Design and Implementation of a Banking System (1) ANISH assignment
docx
keyboard_arrow_up
School
Federation University *
*We aren’t endorsed by this school
Course
2002
Subject
Computer Science
Date
Oct 30, 2023
Type
docx
Pages
16
Uploaded by MasterSummer4547
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
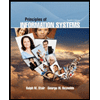
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
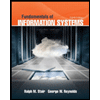
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
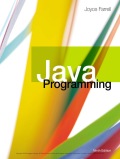
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
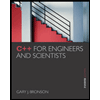
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
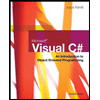
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Recommended textbooks for you
- Principles of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningFundamentals of Information SystemsComputer ScienceISBN:9781305082168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
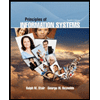
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
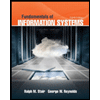
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
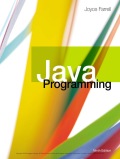
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
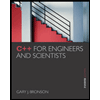
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
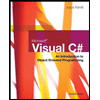
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Browse Popular Homework Q&A
Q: In 50 words, tell the reason why PPF do not bow inward.
Q: A car manufacturer is designing a new, lighter sports car. It has a mass of 1,000 kg. They ran 5…
Q: management of Advanced Alternative Power Inc. is considering two capital investment projects. The…
Q: What are the premises and conclusion of the following argument?
"If x = 9, then x + 4 = 13."…
Q: 150
125
100
75+
50
1
25
Q: Identify the relational keys for each table. List each relational key in a separate line.
Candidate…
Q: 2. For F(x, y, z) = (6xsin y+10x=' )i + (3x² cos y-4ye* −2y²z³)j + (-2y³e² +15x²2²)k, find
a…
Q: Organotin compounds play a significant role in diverse industrial applications. They have been used…
Q: 2. Use the method of undetermined coefficients in section 3.5 to find the form of a particular…
Q: Find the vertex, focus, and directrix for the parabola. (y + 3)2 = 8(x − 2)
Vertex:…
Q: A semicircular gate with a diameter of 6 ft is installed at 50º as shown below. The top of the gate…
Q: 2-Sided CI, a 95, Sample Average 100, Population St Dev, s 5, 15…
Q: Describe the similarities between risk transfer and risk sharing.
Q: To induce a current in a wire coil, a magnet must be
spinning
at rest
moving
O strong
Q: 2
3
Q: C. Logarithmic on one side
6. log, x+log,2(x-3)=2
D. Logarithmic on both sides.
7. In(3x+8) =…
Q: A foreign key is the candidate key that is selected to identify tuples.
True or False.
Q: What is the approximate concentration of free Al³+ ion at equilibrium when 1.86x10-²
mol aluminum…
Q: a. $600 interest from a savings account at a Florida bank.
b. $5,000 dividend from U.S. Flower…
Q: 7. Solve the system of equations. You may use any method (elimination, substitution, matrices,…
Q: Solve the system by back substitution.
-4x-8y-2z - 6w = -5
-5y-30z-10w = -40
- 5z-5w = - 10
- 5w=…
Q: 5 m
5 m
A
D
G
H
0.6 m
B
0.2 m
E
0.4 m
C
F
12 kN
6 kN
Q: Give an
Q: and
Q: reater than 30, the Poisson distribution begins to be very similar to the normal with both a mean…