1). Create a stack data structure (MUStack) given the sample class with methods below. public class MUStack<E> { private class Node<E> ( private E data; Node<E> next; public Node (E data) { this.data = data; this.next=null; } } private Node<E> top; private int count; //number of elements in the stack public MUStack() { } public E pop(){ { public void push (E data) { } public E peek() { } public int size () { } 2). Write a a program that implements the following algorithm to evaluate postfix that will use the (MUStack) Evaluation of postfix string Using StringTokenizer or split While (there are more tokens) Get next token If this token is numeric push it on the stack If it is an operator (+. -/, *) Pop the top two elements from the stack Perform the operation indicated by the operator Push the result on the stack End while The answer will on the top of the stack The program should: Ask the user to enter a space delimited postfix string or Quit If the User chooses the Quit option, terminate the program. If the user selects the other option, Ask the user to enter a space delimited postfix string Evaluate the postfix using the MUStack and the algorithm above Print the calculated value Go back to step 1
1). Create a stack data structure (MUStack) given the sample class with methods below. public class MUStack<E> { private class Node<E> ( private E data; Node<E> next; public Node (E data) { this.data = data; this.next=null; } } private Node<E> top; private int count; //number of elements in the stack public MUStack() { } public E pop(){ { public void push (E data) { } public E peek() { } public int size () { } 2). Write a a program that implements the following algorithm to evaluate postfix that will use the (MUStack) Evaluation of postfix string Using StringTokenizer or split While (there are more tokens) Get next token If this token is numeric push it on the stack If it is an operator (+. -/, *) Pop the top two elements from the stack Perform the operation indicated by the operator Push the result on the stack End while The answer will on the top of the stack The program should: Ask the user to enter a space delimited postfix string or Quit If the User chooses the Quit option, terminate the program. If the user selects the other option, Ask the user to enter a space delimited postfix string Evaluate the postfix using the MUStack and the algorithm above Print the calculated value Go back to step 1
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
1). Create a stack data structure (MUStack) given the sample class with methods below.
public class MUStack<E> {
private class Node<E> (
private E data;
Node<E> next;
public Node (E data) {
this.data = data;
this.next=null;
}
}
private Node<E> top;
private int count; //number of elements in the stack
public MUStack() {
}
public E pop(){
{
public void push (E data) {
}
public E peek() {
}
public int size () {
}
2). Write a a program that implements the following
Evaluation of postfix string
- Using StringTokenizer or split
- While (there are more tokens)
- Get next token
- If this token is numeric push it on the stack
- If it is an operator (+. -/, *)
- Pop the top two elements from the stack
- Perform the operation indicated by the operator
- Push the result on the stack
- End while
- The answer will on the top of the stack
The program should:
- Ask the user to enter a space delimited postfix string or Quit
- If the User chooses the Quit option, terminate the program.
- If the user selects the other option,
- Ask the user to enter a space delimited postfix string
- Evaluate the postfix using the MUStack and the algorithm above
- Print the calculated value
- Go back to step 1
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
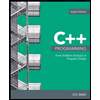
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
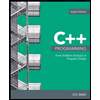
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning