Lab 9 HDL Tutorial 1
docx
keyboard_arrow_up
School
Seneca College *
*We aren’t endorsed by this school
Course
3103
Subject
Electrical Engineering
Date
Dec 6, 2023
Type
docx
Pages
6
Uploaded by henokghebremedhin
Programmable Logic Devices
With the
Altera Cyclone IV FPGA
Lab 9: Verilog Tutorial 1 - Combinational
Students use the Quartus II IDE to experiment
with the Verilog Hardware Description Language.
Verilog HDL code if run and the results compared
to those experienced with schematic entry.
Equipment
-
Quartus II with ModelSIM and USB Blaster
-
DE0-Nano Development Board
-
USB Mini B Cable
Introduction to Verilog Hardware Description Language – Part 1
Abridged from Mano and Kime
Introduction
The first lab activity you performed explained how to design digital circuits on an FPGA using schematic
entry on the Quartus II IDE. Laboratory activity 6 repeated this exercise using Verilog as the source. In the
first case you generated a Block Design File (.
bdf
) called
IntroTutorial.bdf.
You added pins and compiled
that to generate a netlist which you saved as a SRAM Object File (.sof) called
IntroTutorial.sof
. This was
used to configure (or program) the FPGA by loading the .sof file into static ram, or by converting the .sof file
to a JTAG Indirect Configuration File (.jic) to load into flash memory.
The sixth lab repeated all of that to generate the .sof file, but from a .v file not from the .bdf file. You used
Verilog to generate
VerilogIntro.v
which was subsequently compiled to get the
VerilogIntro.sof
file to
program the FPGA. The process was the same, the only difference is that you arrived at the .sof file from
a .v file rather than a .bdf file because you used verilog to describe the circuit rather than a circuit diagram.
In this activity we’ll generate more Verilog circuit description files and compare them to the hardware
equivalent. Keep in mind that even though you might use programming constructs, you’re not programming.
You are describing. You are either describing the way the circuit is configured, describing how the circuit
behaves, or describing how the data flows through the circuit. In any case, you are not programming. Always
keep this in mind so that your coded description reflects circuit operation.
You can either simulate the operation of the code or load it into your FPGA to confirm it’s operation.
1.0
New Project
Open a NEW PROJECT and call it Lab9. The circuit described here will change as you load new Verilog
files, but you don’t need a new project for each. For each section, code is provided along with some
explanation. You are asked to examine the code, and either simulate or load and operate it on the FPGA in
order to complete a truth table or draw circuit diagram to explain it’s operation.
1.1
Lab9Example1
Examine the code following and answer the following questions.
a)
What is the name of the module?
________________
(From Line 3)
b)
How many ports does it have?
________________
(From Line 3)
c)
How many inputs does it have?
________________
(From Line 4)
d)
How many outputs does it have?
________________
(From Line 5)
e)
How many NANDs does it have?
________________
(From Lines 14, 15, 16, 17)
f)
How many INVERTERS does it have?
________________
(From Lines 9, 10, 11)
g)
What are the commas in line 3 for?
________________
(From Line 3)
h)
What is the semicolon in line 3 for?
________________
(From Line 3)
i)
Why is there no semicolon in line 9?
________________
(From Line 9)
j)
Why is there no semicolon in line 19?
________________
(From Line 19)
k)
How many inputs do the
nand
s have?
________________
(From Lines 14, 15, 16, 17)
On the following diagram, you will combine what you have determined above to draw the circuit diagram
described by the Verilog code. Complete the diagram and give name and function of this circuit below. Build
or simulate the circuit if you need help.
Name: __________________
Function: ___________________
// Lab9Example1: Structural Verilog Description
// 1
// 2
module
Lab9Example1(E_n, A0, A1, D0_n, D1_n, D2_n, D3_n);
// 3
input
E_n, A0, A1;
// 4
output
D0_n, D1_n, D2_n, D3_n;
// 5
// 6
wire
A0_n, A1_n, E;
// 7
not
// 8
go(A0_n, A0),
// 9
g1(A1_n, A1),
//10
g2(E, E_n);
//11
//12
nand
//13
g3(D0_n, A0_n, A1_n, E),
//14
g4(D1_n, A0, A1_n, E),
//15
g5(D2_n, A0_n, A1, E),
//16
g6(D3_n, A0,A1, E);
//17
//18
endmodule
//19
The
not
and
nand
gate types are said to be “instantiated”. There are three instances of the
not
gate and four
instances of the
nand
gate. These are the usual Boolean gates that you are familiar with. Since all other
digital devices are composed of these, they are termed “primitives”.
The gates are defines with a name or “instance”, followed by a bracket showing the output first, followed by
inputs, separated by commas. (output terminal, input terminal 1, input terminal 2 …)
Note that there are only three inputs, E_n, A0 and A1. The “_n” indicates inversion so input E_n became
output E of the g2 instance of the inverter shown in line 11.
Input and output pins must be a physical conductor in the real world so they are classes as
wire
type. That
isn’t true of the output of the inverter g0. The input to g0 in line 9 is A0, a wire, but it’s output is A0_n. This
output is connected to the input of
nand
g3 in line 14. Since this connection must be a conductor, the
keyword
wire
list internal signals A0_n, A1_n and E as type
wire
in line 7.
Combine what we have determined about the digital circuit described by the Verilog code by drawing the
circuit. Draw the
inputs
,
outputs
,
wire
s,
nand
s and
not
s below and connect them as described in the code.
D0_n
D1_n
D2_n
D3_n
E_n
A0
A1
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
1.2
Lab9Example2
Examine the code following and analyse it as you did the previous example.
// Lab9Example2: Structural Verilog Description
// 1
// 2
module
Lab9Example2(S, D, Y);
// 3
input
[1:0] S;
// 4
input
[3:0] D;
// 5
output
Y;
// 6
// 7
wire
[1:0] not_S;
// 8
wire
[0:3] N;
// 9
//10
not
//11
gn0(not_S[0], S[0]),
//12
gn1(not_S[1], S[1]);
//13
//14
and
//15
g0(N[0], not_S[1], not_S[0], D[0]),
//16
g1(N[1], not_S[1], S[0], D[1]),
//17
g2(N[2], S[1], not_S[0], D[2]),
//18
g3(N[3], S[1], S[0], D[3]);
//19
//20
or
go(Y, N[0], N[1], N[2], N[3]);
//21
//22
endmodule
//23
In this example, a bus or multiple bit wire called a vector is used. In line 4, there is an input S composed of
two bits, S0 and S1. These are inverted in line 8 to become not_S0 and not_S1.
Applying the procedure we used in the previous example, we have six inputs, S0, S1, D0, D1, D2, D3, and
one output, Y.
There are 7 primitives instantiated:
- Two inverters, gn0 and gn1
- Four 3-input
and
gates, g0, g1, g2 and g3
- One 4-input or gate.
Lines 8 and 9 describe six internal wires:
- not_S0 and not_S1
-
N0, N1, N2 and N3
Build or simulate the circuit if you need help determining the name and function of the circuit described.
Name: __________________
Function: ___________________
S0
S1
D0
D1
D2
D3
Y
1.3
Lab9Example3
The following code describes the same circuit as one described in example 1 or 2. Examine the code
following and analyse it as you did the previous example to determine which circuit is implemented.
// Lab9Example3: Dataflow Verilog Description
//1
//2
module
Lab9Example3(S, D, Y);
//3
//4
input
[1:0] S;
//5
input
[3:0] D;
//6
//7
output
Y;
//8
//9
assign
Y = (~ S[1] & ~ S[0] & D[0])| (~ S[1] & S[0] & D[1])
//10
| (S[1] & ~ S[0] & D[2]) | (S[1] & S[0] & D[3]);
//11
//12
endmodule
//13
Applying the procedure we used in the previous example, we have six inputs, S0, S1, D0, D1, D2, D3, and
one output, Y, so we know this is the same circuit as in Lab9Example2. This time however, the description is
not structural. There are no
not
or
and
gates explicitly declared as the last time.
In this case, there is a Boolean equation
assign
ed to the
output
Y in lines 10 and 11 using the tilde for
complementing so that:
Y = ( /S1 • /S0 • D0) + ( /S1 • /S0 • D1) + ( S1 • /S0 • D2) + (S1 • S0 • D3)
When any of the variables on the right side changes, the equation is evaluated and Y is updated. If there are
more than one equation, they are evaluated in parallel – together. Note that these are bit-wise operators.
Build or simulate the circuit to verify that the name and function of the circuit is the same as Lab9Example2.
Name: __________________
Function: ___________________
1.5
Lab9Example4
The following code describes the same circuit as one described in Lab9Example1. Examine the code
following and analyse it as you did to see that this time it is a dataflow implementation.
