Change words so that it counts sentences. In particular, deal with the problem of mistakenly counting ..." as three sentences. For our purposes, a sentence ends in a period, an exclamation point, or a question mark, and is followed by two spaces or a newline.
Change words so that it counts sentences. In particular, deal with the problem of mistakenly counting ..." as three sentences. For our purposes, a sentence ends in a period, an exclamation point, or a question mark, and is followed by two spaces or a newline.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please help in C++

Transcribed Image Text:The image contains a C++ program with a commented task at the top, instructing to modify a word counting algorithm to count sentences instead. Specifically, the task highlights the issue of incorrectly identifying ellipses ("...") as multiple sentences. The criteria for a sentence end in this context include a period, exclamation point, or question mark that is followed by two spaces or a newline.
The code snippet, `words.cpp`, is as follows:
```cpp
#include <iostream>
using namespace std;
/**
* A state filter which counts words that
* are separated by non-alphanumeric characters.
*/
int main()
{
bool inAWord(false); // haven't read anything yet
int words(0); // so we have no words
char ch;
while (cin.get(ch))
{
if (! (isdigit(ch) || isalpha(ch))) // any non-alpha-numeric character
{
if (inAWord) // transition leaving a word
{
inAWord = false;
words++;
}
}
else
{
inAWord = true;
}
}
cout << "There were " << words << " words." << endl;
}
```
### Explanation:
- **Headers and Libraries**: The program begins by including the iostream header for input/output stream operations.
- **Namespace**: The `std` namespace is used, allowing direct access to functions and objects like `cin` and `cout`.
- **Comment Block**: A brief description mentions that the program is designed to count words separated by non-alphanumeric characters.
- **Main Function**:
- **Variables**:
- `bool inAWord(false);`: A boolean to track whether the current character reading position is inside a word.
- `int words(0);`: A counter for the number of words.
- **Input Loop**: A `while` loop reads characters from the input:
- **Character Condition**: Checks if a character is non-alphanumeric using `isdigit()` and `isalpha()`.
- **Word Transition**: If non-alphanumeric is found while `inAWord` is true, it denotes the end of a word and increments the counter.
- **Reset**: Sets `inAWord` to false, indicating the absence in a word context or true if inside a word.
- **Output**: Finally prints the count of
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
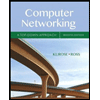
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
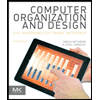
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
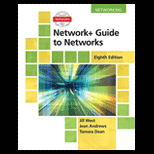
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
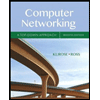
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
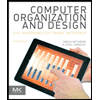
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
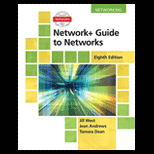
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
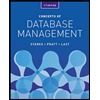
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
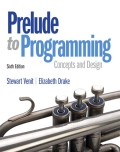
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
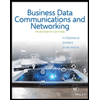
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY