1. Please correct all compile errors in the following declaration statements This program demonstrates the relationship between variables, addresses, and pointers. * / include int main (void) { * / / * int x[5] Declare and initialize variables. {1,2,3,4,5}; %3D Int *ptr=&x[0]; / * * / %p \n",x[0], &x[0] Print the variable and pointer contents. printf("x[0] ptr printf("After ptr printf("ptr* %d; address of x[0] %3D ptr + 1; ptr + 1 ******** \n"); %3D = %d; address of ptr %p \n",ptr,ptr); ptr++; printf("After ptr++ printf("ptr* ******** %d; address of ptr %p \n",*ptr,ptr); /* Exit program. * / return; }
1. Please correct all compile errors in the following declaration statements This program demonstrates the relationship between variables, addresses, and pointers. * / include int main (void) { * / / * int x[5] Declare and initialize variables. {1,2,3,4,5}; %3D Int *ptr=&x[0]; / * * / %p \n",x[0], &x[0] Print the variable and pointer contents. printf("x[0] ptr printf("After ptr printf("ptr* %d; address of x[0] %3D ptr + 1; ptr + 1 ******** \n"); %3D = %d; address of ptr %p \n",ptr,ptr); ptr++; printf("After ptr++ printf("ptr* ******** %d; address of ptr %p \n",*ptr,ptr); /* Exit program. * / return; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![```c
1. Please correct all compile errors in the following declaration statements
/*
This program demonstrates the relationship between
variables, addresses, and pointers.
*/
#include <stdio.h>
int main(void)
{
/* Declare and initialize variables. */
int x[5] = {1,2,3,4,5};
int *ptr = &x[0];
/* Print the variable and pointer contents. */
printf("x[0] = %d; address of x[0] = %p \n", x[0], &x[0]);
ptr = ptr + 1;
printf("After ptr = ptr + 1 ********\n");
printf("ptr* = %d; address of ptr = %p \n", *ptr, ptr);
ptr++;
printf("After ptr++ ********\n");
printf("ptr* = %d; address of ptr = %p \n", *ptr, ptr);
/* Exit program. */
return 0;
}
```
### Explanation of the Code
This C program demonstrates basic pointer operations.
1. It starts by declaring an array `x` with five integers.
2. A pointer `ptr` is initialized to point to the first element of the array `x`.
3. It prints the value and address of `x[0]`.
- **x[0] = %d:** This will print the integer value stored at `x[0]`.
- **address of x[0] = %p:** This will print the memory address of `x[0]`.
4. The pointer `ptr` is incremented first by adding 1, and then using post-increment (`ptr++`), to point to subsequent elements of `x`.
- After each increment, it prints:
- **ptr* = %d:** The value at the new location `ptr` points to.
- **address of ptr = %p:** The address stored in the `ptr`.
### Compile Errors
- The keyword `Int` should be `int` (case-sensitive).
- The `return;` statement should correctly be `return 0;`, indicating successful termination of `main`.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1728920-6e41-44bf-9744-ac0a64f4d19a%2F3f523f86-be22-4956-8e18-6de96417e68a%2Fig2xd9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```c
1. Please correct all compile errors in the following declaration statements
/*
This program demonstrates the relationship between
variables, addresses, and pointers.
*/
#include <stdio.h>
int main(void)
{
/* Declare and initialize variables. */
int x[5] = {1,2,3,4,5};
int *ptr = &x[0];
/* Print the variable and pointer contents. */
printf("x[0] = %d; address of x[0] = %p \n", x[0], &x[0]);
ptr = ptr + 1;
printf("After ptr = ptr + 1 ********\n");
printf("ptr* = %d; address of ptr = %p \n", *ptr, ptr);
ptr++;
printf("After ptr++ ********\n");
printf("ptr* = %d; address of ptr = %p \n", *ptr, ptr);
/* Exit program. */
return 0;
}
```
### Explanation of the Code
This C program demonstrates basic pointer operations.
1. It starts by declaring an array `x` with five integers.
2. A pointer `ptr` is initialized to point to the first element of the array `x`.
3. It prints the value and address of `x[0]`.
- **x[0] = %d:** This will print the integer value stored at `x[0]`.
- **address of x[0] = %p:** This will print the memory address of `x[0]`.
4. The pointer `ptr` is incremented first by adding 1, and then using post-increment (`ptr++`), to point to subsequent elements of `x`.
- After each increment, it prints:
- **ptr* = %d:** The value at the new location `ptr` points to.
- **address of ptr = %p:** The address stored in the `ptr`.
### Compile Errors
- The keyword `Int` should be `int` (case-sensitive).
- The `return;` statement should correctly be `return 0;`, indicating successful termination of `main`.
Expert Solution

Step 1
Here in the given code, x is an array of integers while ptr is integer pointer.
Step by step
Solved in 2 steps

Recommended textbooks for you
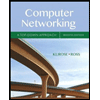
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
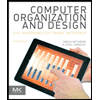
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
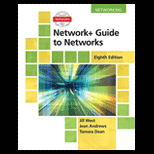
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
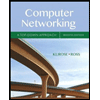
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
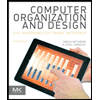
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
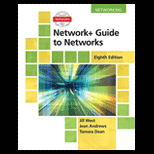
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
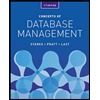
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
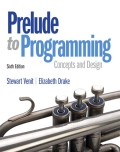
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
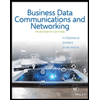
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY