4.6.2: Recursive function: Writing the recursive case. Write code to complete print_factorial()'s recursive case. Sample output with input: 55! = 5 * 4 * 3 * 2 * 1 = 120 def print_factorial(fact_counter, fact_value): output_string = '' if fact_counter == 0: # Base case: 0! = 1 output_string += '1' elif fact_counter == 1: # Base case: print 1 and result output_string += str(fact_counter) + ' = ' + str(fact_value) else: # Recursive case output_string += str(fact_counter) + ' * ' next_counter = fact_counter - 1 next_value = next_counter * fact_value output_string += ''' Your solution goes here ''' return output_string user_val = int(input()) print('%d! = ' % user_val, end="") print(print_factorial(user_val, user_val)) what would solution be for output string above?
4.6.2: Recursive function: Writing the recursive case. Write code to complete print_factorial()'s recursive case. Sample output with input: 55! = 5 * 4 * 3 * 2 * 1 = 120 def print_factorial(fact_counter, fact_value): output_string = '' if fact_counter == 0: # Base case: 0! = 1 output_string += '1' elif fact_counter == 1: # Base case: print 1 and result output_string += str(fact_counter) + ' = ' + str(fact_value) else: # Recursive case output_string += str(fact_counter) + ' * ' next_counter = fact_counter - 1 next_value = next_counter * fact_value output_string += ''' Your solution goes here ''' return output_string user_val = int(input()) print('%d! = ' % user_val, end="") print(print_factorial(user_val, user_val)) what would solution be for output string above?
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
4.6.2: Recursive function: Writing the recursive case.
Write code to complete print_factorial()'s recursive case.
Sample output with input: 55! = 5 * 4 * 3 * 2 * 1 = 120
Sample output with input: 55! = 5 * 4 * 3 * 2 * 1 = 120
def print_factorial(fact_counter, fact_value):
output_string = ''
if fact_counter == 0: # Base case: 0! = 1
output_string += '1'
elif fact_counter == 1: # Base case: print 1 and result
output_string += str(fact_counter) + ' = ' + str(fact_value)
else: # Recursive case
output_string += str(fact_counter) + ' * '
next_counter = fact_counter - 1
next_value = next_counter * fact_value
output_string += ''' Your solution goes here '''
return output_string
user_val = int(input())
print('%d! = ' % user_val, end="")
print(print_factorial(user_val, user_val))
what would solution be for output string above?
![entry level cyber
* Home
Gradebook
zy Section 4.6 - CYB X
A python - LAB: Sor X
b Answered: 4.7.1:
* [Solved] How do
G 5.16 LAB: Cryptog X
+
i learn.zybooks.com/zybook/CYB_135_54915392/chapter/4/section/6
= zyBooks My library > CYB/135: Object-Oriented Security Scripting home > 4.6: Creating recursion
B zyBooks catalog
? Help/FAQ
Kenneth Schultz -
Feedback?
CHALLENGE
4.6.2: Recursive function: Writing the recursive case.
АCTIVITY
Write code to complete print_factorial()'s recursive case.
Sample output with input: 5
5! = 5 * 4 * 3 * 2 * 1 = 120
346682.2019644.qx3zgy7
1 def print_factorial (fact_counter, fact_value):
output_string =
1 test
passed
3
if fact counter == 0:
output_string += '1'
elif fact_counter == 1:
output_string += str(fact_counter) +
4
# Base case: 0! = 1
All tests
6
# Base case: print 1 and result
passed
+ str(fact_value)
7
8
else:
# Recursive case
output_string += str(fact_counter) +
next_counter = fact_counter
next_value = next_counter * fact_value
output_string += '' Your solution goes here '''
10
11
12
13
14
return output_string
15
16 user_val = int(input())
17 print('%d! = ' % user_val, end="")
18 print(print_factorial(user_val, user_val))
Run
Feedback?
(*factorial) In this discussion, we ignore the fact that the math module has a very convenient math.factorial(n) function.
3:09 AM
O Type here to search
77°F Cloudy
9/18/2021](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7ef9d07b-5934-4723-aca6-faf08a622511%2F1ac5061b-a3cb-4ada-91b0-139626d8f769%2F0cgqwza_processed.png&w=3840&q=75)
Transcribed Image Text:entry level cyber
* Home
Gradebook
zy Section 4.6 - CYB X
A python - LAB: Sor X
b Answered: 4.7.1:
* [Solved] How do
G 5.16 LAB: Cryptog X
+
i learn.zybooks.com/zybook/CYB_135_54915392/chapter/4/section/6
= zyBooks My library > CYB/135: Object-Oriented Security Scripting home > 4.6: Creating recursion
B zyBooks catalog
? Help/FAQ
Kenneth Schultz -
Feedback?
CHALLENGE
4.6.2: Recursive function: Writing the recursive case.
АCTIVITY
Write code to complete print_factorial()'s recursive case.
Sample output with input: 5
5! = 5 * 4 * 3 * 2 * 1 = 120
346682.2019644.qx3zgy7
1 def print_factorial (fact_counter, fact_value):
output_string =
1 test
passed
3
if fact counter == 0:
output_string += '1'
elif fact_counter == 1:
output_string += str(fact_counter) +
4
# Base case: 0! = 1
All tests
6
# Base case: print 1 and result
passed
+ str(fact_value)
7
8
else:
# Recursive case
output_string += str(fact_counter) +
next_counter = fact_counter
next_value = next_counter * fact_value
output_string += '' Your solution goes here '''
10
11
12
13
14
return output_string
15
16 user_val = int(input())
17 print('%d! = ' % user_val, end="")
18 print(print_factorial(user_val, user_val))
Run
Feedback?
(*factorial) In this discussion, we ignore the fact that the math module has a very convenient math.factorial(n) function.
3:09 AM
O Type here to search
77°F Cloudy
9/18/2021
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
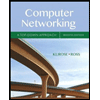
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
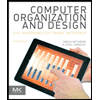
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
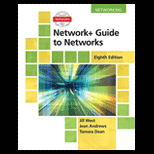
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
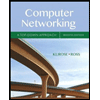
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
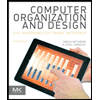
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
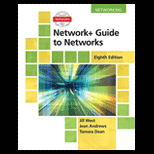
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
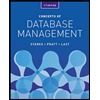
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
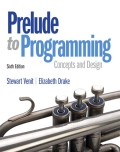
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
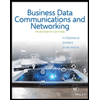
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY