3. Construct a set of user-defined functions based on the following table. Each function must receive the relevant parameters and return the total surface area and volume of the respective geometric shape.
WHY DOES MY CODE APPEARS TO HAVE ERROR? PLEASE CHECK MY CODING (USING R PROGRAMMING)
#For Cuboid
cuboid <- function(l, w, h)
calcArea <- function(l, w, h)
{
Area <- 2*((l*w) +(l*h) +(w*h))
return(Area)
}
calcvol <- function(l, w, h)
{
vol <- l*w*h
return(vol)
}
#For cube
cube <- function(a)
calcArea <- function(a)
{
Area <- 6*a*a
return(Area)
}
calcvol <- function(a)
{
vol <- a*a*a
return(vol)
}
#For circular cylinder
cir_cylinder <- function(r, h)
calcArea <- function(r, h)
{
Area <- 2*3.14*r*(r+h)
return(Area)
}
calcvol <- function(r, h)
{
vol <- 3.14*r*r*h
return(vol)
}
#For circular cone
cir_cone <- function(s, h, r)
calcArea <- function(s, h, r)
{
Area <- 3.14*r*(r+s)
return(Area)
}
calcvol <- function(s, h, r)
{
vol <- ((3.14*r*r*h) /3)
return (vol)
}
#For Sphere
sphere <- function(r)
calcArea <- function(r)
{
Area <- 4*3.14*r*r
return(Area)
}
calcvol <- function(r)
{
vol <- (4/3) *3.14*r*r*r
return(vol)
}
#For hemisphere
hemi_sphere <- function(r)
calcArea<- function(r)
{
Area <- 3*3.14*r*r
return(Area)
}
calcvol <- function(r)
{
vol <- (2/3) *3.14*r*r*r
return(vol)
}
if(interactive()){
l<-as.numeric(readline(prompt="Enter the length (l) of the cuboid "))
w<-as.numeric(readline(prompt="Enter the width (w) of the cuboid "))
h<-as.numeric(readline(prompt="Enter the height (h) of the cuboid "))
print(paste("The area of the cuboid is ", calcArea(l, w, h)))
print(paste("The volume of the cuboid is ", calcvol(l, w, h)))
}
if(interactive()){
a<-as.numeric(readline(prompt="Enter the length (a) of the cube "))
print(paste("The area of the cube is ", calcArea(a)))
print(paste("The volume of the cube is ", calcvol(a)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the length (r) of the circular cylinder "))
h<-as.numeric(readline(prompt="Enter the height (h) of the circular cylinder "))
print(paste("The area of the circular cylinder is ", calcArea(r,h)))
print(paste("The volume of the circular cylinder is ", calcvol(r,h)))
}
if(interactive()){
s<-as.numeric(readline(prompt="Enter the length (s) of the circular cone "))
h<-as.numeric(readline(prompt="Enter the height (h) of the circular cone "))
r<-as.numeric(readline(prompt="Enter the radius (r) of the circular cone "))
print(paste("The area of the circular cone is ", calcArea(s, h, r)))
print(paste("The volume of the circular cone is ", calcvol(s, h, r)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the radius (r) of the sphere "))
print(paste("The area of the sphere is ", calcArea(r)))
print(paste("The volume of the sphere is ", calcvol(r)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the radius (r) of the hemi_sphere "))
print(paste("The area of the hemi_sphere is ", calcArea(r)))
print(paste("The volume of the hemi_sphere is ", calcvol(r)))
}



Step by step
Solved in 4 steps with 3 images

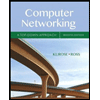
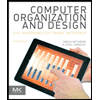
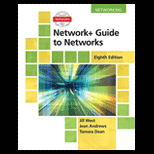
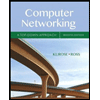
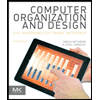
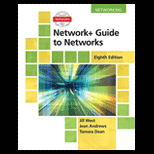
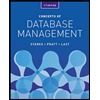
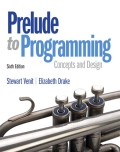
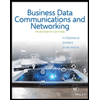