// Lab9Example4: Dataflow Verilog Description
// 1
// 2
module
Lab9Exampl4(E_n, A0, A1, D0_n, D1_n, D2_n, D3_n);
// 3
input
E_n, A0, A1;
// 4
// 5
output
D0_n, D1_n, D2_n, D3_n;
// 6
// 7
assign
D0_n = ~(~E_n & ~A1 & ~A0);
// 8
assign
D1_n = ~(~E_n & ~A1 & A0);
// 9
assign
D2_n = ~(~E_n & A1 & ~A0);
//10
assign
D3_n = ~(~E_n & A1 & A0);
//11
//12
endmodule
//13
Like Lab9Example1 there are three inputs E_n, A0, A1 and four outputs, D0_n, D1_n, D2_n, D3_n. This
time however, the outputs are expressed in terms of Boolean equations. The keyword
assign
is an
assignment statement which assigns the output variable the value generated by the Boolean expression it is
equated to.
Build or simulate the circuit to verify that the name and function of the circuit is the same as Lab9Example1.
Name: __________________
Function: ___________________
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Related Questions
A) List and describe 10 features of the Atmel ATmega 2560 AVR microcontroller (use the
ATmega 2560 complete reference manual for help).
B) List and describe two AVR instructions. As part of your description make sure that you list
the operation for each instruction that is provided in the instruction set summary.
arrow_forward
The numbers from 0-9 and a no characters
is the Basic 1 digit seven segment display
* .can show
False
True
In a (CA) method of 7 segments, the
anodes of all the LED segments are
* "connected to the logic "O
False
True
Some times may run out of pins on your
Arduino board and need to not extend it
* .with shift registers
True
False
arrow_forward
Q2/A) Design 8x1 multiplexer using 2x1 multiplexer?
Q2 B)Simplify the Logic circuit shown below using K-map then draw the
Simplified circuit?
Q2/C) design logic block diagram for adding 12 to 5 using full adder showing
the input for each adder?
arrow_forward
Identify the part of UPS designed with low power rating than the power demand?
O a. Static Switch
O b. Inverter
O c. Battery
O d. Rectifier
arrow_forward
i need the answer quickly
arrow_forward
Please may you give the solution to this computer science question!
Thank you
arrow_forward
(a) Consider the flipflop circuits below:
(i) Name the 2 circuits given in the figure. Explain how they are different from each
other.
a.
b.
(ii) Choose from the list the input that triggers data to travel to the next flipflop in a
counter.
A: Input at top NAND gate
B: Input at bottom NAND gate
C: Clock input clk
Q
arrow_forward
Which of the following are part of the Arduino microcomputer.
The answer could be more than one
arrow_forward
Can i have a written solution solution for better understanding?
arrow_forward
Y=f(A,B,C)=(0,4,5)+don't care(2)
arrow_forward
In IC 74153,the input at pin number 14 is
Options
A-Connected to ground
B-Connected to power/VCC
C-Connected to LOGIC State
D-Connected to LOGIC Probe
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
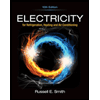
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning
Related Questions
- A) List and describe 10 features of the Atmel ATmega 2560 AVR microcontroller (use the ATmega 2560 complete reference manual for help). B) List and describe two AVR instructions. As part of your description make sure that you list the operation for each instruction that is provided in the instruction set summary.arrow_forwardThe numbers from 0-9 and a no characters is the Basic 1 digit seven segment display * .can show False True In a (CA) method of 7 segments, the anodes of all the LED segments are * "connected to the logic "O False True Some times may run out of pins on your Arduino board and need to not extend it * .with shift registers True Falsearrow_forwardQ2/A) Design 8x1 multiplexer using 2x1 multiplexer? Q2 B)Simplify the Logic circuit shown below using K-map then draw the Simplified circuit? Q2/C) design logic block diagram for adding 12 to 5 using full adder showing the input for each adder?arrow_forward
- (a) Consider the flipflop circuits below: (i) Name the 2 circuits given in the figure. Explain how they are different from each other. a. b. (ii) Choose from the list the input that triggers data to travel to the next flipflop in a counter. A: Input at top NAND gate B: Input at bottom NAND gate C: Clock input clk Qarrow_forwardWhich of the following are part of the Arduino microcomputer. The answer could be more than onearrow_forwardCan i have a written solution solution for better understanding?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Electricity for Refrigeration, Heating, and Air C...Mechanical EngineeringISBN:9781337399128Author:Russell E. SmithPublisher:Cengage Learning
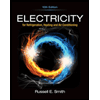
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning