2,3,4,5,6,9,4,3,2,1,12,16,19,18,11,19,18,23,21,13,16,18,19,3,4,5,6,9,4,12,16,19,18,11,19,18,23, 21,13,16,18,19,3,4,5,6,9,4,1,12,16,19,18,11,19,18,23,21,13,16,18,19,3,4,5,6,9,4,12,16,19,18,11, 19,18,23,21,13,16,18,19,3,4,5,6,9,4,4,5,6,9,4,3,2,1,12,16,19,18,11,19,18,23,21,13,16,18,19,3,4,5, 6,9,4,12,16,19,18,11,19,18,23,21,13,16,18,19,3,4,5,6,9,4,1,12,16,19,18,11,19,18,23,21,13,16,18, 19,3,4,5,6,9,4,12,16,19,18,11,19,18,23,21,13,16,18,19,3,4,5,6,9,4
Please write a C ++ program to find the median of the data in the file data.txt


- Declare the required variables like filename , median and vector.
- User enters the filename. Read the file using ifstream;
- The Amount of data present in file named input is unknown so use vector to store integer data from file.
- Open the file to read, the file is read using while loop till the end of file is not reached.
- Read data using getline based on comma separator one by one. convert the data into string then convert it to int value. Now store the int value in the vector.
- The process is repeated till the end of the file is not reaches and read data is converted to int then stored in the vector.
- close the file now.Display the vector which contains unsorted data.
- median is always found on sorted data, first sorted the vector in ascending order.
- display the sorted vector.
- find the media now. check if size of the vector is even or odd.
- if vector size is even then median is mean of 2 middle elements. size/2 and size/2-1.
- else median is the element present at size/2 index
- display the median now.
//read the input txt file store in a vector
//median is found from sorted data
//sort the vector then find median
#include <iostream>
#include <fstream>
#include <string>
#include <cstdlib>
#include <vector>
#include <bits/stdc++.h>
using namespace std;
int main()
{
//reaf txt file using ifstream
ifstream in_file;
//take user input for filename only txt file
string filename;
//storing int data read from file
vector<int> vectorOFInts;
//storing median
float median = 0.0;
//user enters filename
cout <<"\nEnter file name: ";
cin >>filename;
//we are reading text file only right now
filename = filename+".txt";
// open file to read now
in_file.open(filename.c_str());
//if file opened successfully
if(in_file){
//file opened successfully
//Read data from file now
string line;
//loop will go till end of file is not reached.
while( getline(in_file,line,',')){
//convert string to int
int data = atoi(line.c_str());
//add element in vector
vectorOFInts.push_back(data);
}
cout <<"\n close file now ..\n";
in_file.close();//close the in_file
cout<<"file is closed\n";
//display the vector elements before sorting
cout<<"**** vector before sorting **** \n";
for (int i = 0; i < vectorOFInts.size(); i++) {
cout << vectorOFInts.at(i) << ' ';
}
//WORK ON THE DATA NOW
//for finding the median data must be sorted
//sort the vector in ascending order
sort(vectorOFInts.begin(),vectorOFInts.end());
//size of vector
int totalElements = vectorOFInts.size();
//display the vector elements after sorting
cout<<"\n\n**** vector AFTER SORTING ****\n ";
for (int i = 0; i < vectorOFInts.size(); i++) {
cout << vectorOFInts.at(i) << ' ';
}
//index for getting middle index element
int half = totalElements /2;
// if size is even
if(totalElements % 2 == 0){
median = (vectorOFInts.at( half-1) + vectorOFInts.at( half))/2.0 ;
}else{//if size is odd
median = vectorOFInts.at( half) ;
}
//display the median
cout<<"\n\n MEDIAN : "<<median;
}else{
cout <<"\n UNABLE TO OPEN file ";
//signify abnormal termination
return 1;
}
return 0;
}
Step by step
Solved in 3 steps with 1 images

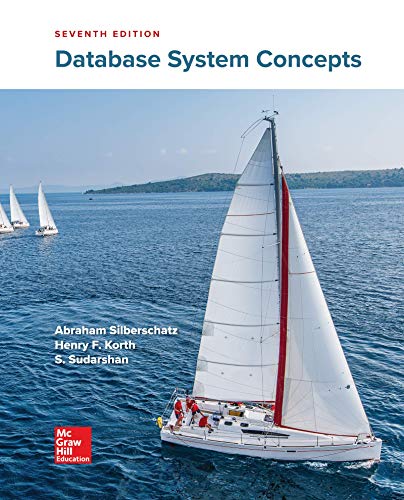
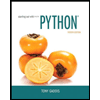
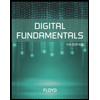
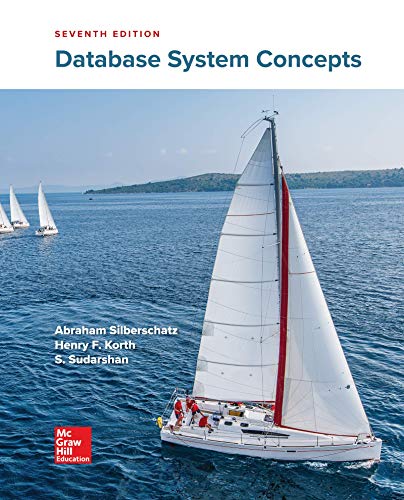
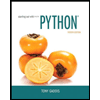
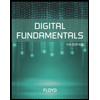
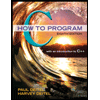
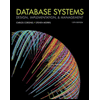
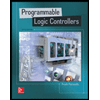