CIS1500_Assignment3_Description_updated
pdf
keyboard_arrow_up
School
University of Guelph *
*We aren’t endorsed by this school
Course
1300
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
12
Uploaded by MajorThunderIbex35
1) CIS1500 Assignment 3: Student Finance Calculator
a)
Total Marks:
10 marks
b)
Weight:
10%
c)
Due:
Friday, 1st Dec 2023, at 11:59 pm
Welcome to your last CIS1500 assignment! Please ensure you read all the sections and
follow the
instructions exactly
as we will grade your submission with an auto-grader. You must
test your
assignment on the SoCS Linux server
, and it will be similarly graded on the SoCS Linux server for
consistency.
Your program should only use concepts already taught in this course.
You will receive a 0 if your code
includes: global variables
(variables declared outside of functions),
goto, malloc, calloc, regex, or if
your code fails to compile without errors.
Note there are already two global
constants
provided for you
in the .h file.
This pdf goes in conjunction with videos available on courselink.
There is also a file containing “Skeleton Code”; you should use this as your starting point. It contains 3
files:
2)
A .c file for your main called
skeleton.c. You must rename this before submitting.
3)
A .c file for your functions called
A3_Functions.c. Do
not
rename this before submitting.
4)
A .h file for function prototype called
A3_Functions.h. This file is already completed, do not
modify it.
You do not need to submit it.
This document has 15 pages. Read them all and understand the requirements.
REMEMBER: You are to submit
one zip file containing two source code files
for your program.
●
The name of the
zip file
follows the following format:
studentNumberA3.zip
o
Example:
John
Snow’s
student
number
is
1770770
then
the
zip
file
name
is
1770770A3.zip
●
The two files within the zip you are to submit are non-compiled code C files (ends with .c)
containing your code
o
Do not include any other files or folders in your submission; they will be ignored.
o
Do not include this file inside another folder; it will be ignored by the auto-grader.
●
The name of the
C file
containing your main follows the following format:
studentNumberA3.c
o
Example: John Snow’s student number is 1770770 then the C file name is 1770770A3.c
●
The name of the C file containing your functions is A3_Functions.c
●
Incorrect submission will result in a grade of 0.
●
More details are outlined in Section 4: Program Submission and Administration Information.
Start Early. Get Help Early. Test Often.
1. Background
Please refer to the A1 and A2 outline for background, as this assignment is heavily based on A2. You
need to thoroughly understand both assignment outlines. In this assignment we will make 3 major
changes:
●
A menu is displayed asking the user what the wish to do.
●
Finance data is located in files, they can be loaded from files to view, or new files can be
created with the program.
●
We can change the “budget rules”, whereas previously we had hardcoded a 50/30/20 rule,
it can now be set and changed to bette
●
When finance data is loaded, it is kept in a struct that is provided. We are able to store
finance information for multiple people/years so an array of structs is required.
The output of your program should look very similar to that of A2 with three exceptions:
●
We use a menu system for the user to select options.
●
We request a name and year for finance data in addition to the actual finance information.
This program is intended to test your ability to:
●
Use structs, pass them to functions, return from functions, and modify the members.
●
Read and write data from/to text files.
This is an example of the contents of the data file. We have provided multiple sample files for you to test
your code with. When testing we will only use valid input files, with the exact format as those provided.
The course and assignment are not intended as actual finance advice.
2. Program Requirements
Your program will start with a welcome message, then prompt the user to select an option from a menu.
The menu requires the user to enter an integer value associated with the member option.
i)
2.1 Required Functions and Menu
This assignment has 5 options, each requiring 1 or two mandatory functions:
1)
Read finances from file.
This option will allow the user to enter a filename, which will load the data from that associated file. The
filename is passed into the function
FinanceData readFinanceDataFromFile(char filename[]);
which
will create a FinanceData struct, set the members of the struct to the appropriate values, and then return
the struct. Most of the data for the members are stored in the file, but some values (ex. monthly_funds)
may need to be calculated within the function. If a file cannot be loaded the message “Error: File not
found\n” is displayed.
Below is an example of the program output
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2)
Enter finances and save to file
If the user selects option 2, they are prompted to enter a filename. Then the function
FinanceData
readFinanceDataFromUser();
is called which asks the name and year for the finances. Then the
questions from A2 are asked to obtain finance information. This information is all loaded into a newly
created struct, and that struct is returned. Remember that some of the struct members will require you to
perform calculations based on the input.
After the struct is created and added to an array of structs for future use, the struct is passed to the
function
void saveFinanceRecordToFile(FinanceData fd, char filename[MAX_STRING_LEN]);
which saves the data the filename recorded previously.
3)
Enter budget rules (ex. 50/30/20).
While previously we always followed
50/30/20 rules - for expenses, wants, and
savings - in A3 the user may choose to
select new ratios. So a user may instead opt
to have ratios 70/30/0 for 70% of funds
going to expenses, 30% for wants, and 0%
for savings.
To do this you will need variables that
store these rations, and use the function
void getBudgetRules(int
*essentials_ratio, int *wants_ratio, int
*savings_ratio);
which takes the ratios as
a reference and modifies them based on the
user input.
*** Hints:
- I recommend starting with option 2. It is
separated into two parts, you can copy a lot
of your A2 code for reading input and
create the struct. After you have tested that
and other features, you can work on saving
the struct to a file later.
- Next I would work on option 4, this
option mostly uses existing code that we
provided, and gives you a chance to verify
the output.
- Thirdly I would work on option 3. Now
that
you
have
the
ability
to
print
the
finance data you can verify that it works.
-
Remember
that
you
can
add
print
statements for testing wherever you need.
This is often the easiest and most effective
way to test that your code is doing what
you think, and helps tremendously with
debugging.
4)
Print most recent finances.
This option will print the table and summary for the most recent finance data entered (either by file or
entered by user). Remember that you store the finance structs in an array, so this will print the file in the
last position of the array.
To print you will create a new function
void printFinances(FinanceData fd, int essentials_ratio, int
wants_ratio, int savings_ratio);
which calculates monthly_savings, monthly_wants etc. before calling
the printTable function (provided) and the printSummary function (provided).
See image from option 3 above for an example.
5)
Select finances to print.
This option behaves very similar to option
4, however first the user is prompted to
select which finance data to print.
To select we use a new function:
int
selectFinanceData(FinanceData fds[], int
numEntries);
. A numbered list with the
names and years of the available data are
displayed. The user will enter the value
associated with the finance data. The index
of the finances data they selected is
returned (ie their input -1).
Sample to the right ->
6)
Exit.
The final option is to exit the program, until
the user selects option 6, the program will
loop repeatedly allowing them to select any
of the other options.
*** Note:
-
The
printSummary
and
printTable
functions
provided
have
been
slightly
modified as we have variable budget rules.
- When testing we will only use valid input
files,
with
the
exact
format
as
those
provided.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
As mentioned previously, the finance data is stored in a struct. This struct is already provided in
A3_Functions.h:
typedef struct
{
// Data from user/file
char
username
[
MAX_STRING_LEN
];
int
year
;
float
savings
;
float
grants
;
float
weekly_pay
;
float
annual_allowance
;
float
rent
;
float
utilities
;
int
semesters
;
float
tuition
;
float
textbooks
;
int
num_expenses
;
char
expense_names
[
MAX_NUM_EXPENSES
][
MAX_STRING_LEN
];
float
expense_costs
[
MAX_NUM_EXPENSES
];
// data calculated from above
float
net_funds
;
float
monthly_funds
;
float
monthly_income
;
float
monthly_budget
;
float
essentials_total
;
int
isValid
;
// 1 if data is valid, 0 otherwise
}
FinanceData
;
The final member isValid, can be used to help with determining whether a struct should be added to your
array of structs. For example if a filename does not exist, you might set the value to 0 as a flag that you
should not add to the array.
3. Assessment
The assignment is graded out of 10 marks. The last page of this document shows an example of what a
rubric/feedback file might look like. The 10 marks are split between these three categories:
ii)
3
.1 The First
2
Marks
This section tests the overall flow of your program; your program will receive input from a user, do
calculations, and output the table and summary.
We will run multiple tests using different input values
then compare your output against the expected output.
iii)
3.2 The Next 6 Marks
Function Testing: we will run multiple tests here to
evaluate the required functions.
To do this we will
create a separate .c file with a new main, we will include your A3_Functions.c file, and call your
functions with our main.
This will allow us to evaluate each function one at a time, so even if your program does not pass the flow
tests, you can still receive marks for working functions.
It is imperative that you do not change the function headers
from how they are shown in the
prototypes in A3_Functions.h. The best option would be to directly copy from the .h file in the skeleton
code, and paste into your A3_Functions.c. If you change the function headers in your .c file then errors
will occur when we attempt to unit test the functions.
iv)
3.3
The Next
1
Marks
Your output text formatting will be graded out of 1.
We will flag differences in spellings, spaces,
tables, new lines, etc. and test against different input values using the auto-grader, so make sure you
follow the output exactly. For each unique error, 0.5 marks will be deducted. The formatting grade will
not be negative, it will only range from 0 to 1.
Refer to the sample flows provided for how your program is expected to run (4 Sample Flows).
Altering/customizing sentences will not be accepted, and you will lose grades in this category if you do
so.
v)
3
.
4
The Final
1
Mark
Style: Indentation, variable names, and comments.
Comments are most commonly used to explain confusing or not easily understood parts of code or for
depicting that the following code carries out a certain piece of logic. These are also used to explain the
program and add a header to it. A file header comment is a type of comment that appears at the top of
your program before the #include line(s).
Comment grades include header comments (see 5.3 File
Header Comment Format) and meaningful comments throughout your code.
Each code block should be
indented for readability
(if/else statements are an example of a code block).
We are looking for
meaningful variable names
that depict the values they are representing – this will
make it easier for you to remember what each variable is for and for us to grade you!
4. Sample Flows
Pay close attention to the sample flow images above, and watch the video sample flow posted to
courselink for additional information. The skeleton code contains most of the table formatting already, so
you can use that as a basis.
Note that
your submission will be either fully or partially graded by an auto-grader
. To get full
grades you need to match our output requirements exactly.
5. Program Submission and Administration Information
The following section outlines what is expected when submitting the assignment and various other
administration information with respect to the assignment. To submit your assignment, upload a single zip
file to the Dropbox box for A3 on Courselink.
vi)
5
.1 The Submission File
The following is expected for the file that is to be submitted upon completing the assignment:
●
You are to submit
one zip file containing two source code files
for your program.
●
The name of the
zip file
follows the following format:
studentNumberA3.zip
o
Example:
John
Snow’s
student
number
is
1770770
then
the
zip
file
name
is
1770770A3.zip
●
The two files within the zip you are to submit are non-compiled code C file (ends with .c)
containing your code
o
Do not include any other files or folders in your submission; they will be ignored.
o
Do not include this file inside another folder; it will be ignored by the auto-grader.
●
The name of the
C file containing main()
follows the following format:
studentNumberA3.c
o
Example: John Snow’s student number is 1770770 then the C file name is 1770770A3.c
●
The name of the C file containing your functions is A3_Functions.c
o
Do not include the .h file
o
Do not change the function headers (return types, names, or arguments) from those in the
.h file.
●
Incorrect zip file or C file name will result in a grade of 0.
●
To ensure you zip correctly and in the right format, we require you to zip your submission
through the command line using the following command:
zip studentNumberA3.zip studentNumberA3.c A3_Functions.c
Make sure to replace studentNumber with your own student number.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
vii)
5
.2 Program Expectations
Your program is expected to follow the outlined information exactly. Failure to do so will result in
deductions to your assignment grade.
●
Your program should be compiled and tested on the SoCS Linux server.
●
You must use the following command to compile your code:
gcc -Wall -std=c99 studentNumberA3.c A3_Functions.c -lm
o
Example: For John Snow’s submission, the command would be:
gcc -Wall -std=c99 1770770A1.c A3_Functions.c -lm
●
Programs that produce warnings upon compilation will receive a deduction of 1 mark for each
type/category of warning
●
The program file must contain instructions for the TA on how to compile and run your program in
a file header comment (see 3.3 File Header Comment Format).
You will receive a 0 if:
●
your program does not compile/creates errors when compiled with gcc -std=c99 -Wall on the
SoCS Linux server.
●
your program contains any global variables (variables declared outside of a function)
●
you program uses:goto statements
●
you program uses malloc
●
you program uses calloc
●
you program uses regex
●
you program uses concepts not already taught in this course (ie. structs, dynamic arrays, etc.).
viii)
5
.3 File Header Comment Format
Note: This sample uses the name John Snow; the
file name, student name, student ID, file descriptions,
etc. must be changed
.
/************************** 1770770A3.c **************************
Student Name: John Snow
Student ID: 1770770
Due Date: Friday, 1st Dec 2023, at 11:59 pm
Course Name: CIS*1500
I have exclusive control over this submission via my password.
By including this statement in this header comment, I certify that:
1) I have read and understood the University policy on academic integrity; and
2) I have completed assigned video on academic integrity.
I assert that this work is my own. I have appropriately acknowledged any and all material (code, data,
images, ideas or words) that I have used, whether directly quoted or paraphrase. Furthermore, I certify
that this assignment was prepared by me specifically for this course.
***************************************************************
This file contains…
Describe the program, functions, important variables, etc.
***************************************************************
The program should be compiled using the following flags:
-std=c99
-Wall
-lm
Compiling:
gcc -Wall -std=c99 1770707A3.c A3_Functions.c -o A3 -lm
OR
Gcc -Wall -std=c99 1770707A3.c A3_Functions.c -lm
Running the program:
./A3
OR
./a.out
***************************************************************/
5.3 Grading Scheme:
This is an example of what feedback might look like. The 12 Function Test Cases will test the functions
individually. We will use our own main function, and include your A3_Functions.c file, then test each
function individually. For example, our program might have the code:
float unitTest1 = getFloatInput(“Unit test1: “);
printf(“%f\n”, unitTest1);
And then enter -10 and 42. We expect that “Unit test1: ” is printed, then the phrase “Invalid Input” is
printed, followed by the “42”. This way we can test each function not just the complete program. The 2
flow tests will be similar to those in a1, looking at the overall flow of the program given a complete set of
finance inputs.
a3 Marking Scheme: 1770770
Style (out of 1.0)
* Indentation (0.5/0.5)
* Commenting/Readability (0.5/0.5)
Test Cases and Outputs (out of 8.0)
* Function Test Case 1 (0.5/0.5)
* Function Test Case 2 (0.5/0.5)
* Function Test Case 3 (0.5/0.5)
* Function Test Case 4 (0.5/0.5)
* Function Test Case 5 (0.5/0.5)
* Function Test Case 6 (0.5/0.5)
* Function Test Case 8 (0.5/0.5)
* Function Test Case 9 (0.5/0.5)
* Function Test Case 10 (0.5/0.5)
* Flow Test 1 (1.0/1.0)
* Flow Test 2 (1.0/1.0)
* Flow Test 3 (0.5/0.5)
* Flow Test 3 (0.5/0.5)
Grade for Output Formatting (1.0/1.0)
Final grade: 8.5 /10.0
TA Comments:
Should say "Welcome to the CIS1500 Student Finance Planner. We have a few questions for you:" not
"Hello and Welcome". Program did not exit after invalid input from use.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
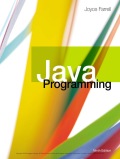
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
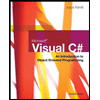
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
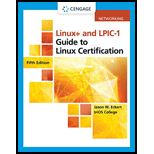
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
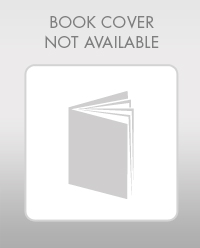
A+ Guide To It Technical Support
Computer Science
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:Cengage,
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE LNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageA+ Guide To It Technical SupportComputer ScienceISBN:9780357108291Author:ANDREWS, Jean.Publisher:Cengage,
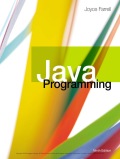
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
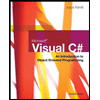
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
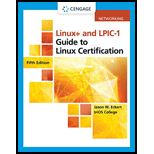
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
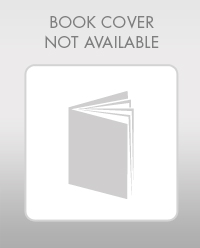
A+ Guide To It Technical Support
Computer Science
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:Cengage,