3. Write a MapReduce driver program in Java for the given mapper and reducer classes that perform a word count on a data set stored in a text file. public class Map extends Mapper<LongWritable,Text,Text,IntWritable> { @Override public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] row = value.toString().split("\t"); context.write(new Text(row[2]),new IntWritable(1)); } } public class Reduce extends Reducer<Text, IntWritable,Text,IntWritable> { @Override public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int count = 0; for (IntWritable value:values) { count += value.get(); } context.write(key, new IntWritable(count)); } }
C3. Write a MapReduce driver program in Java for the given mapper and reducer classes that
perform a word count on a data set stored in a text file.
public class Map extends Mapper<LongWritable,Text,Text,IntWritable> {
@Override
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String[] row = value.toString().split("\t");
context.write(new Text(row[2]),new IntWritable(1));
}
}
public class Reduce extends Reducer<Text, IntWritable,Text,IntWritable> {
@Override
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int count = 0;
for (IntWritable value:values) {
count += value.get();
}
context.write(key, new IntWritable(count));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 13 images

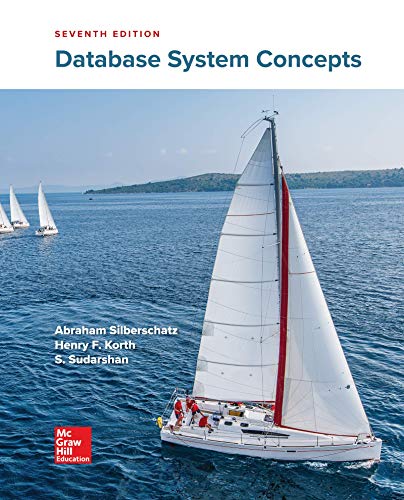
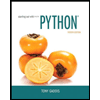
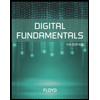
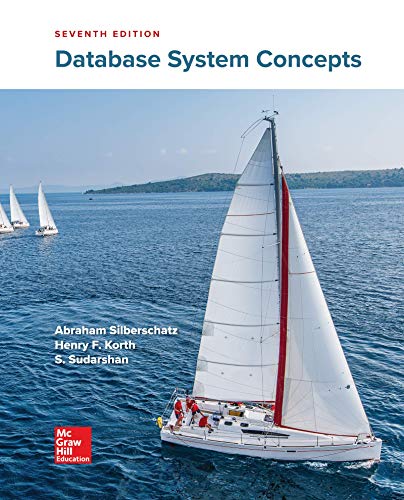
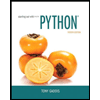
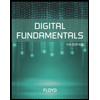
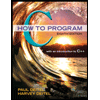
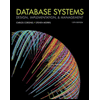
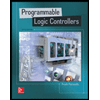