ELD-3110
docx
keyboard_arrow_up
School
Thomas Edison State College *
*We aren’t endorsed by this school
Course
311
Subject
Mechanical Engineering
Date
Jan 9, 2024
Type
docx
Pages
8
Uploaded by DrJellyfish3760
Messier, Michael J (mjmessier)
ELD-3110
LAB3
Report (60 percent):
The report should list all the major procedures required to
complete each of the exercises. Here are the general guidelines for the report:
1.
Cover Sheet (5 points): The lab report must include a completed cover sheet
with your name, student ID number, the lab number, lab title, and submission
date.
Note:
Your lab report will not be graded without a completed cover
sheet.
2.
Objective (15 points): A short paragraph stating the purpose (main ideas) of
the experiment. Procedure (30 points): At the beginning of this section, give a
summary description of the procedures taken during the lab.
3.
Discussion/Conclusion (30 points): State your understanding of this
experiment. (What did you learn from these experiment?) State the
challenges and problems faced, and measures taken to resolve these
problems and overcome challenges.
4.
Arduino Sketches (20 points): At the end of the report, please append the text
of all the Arduino sketches you have developed. To do this, simply cut the
entire text from the Arduino IDE editor window and paste it in the report
document. Start on a fresh page for each sketch.
Video Demonstration (40 percent):
In this section, your video demonstration
should provide a visual record of the results obtained in each exercise. Please start
with recording a brief video clip giving your name, course number, and assignment
number. Record a video clip for each exercise according to the instructions provided.
Use a video editor to combine all the clips into one video file before you
submit/upload to the course website. For guidelines to record and merge video clips,
check
Record, Merge, and Upload Your Videos
.
Exercise 1: Toggling LEDs using IR remote
Connect three LEDs (different colors) and the IR receiver to the UNO board. The
purpose is to turn the LEDs on and off using keys on the remote. Select three
keys on the remote that you would use to toggle the LEDs. Write an Arduino
sketch to detect the keys and turn the corresponding LED on or off when the key
is pressed. The sketch should turn on all the LEDs in the setup part. In the loop
part, it will simply look for the selected keys being pressed and change the state
of the corresponding LED. This implies that you will need to store the present
current of each LED in a variable.
The video clip for this exercise should show the wiring of the experiment and the
use of the remote to toggle the three LEDs.
Exercise 2: Using a potentiometer to control the servo
Attach the small (one-sided) arm to the servo. Connect the servo and a
potentiometer to the UNO board as shown in the example. Use the
potentiometer to position the servo.
The video clip for this exercise should show the wiring of the experiment and the
changing position of the servo arm with the setting of the potentiometer.
Exercise 3: Controlling the speed of a stepper motor
Modify the test program to let the stepper motor run continuously in the
clockwise direction at about 10 RPM. This means that one full rotation should
take about 6 seconds. The motion should take place at a constant speed which
would require that the delay in each step is the same.
Experiment Report: Arduino Uno Control Experiments
Objective
The overarching objective of these experiments was to explore various control mechanisms using an
Arduino Uno board, encompassing IR remote control for LEDs, potentiometer-driven servo motor
control, and stepper motor speed regulation. The experiments aimed to showcase interfacing
capabilities, remote-controlled functionalities, and motor control mechanisms within the Arduino
ecosystem.
Exercise 1: Toggling LEDs using IR Remote
Objective
To control three LEDs through an IR remote, toggling their states (ON/OFF) using specific keys on the
remote.
Procedure
Connected three LEDs to digital pins and an IR receiver to another pin on the Arduino Uno. The code
initialized the IR receiver, set LED pins as outputs, and toggled LED states based on received IR signals.
Discussion/Conclusion
Insights into IR communication and remote-based control systems were gained. Challenges involved IR
code interpretation and verification, resolved through meticulous code validation and hardware
verification.
SKETCH:
include
<IRremote.h>
// Define the pins for LEDs
const int
redLedPin =
9
;
const int
greenLedPin =
10
;
const int
blueLedPin =
11
;
// Define the pin for the IR receiver
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
const int
receiverPin =
12
;
// Define IR receiver object
IRrecv
irrecv
(
receiverPin
)
;
decode_results results;
// Variables to store LED states
bool
redLedState = HIGH;
// Assume initially LEDs are off
bool
greenLedState = HIGH;
bool
blueLedState = HIGH;
// Replace these with the actual IR codes from your remote
#define
RED_CODE
0x
FF30CF
#define
GREEN_CODE
0x
FF18E7
#define
BLUE_CODE
0x
FF7A85
void
setup
() {
Serial
.
begin
(
9600
)
;
// Initialize Serial communication
irrecv
.
enableIRIn
()
;
// Initialize IR receiver
// Set LED pins as outputs
pinMode
(
redLedPin, OUTPUT
)
;
pinMode
(
greenLedPin, OUTPUT
)
;
pinMode
(
blueLedPin, OUTPUT
)
;
// Turn on all LEDs initially
digitalWrite
(
redLedPin, redLedState
)
;
digitalWrite
(
greenLedPin, greenLedState
)
;
digitalWrite
(
blueLedPin, blueLedState
)
;
}
void
loop
() {
if
(
irrecv
.
decode
(
&results
)) {
unsigned long
receivedCode =
results
.
value
;
// Get the received IR
code
Serial
.
println
(
receivedCode, HEX
)
;
// Print received code (for
debugging)
// Change the state of LEDs based on the received IR code
if
(
receivedCode == RED_CODE
) {
redLedState = !redLedState;
digitalWrite
(
redLedPin, redLedState
)
;
}
else if
(
receivedCode == GREEN_CODE
) {
greenLedState = !greenLedState;
digitalWrite
(
greenLedPin, greenLedState
)
;
}
else if
(
receivedCode == BLUE_CODE
) {
blueLedState = !blueLedState;
digitalWrite
(
blueLedPin, blueLedState
)
;
}
irrecv
.
resume
()
;
// Receive the next IR signal
}
}
Exercise 2: Using a Potentiometer to Control the Servo Motor
Objective
To manipulate the position of a servo motor using a potentiometer.
Procedure
Connected a servo motor and potentiometer to the Arduino Uno. Wrote code to read potentiometer
values and adjust the servo motor's position accordingly.
Discussion/Conclusion
An understanding of servo motor control via analog input was obtained. Challenges centered around
calibrating the potentiometer's range and mapping values, resolved through iterative adjustments in
code and hardware setup.
Sketch:
include
<Servo.h>
// Create a servo object
Servo myServo;
// Define the potentiometer pin
const int
potPin = A0;
void
setup
() {
// Attach the servo to pin 9
myServo
.
attach
(
9
)
;
}
void
loop
() {
// Read the value from the potentiometer
int
potValue =
analogRead
(
potPin
)
;
// Map the potentiometer value (0-1023) to the servo range (0-180)
int
servoPos =
map
(
potValue,
0
,
1023
,
0
,
180
)
;
// Move the servo to the mapped position
myServo
.
write
(
servoPos
)
;
// Add a delay to slow down the movement (optional)
delay
(
120
)
;
}
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Exercise 3: Controlling the Speed of a Stepper Motor
Objective
To regulate a stepper motor's speed to approximately 10 RPM.
Procedure
Modified the stepper motor control code, adjusting delays between steps to achieve a consistent speed.
Observed the stepper motor's rotation for speed verification.
Discussion/Conclusion
Successful control of stepper motor speed was achieved. Challenges included fine-tuning delay values for
precise speed control, addressed through iterative adjustments and speed verification tests.
Sketch:
v
#include
<
Stepper
.
h
>
// Define the number of steps per revolution
const int
stepsPerRevolution =
200
;
// Create a Stepper object
Stepper
myStepper
(
stepsPerRevolution,
8
,
10
,
9
,
11
)
;
void
setup
() {
// Set the speed for the stepper motor in RPM (revolutions per minute)
myStepper
.
setSpeed
(
30
)
;
// Adjust this value to achieve approximately
10 RPM
// To calculate delay between steps for 10 RPM
// RPM = 10
// Time for one revolution = 60 seconds / RPM = 60 / 10 = 6 seconds
// Number of steps in one revolution = stepsPerRevolution = 200
// Time for one step = Time for one revolution / Number of steps = 6
seconds / 200 = 30 milliseconds
}
void
loop
() {
// Step one step in the clockwise direction
myStepper
.
step
(
1
)
;
// Adjust the delay here if needed for smoother movement
delay
(
30
)
;
// Set the delay to the calculated value for 10 RPM
}
Overall Understanding
The experiments provided a comprehensive understanding of interfacing various components with the
Arduino Uno, ranging from IR remote control to servo and stepper motor manipulation. Challenges were
met with iterative adjustments in code and hardware setup, enhancing proficiency in Arduino-based
control systems.
Related Documents
Related Questions
I need parts 1, 2, and 3 answered pertaining to the print provided.
NOTE: If you refuse to answers all 3 parts and insist on wasting my question, then just leave it for someone else to answer. I've never had an issue until recently one single tutor just refuses to even read the instructions of the question and just denies it for a false reasons or drags on 1 part into multiple parts for no reason.
arrow_forward
I need answers to questions 7, 8, and 9 pertaining to the print provided.
Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.
arrow_forward
This is an engineering problem and not a writing assignment. Please Do Not Reject. I had other engineering tutors on bartleby help me with problems similar to this one.
This problem must be presented in a logical order showing the necessary steps used to arrive at an answer. Each homework problem should have the following items unless otherwise stated in the problem:
a. Known: State briefly what is known about the problem.
b. Schematic: Draw a schematic of the physical system or control volume.
c. Assumptions: List all necessary assumptions used to complete the problem.
d. Properties: Identify the source of property values not given to you in the problem. Most sources will be from a table in the textbook (i.e. Table A-4).
e. Find: State what must be found.
f. Analysis: Start your analysis with any necessary equations. Develop your analysis as completely as possible before inserting values and performing the calculations. Draw a box around your answers and include units and follow an…
arrow_forward
I need parts 8, 9, and 10 answered. Number 1 is an example of how it should be answered.
NOTE: Read the instructions, no where does it say any drawing is required. It is really frustrating when I wait all this time for an answer to a question and some tutor does even read the instructions and just declines it...its ridicilous.
arrow_forward
I need help solving these 3 simple parts, if you can not answer all 3 parts then please leave it for another tutor, thank you.
arrow_forward
I need answers to problems 7, 8, and 9.
NOTE: Please stop wasting my time and yours by rejecting my question because it DOES NOT REQUIRE YOU TO DRAW anything at all. They are simple questions pertaining to the print provided. READ THE INSTRUCTIONS of the assignment before you just reject it for a FALSE reason or leave it for someone to answer that actually wants to do their job. Thanks.
arrow_forward
TOPIC: ENGINEERING ECONOMICS
SPECIFIC INSTRUCTION: Solve each problem NEATLY and SYSTEMATICALLY. Show your
COMPLETE solutions and BOX your final answers. Express all your answers in 2 decimal places.
PROBLEM:
Cardinal Financing lent an engineering company Php 500,000 to retrofit an environmentally
unfriendly building. The loan is for 5 years at 10 % per year simple interest. How much money
will the firm repay at the end of 5 years?
arrow_forward
please help solve A-F. thank you
You are an engineer working on a project and your prototype has failed prematurely. You question whether or not a key component of the prototype was manufactured with the correct material. There are two way to check for the material properties. The first way is to have a material certification done to confirm the exact material composition. This will take some time. The second method to confirm the material properties is to make an ASTM test sample and test for the material properties. This tensile test was completed on a test sample with an initial diameter of .501” and an initial length of 2”. The Load-Deflection data for this tensile test is below. Use this data to answer the first set of questions on the Final Exam in eLearning. A. Determine the Ultimate Tensile Strength B. Determine the 0.2% Offset Yield Strength C. Determine the value of the Proportional Limit D. Determine the Modulus of Elasticity E. Determine the Strain at Yield F. Calculate %…
arrow_forward
Full Answer PLEASE!.
Answer Q 3 and 4 PLease
Don't copy from the internet. PLEASE WRITE YOUR OWN WORDS
THANK YOU
arrow_forward
Please double check before rejecting this question. If it needs to be rejected, please explain why as I cannot see how this is a breach of the honor code.
This is a questions from the previous year's exam at my university in Engineering Science. I have submitted a solution given to us for study purposes as proof that I will not be graded on this assessment.
In this solution, the formula (2gh)1/2 is used to find the solution, but I am on familliar with Bernoulli's Principle (P=1/2pv^2+pgh), and I was not able to find a solution using this. My solution incurred an error when I found that I had two unkown variables left that I could not break down in any meaningful way. (Velocity being the desired variable, Pressure being the problematic variable). Pressure = Force x Area, but I don't know enough about the dimensions of the tank or tap to be able to understand this.
Thank you for your help.
arrow_forward
I need problems 6 and 7 solved.
I got it solved on 2 different occasions and it is not worded correctly.
NOTE: Problem 1 is an example of how it should be answered. Below are 2 seperate links to same question asked and once again it was not answered correctly. 1. https://www.bartleby.com/questions-and-answers/it-vivch-print-reading-for-industry-228-class-date-name-review-activity-112-for-each-local-note-or-c/cadc3f7b-2c2f-4471-842b-5a84bf505857
2. https://www.bartleby.com/questions-and-answers/it-vivch-print-reading-for-industry-228-class-date-name-review-activity-112-for-each-local-note-or-c/bd5390f0-3eb6-41ff-81e2-8675809dfab1
arrow_forward
I need answers to questions 1, 2, and 3 pertaining to the print provided.
Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.
arrow_forward
Please make the charts for the questions. Please refer to Successful Project Management (7th Edition). Attached is the example
Thank you.
arrow_forward
I need answers for problems 13, 14, and 15 pertaining to the print provided.
NOTE: If you refuse to answers all 3 parts and insist on wasting my question by breaking down 1 simple question into 3 parts, then just leave it for someone else to answer. Thank you.
arrow_forward
Need help with this
arrow_forward
Help!!! Answer all parts correctly!! Please
arrow_forward
operations research - pert cpm
arrow_forward
I need these two parts answered (Multiple Choice). If you can not answer all two parts please leave it for another tutor to answer. Thank you.
What size paper was used for the original version of this print? (Multiple Choice)ABCD
The two views near the bottom of the print are called detail views. Which one of theviews above (left side, front, or right side) shows the same geometry, but at the normal 1:1scale? (Multiple Choice)a. left sideb. frontc. right side
The major diameter (100 mm) of this part is interrupted by a flat surface on top. Is an auxiliary view required to show the true size and shape of that flat surface? (Multiple Choice)a. Yesb. No
arrow_forward
Josh and Jake are both helping to
build a brick wall which is 6 meters in
height. They lay 250 bricks each, but
Josh finishes this task in three (3)
hours while Jake requires 4.5 hours
to complete his part. select the BEST
response below:
Jake does more work than Josh
O Josh does more work than Jake
Both Josh and Jake do the same amo
O of work and have the same amount of
power
Both Josh and Jake does the same
O amount of work, however, Josh has m
power than Jake.
arrow_forward
I need answers to questions 13, 14, and 15 pertaining to the print provided.
Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.
arrow_forward
Put together a short presentation (pitch) for a mechanical engineering student, emphasizing his period of the course (10th) and his most significant achievements such as his change from the naval to mechanical course, the learning of programming languages focused on data analysis (Python and SQL), since the engineering course does not explore these skills, and the improvement of Excel with the VBA language.
arrow_forward
i need the answer quickly
arrow_forward
Please answer the 4th question
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
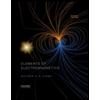
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
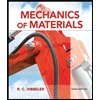
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
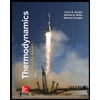
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
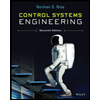
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
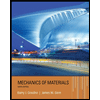
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
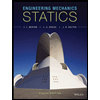
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- I need parts 1, 2, and 3 answered pertaining to the print provided. NOTE: If you refuse to answers all 3 parts and insist on wasting my question, then just leave it for someone else to answer. I've never had an issue until recently one single tutor just refuses to even read the instructions of the question and just denies it for a false reasons or drags on 1 part into multiple parts for no reason.arrow_forwardI need answers to questions 7, 8, and 9 pertaining to the print provided. Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.arrow_forwardThis is an engineering problem and not a writing assignment. Please Do Not Reject. I had other engineering tutors on bartleby help me with problems similar to this one. This problem must be presented in a logical order showing the necessary steps used to arrive at an answer. Each homework problem should have the following items unless otherwise stated in the problem: a. Known: State briefly what is known about the problem. b. Schematic: Draw a schematic of the physical system or control volume. c. Assumptions: List all necessary assumptions used to complete the problem. d. Properties: Identify the source of property values not given to you in the problem. Most sources will be from a table in the textbook (i.e. Table A-4). e. Find: State what must be found. f. Analysis: Start your analysis with any necessary equations. Develop your analysis as completely as possible before inserting values and performing the calculations. Draw a box around your answers and include units and follow an…arrow_forward
- I need parts 8, 9, and 10 answered. Number 1 is an example of how it should be answered. NOTE: Read the instructions, no where does it say any drawing is required. It is really frustrating when I wait all this time for an answer to a question and some tutor does even read the instructions and just declines it...its ridicilous.arrow_forwardI need help solving these 3 simple parts, if you can not answer all 3 parts then please leave it for another tutor, thank you.arrow_forwardI need answers to problems 7, 8, and 9. NOTE: Please stop wasting my time and yours by rejecting my question because it DOES NOT REQUIRE YOU TO DRAW anything at all. They are simple questions pertaining to the print provided. READ THE INSTRUCTIONS of the assignment before you just reject it for a FALSE reason or leave it for someone to answer that actually wants to do their job. Thanks.arrow_forward
- TOPIC: ENGINEERING ECONOMICS SPECIFIC INSTRUCTION: Solve each problem NEATLY and SYSTEMATICALLY. Show your COMPLETE solutions and BOX your final answers. Express all your answers in 2 decimal places. PROBLEM: Cardinal Financing lent an engineering company Php 500,000 to retrofit an environmentally unfriendly building. The loan is for 5 years at 10 % per year simple interest. How much money will the firm repay at the end of 5 years?arrow_forwardplease help solve A-F. thank you You are an engineer working on a project and your prototype has failed prematurely. You question whether or not a key component of the prototype was manufactured with the correct material. There are two way to check for the material properties. The first way is to have a material certification done to confirm the exact material composition. This will take some time. The second method to confirm the material properties is to make an ASTM test sample and test for the material properties. This tensile test was completed on a test sample with an initial diameter of .501” and an initial length of 2”. The Load-Deflection data for this tensile test is below. Use this data to answer the first set of questions on the Final Exam in eLearning. A. Determine the Ultimate Tensile Strength B. Determine the 0.2% Offset Yield Strength C. Determine the value of the Proportional Limit D. Determine the Modulus of Elasticity E. Determine the Strain at Yield F. Calculate %…arrow_forwardFull Answer PLEASE!. Answer Q 3 and 4 PLease Don't copy from the internet. PLEASE WRITE YOUR OWN WORDS THANK YOUarrow_forward
- Please double check before rejecting this question. If it needs to be rejected, please explain why as I cannot see how this is a breach of the honor code. This is a questions from the previous year's exam at my university in Engineering Science. I have submitted a solution given to us for study purposes as proof that I will not be graded on this assessment. In this solution, the formula (2gh)1/2 is used to find the solution, but I am on familliar with Bernoulli's Principle (P=1/2pv^2+pgh), and I was not able to find a solution using this. My solution incurred an error when I found that I had two unkown variables left that I could not break down in any meaningful way. (Velocity being the desired variable, Pressure being the problematic variable). Pressure = Force x Area, but I don't know enough about the dimensions of the tank or tap to be able to understand this. Thank you for your help.arrow_forwardI need problems 6 and 7 solved. I got it solved on 2 different occasions and it is not worded correctly. NOTE: Problem 1 is an example of how it should be answered. Below are 2 seperate links to same question asked and once again it was not answered correctly. 1. https://www.bartleby.com/questions-and-answers/it-vivch-print-reading-for-industry-228-class-date-name-review-activity-112-for-each-local-note-or-c/cadc3f7b-2c2f-4471-842b-5a84bf505857 2. https://www.bartleby.com/questions-and-answers/it-vivch-print-reading-for-industry-228-class-date-name-review-activity-112-for-each-local-note-or-c/bd5390f0-3eb6-41ff-81e2-8675809dfab1arrow_forwardI need answers to questions 1, 2, and 3 pertaining to the print provided. Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
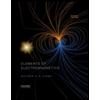
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
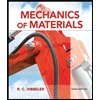
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
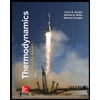
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
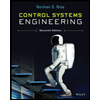
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
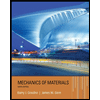
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
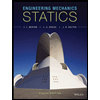
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY