Assignment 03
docx
keyboard_arrow_up
School
Centennial College *
*We aren’t endorsed by this school
Course
123
Subject
Computer Science
Date
Feb 20, 2024
Type
docx
Pages
21
Uploaded by DukeKnowledge13209
Programming II
Medal: enums, properties, filtering a list
Page 1 of 21
Part A
This exercise you will be exploring more usage of the static keyword.
You must following the specifications exactly
The Account class
This is the front end of the application. There are 12 members.
Account
Class
Constants
-
TRANSIT_NUMBER : int
= 314
Fields
$-
NEXT_ACCOUNT_NUMBER : int
+
«readonly» Number : string
Properties
+
«property private setter» Balance : double
+
«property private setter» Names : List
<
string
>
Methods
-
«constructor» Account(number :
string
, name :
string
,
balance :
double
)
$
«constructor» Account()
$+
CreateAccount(name :
string
, balance :
double
) : Account
+
ToString () : string
+
Deposit(amount : double
) : void
+
Withdraw(amount : double
) : void
+
AddName(name : string
) : void
Programming II
Medal: enums, properties, filtering a list
Page 2 of 21
Description of class members
Constants:
TRANSIT_NUMBER
– this private int is a constant
representing the branch number of all the accounts.
That is initialized to 314. It is used in the CreateAccount()
method to build the account
number of this account.
Fields:
NEXT_ACCOUNT_NUMBER
– this private class variable is an int representing the unique number to be used when creating a new account. This is initialized in the static constructor. This is incremented in the instance constructor
Number
– this public instance variable is a string
indicating the current account number of this object
reference. This must be decorated with the readonly
keyword. This is set in the Account(string,
string, double)
method.
Properties:
Balance
– this property is a double that represents the current balance of this object. . This getter is public and the setter is private.
Names
– this property is a list of string representing the name associated with this object. This getter is public and the setter is private.
Constructor:
There are two constructors for this class: a static that is called before any member is accessed and a private one to prevent object instantiation outside of class.
static Account()
– This is the static constructor.
It will be used to initialize the class variable CURRENT_ACCOUNT_NUMBER
to a suitable value
e.g. 100_000
private Account(
string number, string
name, double balance)
– This is a private
constructor that does the following:
The Number field and the Balance property are initialized to the values of the arguments
The Names property is initialised to an empty list (use the new operator). And the argument is added to this collection
A const
is a value that cannot be modified. Its value is set at declaration. i.e. When the program is compiled.
A readonly
field is like a consts
but it is normally assigned when the program is running.
A static constructor is used to initialise the static fields and properties. It is invoked once in the life time of a program, before ANY member is accessed. There is no access modifier i.e.
public, protected or private
Programming II
Medal: enums, properties, filtering a list
Page 3 of 21
Methods
public static Account CreateAccount(
string name, double balance = 500)
– This is a public static method is used to create accounts. It does the following:
Builds an account number from the TRANSIT_NUMBER
and CURRENT_ACCOUNT_NUMBER
according to the template “AC-[transit number]-[current account number]. e.g. if the value of TRANSIT_NUMBER
and CURRENT_ACCOUNT_NUMBER
are 314 and 10005 respectively then the account number will be "AC-314-10005". [Hint: use string.Format()
method to do this.]
It also increments the CURRENT_ACCOUNT_NUMBER
field so the next object will have a unique number.
Instantiate a new account object with the appropriate arguments.
Returns the above object
public void AddName(
string name)
– This is a public method adds the argument to the Names collection. It is possible to have multiple names associated with this account. This method does not return a value.
public void Deposit(
double amount)
– This is a public method that increases the property Balance by the amount specified by the argument. This method does not return a value.
public void Withdraw(
double amount)
– This is a public method that decreases the property Balance by the amount specified by the argument. This method does not return a value.
public override string ToString()
– Add word “Liaison” to the output of the
method please
This is a public method overrides the corresponding
method in the object class to return a stringify form of
the object. To display the Names field, you will have to
do some extra work because it is a list of strings [] Test Harness
Insert the following code statements in your Program.cs file:
List
<
Account
> accounts = new
List
<
Account
>();
Random
rand = new
Random
();
accounts.Add(
Account
.CreateAccount(
"Narendra"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Ilia"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Yin"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Arben"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Patrick"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Joanne"
, rand.Next(50, 500)));
The static string method Join enables you to stringify a collection. Use the following code to get string from a collection:
string
.Join(
", "
, Names);
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Programming II
Medal: enums, properties, filtering a list
Page 4 of 21
accounts.Add(
Account
.CreateAccount(
"Nicoleta"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Mohammed"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Hao"
, rand.Next(50, 500)));
accounts.Add(
Account
.CreateAccount(
"Mayy"
, rand.Next(50, 500)));
Console
.WriteLine(
"\nAll accounts"
);
foreach
(
Account
account in
accounts)
{
Console
.WriteLine(account);
}
foreach
(
Account
account in
accounts)
{
account.Deposit(rand.Next(4, 8) * 10);
}
Console
.WriteLine(
"\nAfter deposit "
);
foreach
(
Account
account in
accounts)
{
Console
.WriteLine(account);
}
foreach
(
Account
account in
accounts)
{
account.Withdraw(rand.Next(2, 5) * 10);
if
(account.Balance > 300)
account.AddName(
"(Hacked)"
);
}
Console
.WriteLine(
"\nAfter withdrawal"
);
foreach
(
Account
account in
accounts)
{
Console
.WriteLine(account);
}
Test Harness
Output
Note the following balances will be different from the following output.
All accounts
[AC-314-10000] Narendra $390.00
[AC-314-10001] Ilia $228.00
[AC-314-10002] Yin $210.00
[AC-314-10003] Arben $351.00
[AC-314-10004] Patrick $224.00
[AC-314-10005] Joanne $210.00
[AC-314-10006] Nicoleta $126.00
Programming II
Medal: enums, properties, filtering a list
Page 5 of 21
[AC-314-10007] Mohammed $194.00
After deposit
[AC-314-10000] Narendra $445.55
[AC-314-10001] Ilia $283.55
[AC-314-10002] Yin $265.55
[AC-314-10003] Arben $406.55
[AC-314-10004] Patrick $279.55
[AC-314-10005] Joanne $265.55
[AC-314-10006] Nicoleta $181.55
[AC-314-10007] Mohammed $249.55
After withdrawal
[AC-314-10000] Narendra, (Hacked) $444.44
[AC-314-10001] Ilia $282.44
[AC-314-10002] Yin $264.44
[AC-314-10003] Arben, (Hacked) $405.44
[AC-314-10004] Patrick $278.44
[AC-314-10005] Joanne $264.44
[AC-314-10006] Nicoleta $180.44
[AC-314-10007] Mohammed $248.44
Press any key to continue . . .
Programming II
Medal: enums, properties, filtering a list
Page 6 of 21
Part B
You will practice using Properties and iterating a list.
The MedalColor Enum
Code the MedalColor enum below:
This enum consist of 3 constants which does not require any special treatment (such as setting any Flags attribute). MedalColor
enum
Constants
Bronze
Silver
Gold
The Medal Class
Code the Medal class below:
This class comprise of five properties, a constructor and a ToString()
method. All the properties are public readonly. Medal
Class
Properties
+
«property setter absent» Name : string
+
«property setter absent» TheEvent : string
+
«property setter absent» Color : MedalColor
+
«property setter absent» Year : int
+
«property setter absent» IsRecord : bool
Methods
+
«constructor» Medal(
name : string
,
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Programming II
Medal: enums, properties, filtering a list
Page 7 of 21
theEvent : string
, color : string
, year : int
, isRecord : bool
)
+
ToString() : string
Description of class members
Properties:
All the properties have public getter and the setter is absent making them all readonly properties
Name
– this is a string representing the holder of this object. The getter is public and the setter is absent.
TheEvent
– this is a string representing the event of this object. (Event is a reserved word in C#). The getter is public and the setter is absent.
Color
– this is an enum representing the color of this object The getter is public and the setter is absent.
Year
– this is an integer representing the year of this object. The getter is public and the setter is absent.
IsRecord
– this is a bool indicating if this was a record setting event. The getter is public and the setter
is absent.
Fields:
No fields are defined in this class
Constructor:
public
Medal(
string name, string theEvent, MedalColor color, int year,
bool isRecord)
– This public constructor takes five arguments: a string representing the name, a string representing the event, a string representing the type of medal, an integer representing the year and a bool indicating if a World Record or Olympic Record was set in this event. It assigns the arguments
to the appropriate fields.
Methods:
public override string
ToString()
– This public method overrides the ToString of the object class. It does not take any argument and returns a string representation of the object. You may return a string in the format “
2012 - Boxing(R) Narendra(Gold)
”.
Programming II
Medal: enums, properties, filtering a list
Page 8 of 21
If the event is not a record event then the “
(R)
” should not be present in the output. The ToString()
method is the best place to implement this feature.
Programming II
Account: private constructor, const, readonly, static Test Harness
Insert the following code statements in the Main()
method of your Program.cs file:
//create a medal object
Medal
m1 = new
Medal
(
"Horace Gwynne
"
, "Boxing"
, MedalColor.
Gold
, 2012, true
);
//print the object
Console
.WriteLine(m1);
//print only the name of the medal holder
Console
.WriteLine(m1.Name);
//create another object
Medal
m2 = new
Medal
(
"Michael Phelps"
, "Swimming"
, MedalColor.
Gold
, 2012, false
);
//print the updated m2
Console
.WriteLine(m2); //create a list to store the medal objects
List
<
Medal
> medals = new
List
<
Medal
>(){ m1, m2};
medals.Add(
new
Medal
(
"Ryan Cochrane
"
, "Swimming"
, MedalColor.
Silver
, 2012, false
));
medals.Add(
new
Medal
(
"Adam van Koeverden
"
, "Canoeing"
, MedalColor.
Silver
, 2012, false
));
medals.Add(
new
Medal
(
"Rosie MacLennan
"
, "Gymnastics"
, MedalColor.
Gold
, 2012, false
));
medals.Add(
new
Medal
(
"Christine Girard
"
, "Weightlifting"
, MedalColor.
Bronze
, 2012, false
));
medals.Add(
new
Medal
(
"Charles Hamelin
"
, "Short Track"
, MedalColor.
Gold
, 2014, true
));
medals.Add(
new
Medal
(
"Alexandre Bilodeau
"
, "Freestyle skiing"
, MedalColor.
Gold
, 2012, true
));
medals.Add(
new
Medal
(
"Jennifer Jones
"
, "Curling"
, MedalColor.
Gold
, 2014, false
));
medals.Add(
new
Medal
(
"Charle Cournoyer
"
, "Short Track"
, MedalColor.
Bronze
, 2014, false
));
medals.Add(
new
Medal
(
"Mark McMorris
"
, "Snowboarding"
, MedalColor.
Bronze
, 2014, false
));
medals.Add(
new
Medal
(
"Sidney Crosby
"
, "Ice Hockey"
, MedalColor.
Gold
,
2014, false)
);
medals.Add(
new
Medal
(
"Brad Jacobs
"
, "Curling"
, MedalColor.
Gold
, 2014,
false)
);
medals.Add(
new
Medal
(
"Ryan Fry
"
, "Curling"
, MedalColor.
Gold
, 2014, false)
);
Page 9
of 21
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Programming II
Account: private constructor, const, readonly, static medals.Add(
new
Medal
(
"Antoine Valois-Fortier
"
, "Judo"
, MedalColor.
Bronze
, 2012, false
));
medals.Add(
new
Medal
(
"Brent Hayden
"
, "Swimming"
, MedalColor.
Bronze
, 2012, false)
);
//prints a numbered list of 16 medals.
Console
.WriteLine(
"\n\nAll 16 medals"
); //prints a numbered list of 16 names (ONLY)
Console
.WriteLine(
"\n\nAll 16 names"
); //prints a numbered list of 9 gold medals
Console
.WriteLine(
"\n\nAll 9 gold medals"
); //prints a numbered list of 9 medals in 2012
Console
.WriteLine(
"\n\nAll 9 medals"
); //prints a numbered list of 4 gold medals in 2012
Console
.WriteLine(
"\n\nAll 4 gold medals"
); //prints a numbered list of 3 world record medals
Console
.WriteLine(
"\n\nAll 3 records"
); //saving all the medal to file Medals.txt
Console
.WriteLine(
"\n\nSaving to file"
); Page 10
of 21
Programming II
Account: private constructor, const, readonly, static Part C
You will be practicing to read from a file and building a relatively more complex system consisting o the three interconnected types that is illustrated below:
All the above type must decorated with the public keyword
The SongGenre enum
This enum comprise of seven types of songs
To code this add a new item to your project and select the class option. Make the necessary changes to the header to reflect the enum type. You should decorate this enum with the Flags
attribute, because values may be combined e.g. a song may belong to multiple genres such as Country+Blues.
In order to make the flags work as you would expect, you must set each value to an ascending power of 2 e.g. 0, 1, 2, 4, 8, 16 …
or simply use the binary values as shown in the diagram below.
SongGenre
Enum
Members
Unclassified = 0
Pop = 0b1
Rock = 0b10
Blues = 0b100
Country = 0b1_000
Metal = 0b10_000
Soul = 0b100_000
If you examine the binary values for each enum, you will notice that each value has exactly a single value
one, therefore as possible value can only come from a unique combination of the base value. e.g. 0b1_010
is a result of combining Rock
and Country
Page 11
of 21
Programming II
Account: private constructor, const, readonly, static The Song class
This acts like a record for the song. The setter is missing for all the properties
Song
Class
Fields
Properties
+
«property setter absent» Artist : string
+
«property setter absent» Title : string
+
«property setter absent» Length : double
+
«property setter absent» Genre : SongGenre
Methods
+
«constructor» Song(title : string
, artist : string
, length : double
, genre : SongGenre
)
+
ToString() : string
Description of the class members
Fields
There are no fields.
Properties:
This class comprise of four auto-implemented properties with public getters and setters absent. See the UML class diagram above for more details.
Constructor:
public
Song(
string title, string artist, double length, SongGenre genre)
– This constructor that takes four arguments and assigns them to the appropriate properties.
Method:
public
override
string
ToString()
– This public method overrides the ToString()
method of the object class. It does not take any argument and returns a string representation of the object. See the output for hints on the return value of this method.
Page 12
of 21
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Here a foreach loop is recommended for all of the overloaded DisplaySongs
methods.
Programming II
Account: private constructor, const, readonly, static The Library class
This is the front end of the application. This is a static class therefore all the members also have to be static. Remember class members are accessed using the type instead of object reference.
Library
Static Class
Fields
$-
songs : List
<
Song
>
Methods
$+
LoadSongs(filename : string
,) : void
$+
DisplaySongs() : void
$+
DisplaySongs(longerThan : double
) : void
$+
DisplaySongs(genre : SongGenre
) : void
$+
DisplaySongs(artist : string
) : void
Description of class members
Fields:
songs
– this private field is a list of song object is a class variable.
Properties:
There are no properties.
Constructor:
There is no constructor for this class.
Methods
There are four over-loaded methods. Remember that overloading is a technique used to reduce the complexity of the API exposed by the Song
class.
public
static void
DisplaySongs()
– This
is a public class method that does not take any argument
and
displays all the songs in the collection.
public
static void
DisplaySongs(
double
longerThan)
– This is a public class method that takes
a double
argument and displays only songs that are longer than the argument.
Page 13
of 21
The contents of text file
Baby
Justin Bebier
3.35
Pop This is a complex method and rightfully it should be a part of
the Song Class
Programming II
Account: private constructor, const, readonly, static public
static void
DisplaySongs(
SongGenre genre)
– This is a public class method that takes a SongGenre
argument and displays only songs that are of this genre. You must not test for equality, you should use the HasFlags()
method.
public
static void
DisplaySongs(
string artist)
– This is a public class method that takes a string
argument and displays only songs by this artist.
public
static void
LoadSongs(
string fileName)
– This a class method that is public. It
takes a single string argument that represents a text file containing a collection of songs. You will read all
the data and create songs and add it to the songs collection.
Examine the contents of the files for a better understanding
of how this should be done. You will have to read four lines to
create one Song. Your loop body should have four
ReadLine(). The recipe below might clarify things:
Initialize the songs field to a new List of Song
Declare four string variable (title, artist, length, genre) to store the results of four reader.ReadLine()
.
The first ReadLine()
is a string representing the artist of the song. This can and should used as a check for termination condition. If this is empty then there are no more songs to read i.e. it is the end of the file
The next ReadLine()
will get the title
The next ReadLine()
will be a string that
represents the genre. Use the Enum.Parse()
to
get the required type
The next ReadLine()
will be a string that
represents the length. Use the Convert.ToDouble
to get the required type
Use the above four variables to create a Song object.
Add the newly created object to the collection.
And finally do one more read for the title to re-enter the loop.
P.S. Before running the Library
.LoadSongs() you will need to copy the file wk03_lab09_song4.txt
(or any text file that has some songs in the above format) to the bin\debug
folder or where the executable is created.
Page 14
of 21
Programming II
Account: private constructor, const, readonly, static Test Harness
Insert the following code statements in the Main()
method of your Program.cs file:
//To test the constructor and the ToString method
Console
.WriteLine(
new
Song
(
"Baby"
, "Justin Bebier"
, 3.35, SongGenre
.Pop));
//This is first time that you are using the bitwise or. It is used to specify a combination of genres
Console
.WriteLine(
new
Song
(
"The Promise"
, "Chris Cornell"
, 4.26, SongGenre
.Country |
SongGenre
.Rock));
Library
.LoadSongs(
"Week_03_lab_09_songs4.txt"
); //Class methods are invoke with the class name
Console
.WriteLine(
"\n\nAll songs"
);
Library
.DisplaySongs();
SongGenre
genre = SongGenre
.Rock;
Console
.WriteLine($
"\n\n{
genre
} songs"
);
Library
.DisplaySongs(genre);
string
artist = "Bob Dylan"
;
Console
.WriteLine($
"\n\nSongs by {
artist
}"
);
Library
.DisplaySongs(artist);
double
length = 5.0;
Console
.WriteLine($
"\n\nSongs more than {
length
}mins"
);
Library
.DisplaySongs(length);
Page 15
of 21
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Programming II
Account: private constructor, const, readonly, static Program output
Baby by Justin Bebier (Pop) 3.35min
The Promise by Chris Cornell (Rock, Country) 4.26min
All songs
Baby by Justin Bebier (Pop) 3.35min
Fearless by Taylor Swift (Pop) 4.03min
Runaway Love by Ludacris (Pop) 4.41min
My Heart Will Go On by Celine Dion (Pop) 4.41min
Jesus Take The Wheel by Carrie Underwood (Country) 3.31min
If Tomorrow Never Comes by Garth Brooks (Country) 3.40min
Set Fire To Rain by Adele (Soul) 4.01min
Don't You Remember by Adele (Soul) 3.03min
Signed Sealed Deliverd I'm Yours by Stevie Wonder (Soul) 2.39min
Just Another Night by Mick Jagger (Rock) 5.15min
Brown Sugar by Mick Jagger (Rock) 3.50min
All I Want Is You by Bono (Metal) 6.30min
Beautiful Day by Bono (Metal) 4.08min
Like A Rolling Stone by Bob Dylan (Rock) 6.08min
Just Like a Woman by Bob Dylan (Rock) 4.51min
Hurricane by Bob Dylan (Rock) 8.33min
Subterranean Homesick Blues by Bob Dylan (Rock) 2.24min
Tangled Up In Blue by Bob Dylan (Rock) 5.40min
Love Me by Elvis Presley (Rock) 2.42min
In The Getto by Elvis Presley (Rock) 2.31min
All Shook Up by Elvis Presley (Rock) 1.54min
Rock songs
Just Another Night by Mick Jagger (Rock) 5.15min
Brown Sugar by Mick Jagger (Rock) 3.50min
Like A Rolling Stone by Bob Dylan (Rock) 6.08min
Just Like a Woman by Bob Dylan (Rock) 4.51min
Hurricane by Bob Dylan (Rock) 8.33min
Subterranean Homesick Blues by Bob Dylan (Rock) 2.24min
Tangled Up In Blue by Bob Dylan (Rock) 5.40min
Love Me by Elvis Presley (Rock) 2.42min
In The Getto by Elvis Presley (Rock) 2.31min
All Shook Up by Elvis Presley (Rock) 1.54min
Songs by Bob Dylan
Like A Rolling Stone by Bob Dylan (Rock) 6.08min
Just Like a Woman by Bob Dylan (Rock) 4.51min
Hurricane by Bob Dylan (Rock) 8.33min
Subterranean Homesick Blues by Bob Dylan (Rock) 2.24min
Tangled Up In Blue by Bob Dylan (Rock) 5.40min
Songs more than 5mins
Just Another Night by Mick Jagger (Rock) 5.15min
All I Want Is You by Bono (Metal) 6.30min
Like A Rolling Stone by Bob Dylan (Rock) 6.08min
Hurricane by Bob Dylan (Rock) 8.33min
Tangled Up In Blue by Bob Dylan (Rock) 5.40min
Press any key to continue . . .
