control_flow_exercises
pdf
keyboard_arrow_up
School
University of Massachusetts, Boston *
*We aren’t endorsed by this school
Course
110
Subject
Electrical Engineering
Date
Jan 9, 2024
Type
Pages
3
Uploaded by HighnessThunderCobra34
Control Flow
1
Exercises
Exercise 1.
Consider the following code fragment:
if
m
>=
1
and
m
<=
5:
stdio.write(
"Spring
"
)
elif
m
>=
6
and
m
<=
8:
stdio.write(
"Summer
"
)
else
:
stdio.write(
"Fall
"
)
stdio.writeln(y)
What does the program write when
m
and
y
take on the following values?
a.
m = 10
and
y = 2020
b.
m = 5
and
y = 2021
c.
m = 6
and
y = 2022
Exercise 2.
What does the following code fragment write?
i
=
9
while
i
>=
0:
stdio.writeln(
str
(i)
+
"
"
+
str
(2
**
i))
i
-=
2
Exercise 3.
What are the arithmetic progressions returned by the following calles to
range()
?
a.
range(-5)
b.
range(5)
c.
range(3, 10)
d.
range(3, 10, 2)
e.
range(5, -5, -1)
Exercise 4.
What does the following code fragment write?
for
i
in
range
(3,
40,
4):
if
i
%
5
==
0:
stdio.writeln(i)
Exercise 5.
What does the following code fragment write?
i
=
1
for
c
in
"hello"
:
stdio.writeln(c
*
i)
i
+=
1
Exercise 6.
What does the following code fragment write?
for
i
in
range
(5):
for
j
in
range
(6):
if
j
==
5:
stdio.writeln(i
+
j)
else
:
stdio.write(
str
(i
+
j)
+
"-"
)
1 / 3
Control Flow
Exercise 7.
Implement a program called
genaralizedharmonic.py
that accepts
n
(int) and
r
(int) as command-line arguments
and writes the value of the generalized harmonic number
H
(
n, r
) to standard output, computed using the formula
H
(
n, r
) =
1
1
r
+
1
2
r
+
1
3
r
+
· · ·
+
1
n
r
.
Exercise 8.
Implement a program called
matrix.py
that accepts
n
(int) and
k
(int) as command-line arguments and writes
an
n
×
n
matrix in which the elements below the main diagonal are all zeros and the rest of the elements have the value
k
.
The elements of the matrix must be separated by a single space and each row must end with a newline character at the end.
~/workspace/ipp/programs
python
matrix.py
5
2
2
2
2
2
2
0
2
2
2
2
0
0
2
2
2
0
0
0
2
2
0
0
0
0
2
_
Exercise 9.
Consider the program
gambler.py
.
a. How many variables does the program define?
b. Write down the names of the variables and the scope of each variable.
2
Solutions
Solution 1.
a.
Fall 2020
b.
Spring 2021
c.
Summer 2022
Solution 2.
9
512
7
128
5
32
3
8
1
2
_
Solution 3.
a.
[]
b.
[0, 1, 2, 3, 4]
c.
[3, 4, 5, 6, 7, 8, 9]
d.
[3, 5, 7, 9]
e.
[5, 4, 3, 2, 1, 0, -1, -2, -3, -4]
2 / 3
Control Flow
Solution 4.
15
35
Solution 5.
h
ee
lll
llll
ooooo
Solution 6.
0-1-2-3-4-5
1-2-3-4-5-6
2-3-4-5-6-7
3-4-5-6-7-8
4-5-6-7-8-9
Solution 7.
generalizedharmonic.py
import
stdio
import
sys
n
=
int
(sys.argv [1])
r
=
int
(sys.argv [2])
total
=
0
for
i
in
range
(1,
n
+
1):
total
+=
1
/
i
**
r
stdio.writeln(total)
Solution 8.
matrix.py
import
stdio
import
sys
n
=
int
(sys.argv [1])
k
=
int
(sys.argv [2])
for
i
in
range
(n):
for
j
in
range
(n):
e
=
0
if
i
>
j
else
k
if
j
==
n
-
1:
stdio.writeln(e)
else
:
stdio.write(
str
(e)
+
"
"
)
Solution 9.
a. There are seven variables defined in
gambler.py
.
b. Here are their names and scopes:
Variable
Scope
stake
lines 9 — 25
goal
lines 10 — 25
trials
lines 11 — 25
bets
lines 12 — 25
wins
lines 13 — 25
t
lines 14 — 23
cash
lines 15 — 23
3 / 3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Related Questions
3. Discussion:
1. Convert the gray code 01011001 to decimal number and show your work.
2. Convert the gray code 00101101 to binary number and show your work.
3. Design a 8-bit binary to gray code conversion circuit using logic gates and verify your design.
4. Design a 8-bit gray to binary code conversion circuit using logic gates and verify your design.
arrow_forward
so we were asked to implement a 3-bit BCD number on DE0’s board segment display for quartus... using 7447 but 7447 has 4 inputs? (see attached screenshot for problem) also not sure what the items in the second screenshot should be doing? like i can put inputs and outputs..but i don't know what they are? and its not discussed other than they can supply power?
arrow_forward
PLEASE HELP WITH PARTS A,B,C
arrow_forward
Explain simply!
arrow_forward
Give solution of Q4 (its all parts)
Subject Digital Logic Design
arrow_forward
Design a combinatorial circuit that will be able to detect prime numbers given as input in binary. The input to the system will be any 4 bit binary number. The output of the circuit should be a single bit that will be 1 (high) if the input is a prime number and 0 (low) if the input is not a prime number. For example, if the input to the circuit is 0101 the output should be 1. Again if the input is 1010, the output should be 0.
arrow_forward
A 12-bit D/A converter has VREF = 5.12 V. What is the output voltage for a binary input code of (101010101010)? What is VLSB? What is the size of the MSB?
arrow_forward
Up/Down State Machine
Cousider a state machine implementation of a two bit up/down counter
mput: up/doun, and two outputs: Outo and Out,, which also indicate the next state. When up/dowTI
is high, the counter counts up (00,01,10,11,00, ). When up/doun is low, the counter counts down
(00,11.10.01.00, ..).
The state machine has one
Part A Complete the state diagram below by adding all required transition arcs with input annotations.
Output annotations are not required since they correspond to the new state.
state
state
00
01
state
state
10
11
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
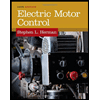
Related Questions
- 3. Discussion: 1. Convert the gray code 01011001 to decimal number and show your work. 2. Convert the gray code 00101101 to binary number and show your work. 3. Design a 8-bit binary to gray code conversion circuit using logic gates and verify your design. 4. Design a 8-bit gray to binary code conversion circuit using logic gates and verify your design.arrow_forwardso we were asked to implement a 3-bit BCD number on DE0’s board segment display for quartus... using 7447 but 7447 has 4 inputs? (see attached screenshot for problem) also not sure what the items in the second screenshot should be doing? like i can put inputs and outputs..but i don't know what they are? and its not discussed other than they can supply power?arrow_forwardPLEASE HELP WITH PARTS A,B,Carrow_forward
- Explain simply!arrow_forwardGive solution of Q4 (its all parts) Subject Digital Logic Designarrow_forwardDesign a combinatorial circuit that will be able to detect prime numbers given as input in binary. The input to the system will be any 4 bit binary number. The output of the circuit should be a single bit that will be 1 (high) if the input is a prime number and 0 (low) if the input is not a prime number. For example, if the input to the circuit is 0101 the output should be 1. Again if the input is 1010, the output should be 0.arrow_forward
- A 12-bit D/A converter has VREF = 5.12 V. What is the output voltage for a binary input code of (101010101010)? What is VLSB? What is the size of the MSB?arrow_forwardUp/Down State Machine Cousider a state machine implementation of a two bit up/down counter mput: up/doun, and two outputs: Outo and Out,, which also indicate the next state. When up/dowTI is high, the counter counts up (00,01,10,11,00, ). When up/doun is low, the counter counts down (00,11.10.01.00, ..). The state machine has one Part A Complete the state diagram below by adding all required transition arcs with input annotations. Output annotations are not required since they correspond to the new state. state state 00 01 state state 10 11arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
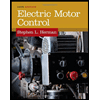