M6L5 Generalization of LCGs
pdf
keyboard_arrow_up
School
California Lutheran University *
*We aren’t endorsed by this school
Course
ISYE6644
Subject
Electrical Engineering
Date
Oct 30, 2023
Type
Pages
6
Uploaded by CountTapir3340
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
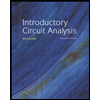
Introductory Circuit Analysis (13th Edition)
Electrical Engineering
ISBN:9780133923605
Author:Robert L. Boylestad
Publisher:PEARSON
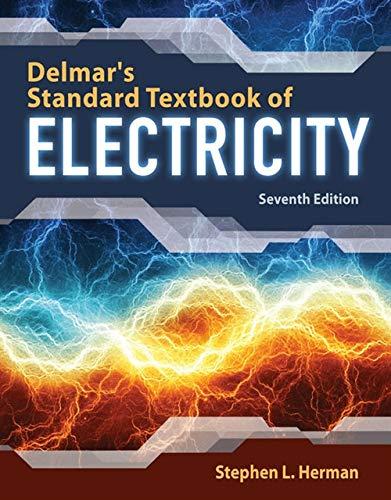
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
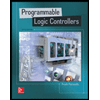
Programmable Logic Controllers
Electrical Engineering
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
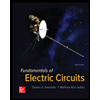
Fundamentals of Electric Circuits
Electrical Engineering
ISBN:9780078028229
Author:Charles K Alexander, Matthew Sadiku
Publisher:McGraw-Hill Education
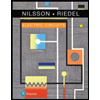
Electric Circuits. (11th Edition)
Electrical Engineering
ISBN:9780134746968
Author:James W. Nilsson, Susan Riedel
Publisher:PEARSON
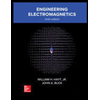
Engineering Electromagnetics
Electrical Engineering
ISBN:9780078028151
Author:Hayt, William H. (william Hart), Jr, BUCK, John A.
Publisher:Mcgraw-hill Education,
Recommended textbooks for you
- Introductory Circuit Analysis (13th Edition)Electrical EngineeringISBN:9780133923605Author:Robert L. BoylestadPublisher:PEARSONDelmar's Standard Textbook Of ElectricityElectrical EngineeringISBN:9781337900348Author:Stephen L. HermanPublisher:Cengage LearningProgrammable Logic ControllersElectrical EngineeringISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
- Fundamentals of Electric CircuitsElectrical EngineeringISBN:9780078028229Author:Charles K Alexander, Matthew SadikuPublisher:McGraw-Hill EducationElectric Circuits. (11th Edition)Electrical EngineeringISBN:9780134746968Author:James W. Nilsson, Susan RiedelPublisher:PEARSONEngineering ElectromagneticsElectrical EngineeringISBN:9780078028151Author:Hayt, William H. (william Hart), Jr, BUCK, John A.Publisher:Mcgraw-hill Education,
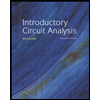
Introductory Circuit Analysis (13th Edition)
Electrical Engineering
ISBN:9780133923605
Author:Robert L. Boylestad
Publisher:PEARSON
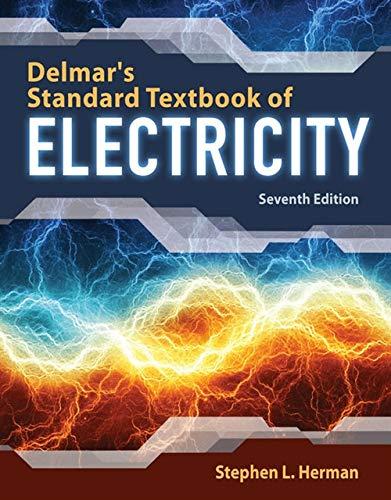
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
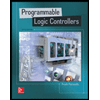
Programmable Logic Controllers
Electrical Engineering
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
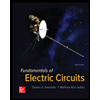
Fundamentals of Electric Circuits
Electrical Engineering
ISBN:9780078028229
Author:Charles K Alexander, Matthew Sadiku
Publisher:McGraw-Hill Education
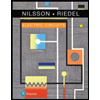
Electric Circuits. (11th Edition)
Electrical Engineering
ISBN:9780134746968
Author:James W. Nilsson, Susan Riedel
Publisher:PEARSON
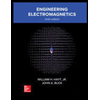
Engineering Electromagnetics
Electrical Engineering
ISBN:9780078028151
Author:Hayt, William H. (william Hart), Jr, BUCK, John A.
Publisher:Mcgraw-hill Education,
Browse Popular Homework Q&A
Q: Among the descendants of the ancestor common to lineages A and B, how many total extinction events…
Q: When a variety of processes are active at once, how does the capacity analysis react?
Q: . A physically small (treat as a point mass) box weighing 80 lb is
sitting on a floor. The…
Q: 2. You are trying to determine the average anxiety level of students in a university class over
time…
Q: A particle moves according to a law of motion s = f(t), t ≥ 0, where t is measured in seconds and s…
Q: Researchers conducted a study to determine whether magnets are effective in treating back pain. The…
Q: An electromagnetic plane wave travels from a denser medium with a refractive index of 2.8
to a rarer…
Q: A swimming pool has dimensions 36.0 m ✕ 7.0 m and a flat bottom. The pool is filled to a depth of…
Q: Describe what immunity is and how the different types of immunity are acquired.
Q: Do you know examples for experiments where light shows
wave-like or particle-like character?…
Q: You are conducting an exercise test, and the ability to read the ECG progressively gets worse. By…
Q: 3. Analyze the following force diagram. That is, write down EF, and EFy. Don't do the ma part.
A
B
9…
Q: Assume that a simple random sample has been selected from a normally distributed population and test…
Q: 5
Sº
dx
400 + x²
Q: 2-D Motion Horizontally Launched Projectiles Part 1
Here are the equations you may need...
You will…
Q: You will need to use these equations as you solve the problems.
horizontal
X=
dx=vxt
Vx
dx
t
Y =…
Q: P = 0.1176
(a) Do you reject or fail to reject Ho at the 0.01 level of significance?
O A. Fail to…
Q: A 2.20-kg particle has a velocity (2.10 î − 5.00 ĵ) m/s, and a 3.50-kg particle has a velocity (1.00…
Q: failure of HR to decrease by _________ during the 1st minute or by ______ by the end of the 2nd…
Q: Is the number of points Jimmy Butler scored per game within one standard deviation from the average…
Q: A 90% confidence interval for a population mean is (65, 77). The population distribution is…
Q: Who killed Reconstruction ?
Q: 16. A 170 pound man wants to design a workout (on a treadmill) that will
expend 300 kcal. He wants…
Q: Why is it important for persons who work in other areas in a business to have an understanding of…
Q: What are the arguments for and against Economic Growth? Write in four paragraphs