HW-4_EulersMethod
pdf
keyboard_arrow_up
School
University of Michigan *
*We aren’t endorsed by this school
Course
240
Subject
Mechanical Engineering
Date
Apr 3, 2024
Type
Pages
4
Uploaded by DrSummerDolphin39
Implementing Euler's Method in MATLAB
The below live script can be editted and filled in to complete the MATLAB problem on Homework 4. There are only five lines below with ???'s that need to be editted for the code to run properly.
%Please put your name here:
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%% ADD YOUR CODE HERE TO REPLACE THE ???'s %%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
name = 'Geralynn McAdans'
;
fprintf(
'Created by: %s'
, name)
Created by: Geralynn McAdans
Preamble
%Begin by clearing the workspace:
clear; close all
; clc;
%Our given variables are:
t0 = 0; %Initial time at which we see the train
tf = 60; %Final time to simulate to
x0 = 1000; %The initial distance of the train from the station when we first spot it (in m
%Setup figure for plotting to have a hold so multiple plots can be made on
%the same figure
figure(1)
hold on
Euler's Method (Numerical Approximation)
Because we are going to be estimating the differential equation using a number of different step sizes, let us automate the process using a for loop.
%Step size scenarios to loop through and evaluate
stepSizes = [10, 5, 3, 1, 0.5];
for i = 1:length(stepSizes)
%Step size (delta) to consider in loop i.
delta = stepSizes(i);
%Create a time vector (t) from t0 to tf with a uniform step size of delta
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%% ADD YOUR CODE HERE TO REPLACE THE ???'s %%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
t = 0:stepSizes(i):60;
%Create a position vector (x) the same size as our time vector to be 1
%filled in while implementing Euler's method (this is known as
%preallocating a vector and is good coding practice since our vector
%will now not grow with each loop of j below which can be inefficient)
x = zeros(size(t));
%Assign the initial position condition to the position vector (in
%meters)
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%% ADD YOUR CODE HERE TO REPLACE THE ???'s %%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
x(1) = 1000*exp (-.1);
%Loop through the length of x to evaluate each position using Euler's Method
for j = 2:length(x) %We start at j=2 since the initial condition was already enforced
%Using the equation provided in the problem statement for Euler's
%Method we can approximate x(j) as:
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%% ADD YOUR CODE HERE TO REPLACE THE ???'s %%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
x(j) = 1000*exp(-.1*j);
end
%Add the step size condition to the plot
plot(t, x)
end
Exact Solution for Reference
Let us plot the exact solution as a point of reference so we can tell if your Euler method is behaving as expected
t_exact = linspace(t0, tf, 1e6); %Create a time vector to evaluate the exact solution over t
x_exact = 1000*exp(-0.1*t_exact); %Evaluate the exact position of the train at each time poin
plot(t_exact, x_exact, 'Linewidth'
, 2) %Plot x vs t for the exact solution with a line
xlabel(
'Time (s)'
) %X-axis label
ylabel(
'Train Distance from the Station (m)'
) %Y-axis label
title(
'Euler''s Method Example'
) %Plot title
hold on %Hold the plot so we can add lines to it as we %Now add a legend to your plot so we know which lines are which
legendVec = [cellstr(num2str(stepSizes', 'Step Size = %0.1f'
)); 'Exact Solution'
];
legend(legendVec)
2
Conclusion
Do you make the train? Explain why or why not.
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%% ADD YOUR CODE HERE TO REPLACE THE ???'s %%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
disp(
'Yes I made the train because as seen in the graph above it takes about 30 sec'
)
Yes I made the train because as seen in the graph above it takes about 37 sec
Export PDF
Click "Export to PDF" on the top left of the screen to export this file to a PDF for submission.
3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
4
Related Documents
Related Questions
please write a matlab code
arrow_forward
Use MATLAB please make code for this.
arrow_forward
Please follow the instructions and the requirements according to the pictures above and I kinda need the solution quickly. The language of the code is in Matlab, thank you in advance.
arrow_forward
Could you please fix my code it’s supposed to look like the graph that’s on the picture. But the lines do not cross eachother at the beginning. Could you make the lines look like the lines on the graph?
Use this code in MATLAB and fix it.
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Define your seed here
seed = 50;
rand('seed',seed); % Set the seed for reproducibility
% Assumed CO2 emissions for Diesel and Petrol
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves with a reduced degree of 2
pDiesel = polyfit(carPosition, CO2Diesel, 2);
pPetrol = polyfit(carPosition, CO2Petrol, 2);
% Generate points for best fit lines
fitDiesel = polyval(pDiesel, carPosition);
fitPetrol = polyval(pPetrol, carPosition);
% Plotting the data
figure;
hold on;
% Plot Diesel best fit line…
arrow_forward
I need help with the first part and Matlab for this problem
arrow_forward
This code keeps on generating graphs with different curves. The picture that you see two different graphs comes from the same code but both of them have different curves. I need the curve to look like the picture that only has one graph. I basically need the line to have a slight curve and every time I run the code it will come up as the same graph every time. Use this code on MATLAB and fix it
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Use the 'seed' function instead of 'rng'
seed = 50; % Define your seed here
rand('seed',seed);
% Assumed CO2 emissions for Diesel and Petrol
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves with a reduced degree of 2
pDiesel = polyfit(carPosition, CO2Diesel, 2);
pPetrol = polyfit(carPosition, CO2Petrol, 2);
% Generate points for best fit…
arrow_forward
There is a small space between the orange and purple line could you please connect the two lines together also can you please make the purple line shorter and then connect the purple line to the orange line, please take out the box that says “Diesel, petrol, Diesel best fit, petrol best fit”. Also when ever I run this code the graph shows up but there are still errors that comes up could you please fix them when you are running this on MATLAB.
Please use this code on MATLAB and fix it.
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Fix the random seed for reproducibility
rng(50);
% Assumed CO2 emissions for Diesel and Petrol
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves
pDiesel = polyfit(carPosition, CO2Diesel, 3);
pPetrol = polyfit(carPosition, CO2Petrol, 3);
% Generate…
arrow_forward
Don't Use Chat GPT Will Upvote And Give Solution In 30 Minutes Please
arrow_forward
Create one Simulink embedded function model to simulate the bungee jumper’s distance (x)
vs. t, the velocity (x’) vs. t and acceleration (x’’) vs. t for the first 500 seconds of the jump.
arrow_forward
Hi I need help to make the line change into a different color, I half of the line to be orange and I need the other half of the line towards the end to be purple as shown in the picture. Also I need there be a box saying Diesel, petrol, diesel best fit, petrol best fit. This part is also shown in the graph.
Please use this code and fix it in MATLAB:
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Fix the random seed for reproducibility
rng(50);
% Assumed positions of cars
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves
pDiesel = polyfit(carPosition, CO2Diesel, 3);
pPetrol = polyfit(carPosition, CO2Petrol, 3);
% Generate points for best fit lines
fitDiesel = polyval(pDiesel, carPosition);
fitPetrol = polyval(pPetrol, carPosition);
% Combine the best fit lines
combinedFit =…
arrow_forward
I having a problem with my code in MATLAB. In the following code results for r is just a 1x3 matrix. Although inside the while loop r equals to multiple 1x3 matrices. I need r equal to one matrix that is of size 27032x3. So, I just need the multiple r matrices to merge together to get one big matrix. I need that matrix to retain its value outside the while loop as well.
cc=0; % set line counter
JD = 2460626.666667;
fid = fopen('tle_catalog.txt'); % load the TLE
tline2='gg';
while ischar(tline2)
cc = cc+1; % counter
name = fgets(fid);% for the ones with three lines
tline1 = fgets(fid); % collect first line of two line elements
tline2 = fgets(fid); % collect second line of two line elements
if tline2>0 % stop at the end of the file
% initialize the propagation
[satrec, startmfe, stopmfe, deltamin] ...
= twoline2rv(721, tline1, tline2, 'c', 'd');
time_JD = tline1(21:32);
yeardayhour = str2double(regexp(tline1, '(\d{2})(\d{3})(\.\d+)', 'tokens', 'once'));
dn =…
arrow_forward
I’m making the graph that you see in the picture but the code that I’m using makes the line with to many curves. Could you make the lines look like the one that you see on the graph. Don’t change the color just make it with a little bit less curves like you see in the picture.
Use this code on MATLAB and fix it.
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Fix the random seed for reproducibility
rng(50);
% Assumed CO2 emissions for Diesel and Petrol
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves
pDiesel = polyfit(carPosition, CO2Diesel, 3);
pPetrol = polyfit(carPosition, CO2Petrol, 3);
% Generate points for best fit lines
fitDiesel = polyval(pDiesel, carPosition);
fitPetrol = polyval(pPetrol, carPosition);
% Combined best fit
combinedFit = (fitDiesel + fitPetrol) / 2;…
arrow_forward
Hello I’m trying to make the graph that you see in the picture, I’m trying the exact copy of that graph using this code but I’m having a hard time doing that. Could you change the code so that it looks like the graph that you see on the picture using MATLAB, please send the code when you are finished.
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Fix the random seed for reproducibility
rng(45);
% Assumed positions of cars
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves
pDiesel = polyfit(carPosition, CO2Diesel, 3);
pPetrol = polyfit(carPosition, CO2Petrol, 3);
% Generate points for best fit lines
fitDiesel = polyval(pDiesel, carPosition);
fitPetrol = polyval(pPetrol, carPosition);
% Plotting the data
figure; hold on;
scatter(carPosition, CO2Diesel, 'o', 'MarkerEdgeColor', [1 0.5…
arrow_forward
I’m using this code in MATLAB but for some odd reason every time I run it on MATLAB I keep on getting a different graphs. In the picture that shows two different graphs are from the same code, but I need to it to look like the picture that has one graph. Could you please fix it. To make it look like the picture that has one graph?
Here is the code:
% Sample data for Diesel and Petrol
carPosition = linspace(1, 60, 50); % Assumed positions of cars
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves
pDiesel = polyfit(carPosition, CO2Diesel, 3);
pPetrol = polyfit(carPosition, CO2Petrol, 3);
% Generate points for best fit lines
fitDiesel = polyval(pDiesel, carPosition);
fitPetrol = polyval(pPetrol, carPosition);
% Plotting the data
figure;
hold on;
scatter(carPosition, CO2Diesel, 'o', 'MarkerEdgeColor', [1 0.5 0]); % Diesel data…
arrow_forward
The picture that has two graphs are generated by this code. Every time I run it on MATLAB it keeps generating graphs with different curves. The picture that shows one graph is the curve that I want to keep. Please keep the color of the lines and the circles and keep the title of the graph the same. I want everything to be the same except I want the line to look exactly like the picture with one graph on it.
Use this code on MATLAB and fix it and then send the correct code back please.
% Sample data for Diesel and Petrol cars
carPosition = linspace(1, 60, 50); % Assumed positions of cars
% Use the 'seed' function instead of 'rng'
seed = 50; % Define your seed here
rand('seed',seed);
% Assumed CO2 emissions for Diesel and Petrol
CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel
CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol
% Fit polynomial curves with a reduced degree of 2
pDiesel = polyfit(carPosition,…
arrow_forward
HW Matlab 1) Create a variable ftemp to store a temperature in degrees Fahrenheit (F). Write m-file to convert this to degrees Celsius and store the result in a variable ctemp. The conversion factor is C = (F —32) * 5/9. 2) Write m-file to generate a matrix of random integers of size 100 by 100 their values between 15 to 80. 3) Free fall of objects is given by y =5mgt? where a is the acceleration, v is the velocity, y is the distance, m is the mass of the object, g is the gravitational acceleration. Plot the distance and velocity of the object for 15 seconds after its fall from rest (y = 0). Take m = 0.2 kg.
arrow_forward
Don't Use Chat GPT Will Upvote And Give Solution In 30 Minutes Please
arrow_forward
Matlab coding
arrow_forward
1)
Model the following problem with integer programming. The marketing department
has been allocated a budget of $10M and would like to determine which products to develop
advertising campaigns; note: at most one campaign can be launched for each product. Their goal
is to maximize revenue for the company. Data is presented in the table below.
Product
A
BCD E F
10
7
12 8 6
Projected
Revenue ($M)
Cost ($M)
6
1 2 5 4 3
arrow_forward
I want to run the SGP4 propagator for the ISS (ID = 25544) I got from spacetrack.org in MATLAB. I don't know where to get the inputs of the function. Where do I get the inFile and outFile that is mentioned in the following function.
% Purpose:
% This program shows how a Matlab program can call the Astrodynamic Standard libraries to propagate
% satellites to the requested time using SGP4 method.
%
% The program reads in user's input and output files. The program generates an
% ephemeris of position and velocity for each satellite read in. In addition, the program
% also generates other sets of orbital elements such as osculating Keplerian elements,
% mean Keplerian elements, latitude/longitude/height/pos, and nodal period/apogee/perigee/pos.
% Totally, the program prints results to five different output files.
%
%
% Usage: Sgp4Prop(inFile, outFile)
% inFile : File contains TLEs and 6P-Card (which controls start, stop times and step size)
% outFile : Base name for five output files
%…
arrow_forward
MATLAB...Hand written plzzzz asap....FAST PLZZZZZZZZZZZ
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
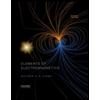
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
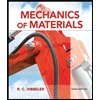
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
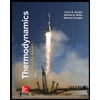
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
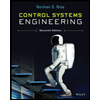
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
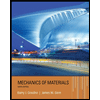
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
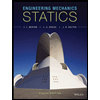
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- Could you please fix my code it’s supposed to look like the graph that’s on the picture. But the lines do not cross eachother at the beginning. Could you make the lines look like the lines on the graph? Use this code in MATLAB and fix it. % Sample data for Diesel and Petrol cars carPosition = linspace(1, 60, 50); % Assumed positions of cars % Define your seed here seed = 50; rand('seed',seed); % Set the seed for reproducibility % Assumed CO2 emissions for Diesel and Petrol CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol % Fit polynomial curves with a reduced degree of 2 pDiesel = polyfit(carPosition, CO2Diesel, 2); pPetrol = polyfit(carPosition, CO2Petrol, 2); % Generate points for best fit lines fitDiesel = polyval(pDiesel, carPosition); fitPetrol = polyval(pPetrol, carPosition); % Plotting the data figure; hold on; % Plot Diesel best fit line…arrow_forwardI need help with the first part and Matlab for this problemarrow_forwardThis code keeps on generating graphs with different curves. The picture that you see two different graphs comes from the same code but both of them have different curves. I need the curve to look like the picture that only has one graph. I basically need the line to have a slight curve and every time I run the code it will come up as the same graph every time. Use this code on MATLAB and fix it % Sample data for Diesel and Petrol cars carPosition = linspace(1, 60, 50); % Assumed positions of cars % Use the 'seed' function instead of 'rng' seed = 50; % Define your seed here rand('seed',seed); % Assumed CO2 emissions for Diesel and Petrol CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol % Fit polynomial curves with a reduced degree of 2 pDiesel = polyfit(carPosition, CO2Diesel, 2); pPetrol = polyfit(carPosition, CO2Petrol, 2); % Generate points for best fit…arrow_forward
- There is a small space between the orange and purple line could you please connect the two lines together also can you please make the purple line shorter and then connect the purple line to the orange line, please take out the box that says “Diesel, petrol, Diesel best fit, petrol best fit”. Also when ever I run this code the graph shows up but there are still errors that comes up could you please fix them when you are running this on MATLAB. Please use this code on MATLAB and fix it. % Sample data for Diesel and Petrol cars carPosition = linspace(1, 60, 50); % Assumed positions of cars % Fix the random seed for reproducibility rng(50); % Assumed CO2 emissions for Diesel and Petrol CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol % Fit polynomial curves pDiesel = polyfit(carPosition, CO2Diesel, 3); pPetrol = polyfit(carPosition, CO2Petrol, 3); % Generate…arrow_forwardDon't Use Chat GPT Will Upvote And Give Solution In 30 Minutes Pleasearrow_forwardCreate one Simulink embedded function model to simulate the bungee jumper’s distance (x) vs. t, the velocity (x’) vs. t and acceleration (x’’) vs. t for the first 500 seconds of the jump.arrow_forward
- Hi I need help to make the line change into a different color, I half of the line to be orange and I need the other half of the line towards the end to be purple as shown in the picture. Also I need there be a box saying Diesel, petrol, diesel best fit, petrol best fit. This part is also shown in the graph. Please use this code and fix it in MATLAB: % Sample data for Diesel and Petrol cars carPosition = linspace(1, 60, 50); % Assumed positions of cars % Fix the random seed for reproducibility rng(50); % Assumed positions of cars CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol % Fit polynomial curves pDiesel = polyfit(carPosition, CO2Diesel, 3); pPetrol = polyfit(carPosition, CO2Petrol, 3); % Generate points for best fit lines fitDiesel = polyval(pDiesel, carPosition); fitPetrol = polyval(pPetrol, carPosition); % Combine the best fit lines combinedFit =…arrow_forwardI having a problem with my code in MATLAB. In the following code results for r is just a 1x3 matrix. Although inside the while loop r equals to multiple 1x3 matrices. I need r equal to one matrix that is of size 27032x3. So, I just need the multiple r matrices to merge together to get one big matrix. I need that matrix to retain its value outside the while loop as well. cc=0; % set line counter JD = 2460626.666667; fid = fopen('tle_catalog.txt'); % load the TLE tline2='gg'; while ischar(tline2) cc = cc+1; % counter name = fgets(fid);% for the ones with three lines tline1 = fgets(fid); % collect first line of two line elements tline2 = fgets(fid); % collect second line of two line elements if tline2>0 % stop at the end of the file % initialize the propagation [satrec, startmfe, stopmfe, deltamin] ... = twoline2rv(721, tline1, tline2, 'c', 'd'); time_JD = tline1(21:32); yeardayhour = str2double(regexp(tline1, '(\d{2})(\d{3})(\.\d+)', 'tokens', 'once')); dn =…arrow_forwardI’m making the graph that you see in the picture but the code that I’m using makes the line with to many curves. Could you make the lines look like the one that you see on the graph. Don’t change the color just make it with a little bit less curves like you see in the picture. Use this code on MATLAB and fix it. % Sample data for Diesel and Petrol cars carPosition = linspace(1, 60, 50); % Assumed positions of cars % Fix the random seed for reproducibility rng(50); % Assumed CO2 emissions for Diesel and Petrol CO2Diesel = 25 + 5*cos(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Diesel CO2Petrol = 20 + 5*sin(carPosition/60*2*pi) + randn(1, 50)*5; % Random data for Petrol % Fit polynomial curves pDiesel = polyfit(carPosition, CO2Diesel, 3); pPetrol = polyfit(carPosition, CO2Petrol, 3); % Generate points for best fit lines fitDiesel = polyval(pDiesel, carPosition); fitPetrol = polyval(pPetrol, carPosition); % Combined best fit combinedFit = (fitDiesel + fitPetrol) / 2;…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
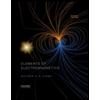
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
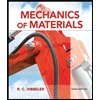
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
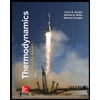
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
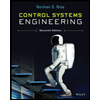
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
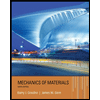
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
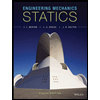
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY