1. You are given a main() function that asks the user for two integer inputs and passes these two values to a function call of the swap() function. 2. The swap function doesn't exist yet so your task is to make its function declaration and implement its function definition. 3. Its details are the following: 1. It accepts two integer addresses as its parameters 2. It will swap the values found in the two integer addresses. For example, if we have two integer values, a = 5 and b = 10, and we pass their addresses to the function call, by the end of the function, the two integer values will be swapped. Meaning, a would now be equal to 10 and b would now be equal to 5. 4. Do not edit anything in the main() function.
1. You are given a main() function that asks the user for two integer inputs and passes these two values to a function call of the swap() function. 2. The swap function doesn't exist yet so your task is to make its function declaration and implement its function definition. 3. Its details are the following: 1. It accepts two integer addresses as its parameters 2. It will swap the values found in the two integer addresses. For example, if we have two integer values, a = 5 and b = 10, and we pass their addresses to the function call, by the end of the function, the two integer values will be swapped. Meaning, a would now be equal to 10 and b would now be equal to 5. 4. Do not edit anything in the main() function.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
hello! can you please help me with my code? i need to get the same expected output. the instructions are on the photo as well as the expected output
here's my code:
#include<stdio.h>
// TODO: Implement the function declaration of swap() here
int main(void) {
int a, b;
printf("Enter value 1: ");
scanf("%d", &a);
printf("Enter value 2: ");
scanf("%d", &b);
swap(&a, &b);
printf("\nNew value 1: %d\n", a);
printf("New value 2: %d\n", b);
return 0;
}
// TODO: Implement the function definition of swap() here

Transcribed Image Text:Instructions:
1. You are given a main() function that asks the user for two
integer inputs and passes these two values to a function call
of the swap() function.
2. The swap function doesn't exist yet so your task is to make
its function declaration and implement its function definition.
3. Its details are the following:
1. It accepts two integer addresses as its parameters
2. It will swap the values found in the two integer
addresses. For example, if we have two integer values,
a = 5 and b = 10, and we pass their addresses to the
function call, by the end of the function, the two
integer values will be swapped. Meaning, a would now
be equal to 10 and b would now be equal to 5.
4. Do not edit anything in the main() function.
Input
1. First integer value
2. Second integer value
Output
Enter value 1: 5
Enter value 2: 10
New value 1: 10
New value 2: 5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
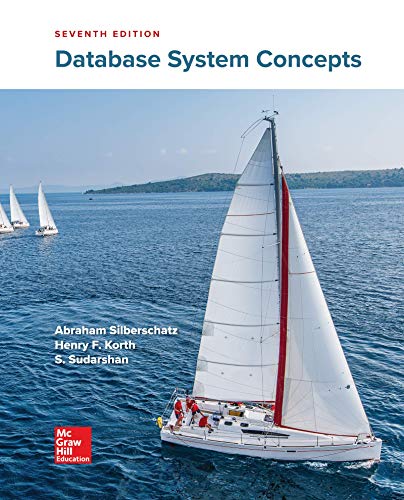
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
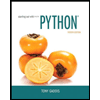
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
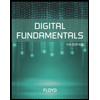
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
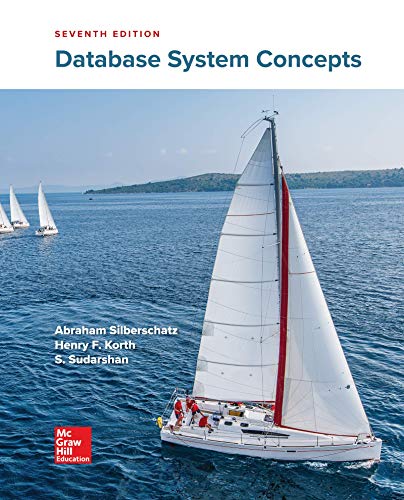
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
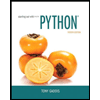
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
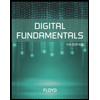
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
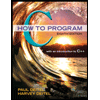
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
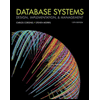
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
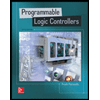
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education