h" #include "Book.h" #include #include using namespace std; void FillLibraries(LinkedListLibrary &linkedListLibrary, VectorLibrary &vectorLibrary) { ifstream inputFS; // File input stream int linkedListOperations = 0; int vectorOperations = 0; BookNode* currNode; Book tempBook; string bookTitle; string bookAuthor; long bookISBN; // Try to open file inputFS.open("books.txt"); while(getline(inputFS, bookTitle)) { inputFS >> bookISBN; inputFS.ignore(1, '\n'); getline(inputFS, bookAuthor); // Insert into linked list currNode = new BookNode(bookTitle, bookAuthor, bookISBN); linkedListOperations = linkedListLibrary.InsertSorted(currNode, linkedListOperations); linkedListLibrary.lastNode = currNode; // Insert into vector tempBook = Book(bookTitle, bookAuthor, bookISBN); vectorOperations = vectorLibrary.InsertSorted(tempBook, vectorOperations); } inputFS.close(); // close() may throw ios_base::failure if fails } int main (int argc, const char* argv[]) { int linkedListOperations = 0; int vectorOperations = 0; // Create libraries LinkedListLibrary linkedListLibrary = LinkedListLibrary(); VectorLibrary vectorLibrary; // Fill libraries with 100 books FillLibraries(linkedListLibrary, vectorLibrary); // Create new book to insert into libraries BookNode* currNode; Book tempBook; string bookTitle; string bookAuthor; long bookISBN; getline(cin, bookTitle); cin >> bookISBN; cin.ignore(); getline(cin, bookAuthor); // Insert into linked list // No need to delete currNode, deleted by LinkedListLibrary destructor currNode = new BookNode(bookTitle, bookAuthor, bookISBN); // TODO: Call LL_Library's InsertSorted() method to insert currNode and return // the number of operations performed linkedListLibrary.lastNode = currNode; // Insert into VectorList tempBook = Book(bookTitle, bookAuthor, bookISBN); // TODO: Call VectorLibrary's InsertSorted() method to insert currNode and return // the number of operations performed // TODO: Print number of operations for linked list // TODO: Print number of operations for vector }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
8.13 LAB: Library book sorting
Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in
Complete main() by inserting a new book into each list using the respective LinkedListLibrary and VectorLibrary InsertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each InsertSorted() returns the number of operations the computer performs.
Ex: If the input is:
The Catcher in the Rye 9787543321724 J.D. Salingerthe output is:
Number of linked list operations: 1 Number of vector operations: 1Which list do you think will require the most operations? Why?
Main.cpp:
#include "LinkedListLibrary.h"
#include "VectorLibrary.h"
#include "BookNode.h"
#include "Book.h"
#include <fstream>
#include <iostream>
using namespace std;
void FillLibraries(LinkedListLibrary &linkedListLibrary, VectorLibrary &vectorLibrary) {
ifstream inputFS; // File input stream
int linkedListOperations = 0;
int vectorOperations = 0;
BookNode* currNode;
Book tempBook;
string bookTitle;
string bookAuthor;
long bookISBN;
// Try to open file
inputFS.open("books.txt");
while(getline(inputFS, bookTitle)) {
inputFS >> bookISBN;
inputFS.ignore(1, '\n');
getline(inputFS, bookAuthor);
// Insert into linked list
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
linkedListOperations = linkedListLibrary.InsertSorted(currNode, linkedListOperations);
linkedListLibrary.lastNode = currNode;
// Insert into vector
tempBook = Book(bookTitle, bookAuthor, bookISBN);
vectorOperations = vectorLibrary.InsertSorted(tempBook, vectorOperations);
}
inputFS.close(); // close() may throw ios_base::failure if fails
}
int main (int argc, const char* argv[]) {
int linkedListOperations = 0;
int vectorOperations = 0;
// Create libraries
LinkedListLibrary linkedListLibrary = LinkedListLibrary();
VectorLibrary vectorLibrary;
// Fill libraries with 100 books
FillLibraries(linkedListLibrary, vectorLibrary);
// Create new book to insert into libraries
BookNode* currNode;
Book tempBook;
string bookTitle;
string bookAuthor;
long bookISBN;
getline(cin, bookTitle);
cin >> bookISBN;
cin.ignore();
getline(cin, bookAuthor);
// Insert into linked list
// No need to delete currNode, deleted by LinkedListLibrary destructor
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
// TODO: Call LL_Library's InsertSorted() method to insert currNode and return
// the number of operations performed
linkedListLibrary.lastNode = currNode;
// Insert into VectorList
tempBook = Book(bookTitle, bookAuthor, bookISBN);
// TODO: Call VectorLibrary's InsertSorted() method to insert currNode and return
// the number of operations performed
// TODO: Print number of operations for linked list
// TODO: Print number of operations for vector
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

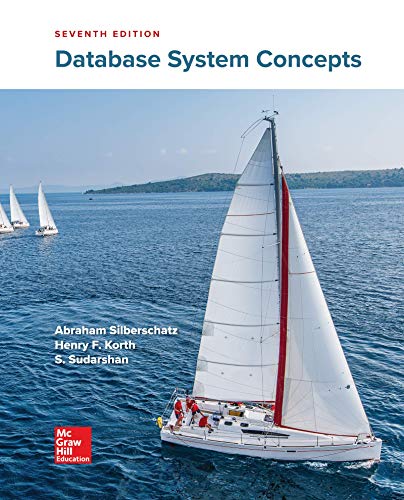
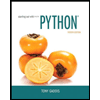
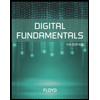
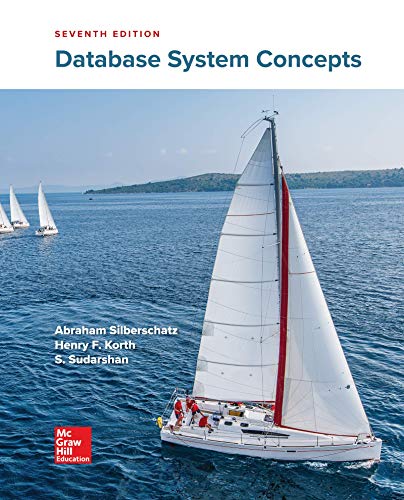
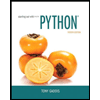
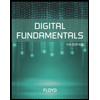
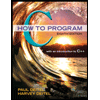
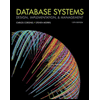
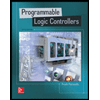