Page 16
of 21
Programming II
Account: private constructor, const, readonly, static Part D
Concepts
You will learn about properties, enums, static members, lists and default arguments.
Enums
An enum is a user-define type like a class. However, all the members are constants with implied values. It provides an efficient way of defining a set of named integral constants such as the days of the week or months of the year or even the set of pre-defined colors. It supports a more robust programming style e.g. If you have a method that expects a month as an argument of type to a string, you will have do lots of defensive programming, instead define an enum type that has the 12 months. During development, the compiler will do the necessary checks for the require type hence you will do less programming.
Static members
The static decorator when used on a member indicates that member belongs to the type rather than an object. You will access that member using the type rather than an object reference. Static methods or properties may only access other static members. The only instance member that can be accessed is the constructor.
You can recognize an instance member by the absence of the static modifier. Instance methods are able to access other instance members as well as class members.
The TimeFormat Enum
Sometimes you don’t even care about the underlining values as in this case or, (Maritial status: Single, Married, Widowed etc.), (Sale status: Confirmed, Shipped, Paid etc.) TimeFormat
Enum
Values
Mil
Hour12
Hour24
You may define/code this type in the Time.cs
file, but it should be external to the Time
class.
Page 17
of 21
UML Class Diagram
$
static
A class member that is decorated with the static keyword implies that only one copy of this member exists and it is shared by all objects of the class. i.e. If it is changed then all object will see the new value immediately.
A class method can only access other class member (fields/properties/methods)
Programming II
Account: private constructor, const, readonly, static The Time Class
Code the Time class below:
This class comprise of six members: a field, two properties and a
constructor a SetTimeFormat()
and a ToString()
method.
All
the fields are private and all the methods are public Time
Class
Fields
-
$
TIME_FORMAT = TimeFormat
.Hour12 : TimeFormat
Properties
+
«setter absent» Hour : int
+
«setter absent» Minute : int
Methods
+
«constructor» Time(hour = 0 : int
, minute = 0: int
)
+
ToString() : string
+
$
SetFormat(timeFormat : TimeFormat
) : void
Description of class members
Fields:
There are no fields for this class.
Properties:
TIME_FORMAT
– this class variable is of
type TimeFormat
and it represents the
intended format of the ToString()
method. This field has private accessibility
and
it initializes to TimeFormat
.Hour12 at
declaration.
Hour
– this is an integer representing the hour value this object. This property has public read access and the setter is missing.
Minute
– this is an integer representing the minute value this object. This property has public read access and the setter is missing.
Page 18
of 21
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
A switch
statement is probably the simplest way to implement this functionality
This method must be static because it needs to access a static field.
Programming II
Account: private constructor, const, readonly, static Constructor:
public
Time(
int hours = 0, int minutes = 0)
– This is a public constructor that takes two integer arguments with default values of 0
. If checks if the first argument is between 0 and 24 it assigns it to the Hour
property otherwise a value of 0 is assign to the hour
property. Similarly the second argument is check if it is between 0 and 60 then it is assigned to the Minute
property otherwise
a value of 0 is assigned to the Minute
property.
Methods:
public
override
string
ToString()
– This public method overrides the ToString of the object class. It does not take any argument and returns a string representation of the object. The return value will depend on the field TIME_FORMAT
value of the class. So it is a good plan if you use a switch statement to handle the three possibilities. Your switch statement should check the value of the TIME_FORMAT
field.
If you have a Time object with the hours value as 18
and the minutes value as 5
then the print out of the object will be “
1805
” if TIME_FORMAT
is set to TimeFormat
.MIL.
Use the d2 format specifier to get the leading zero.
“
18:05
” if TIME_FORMAT
is set to TimeFormat
.HOUR24
.
Again, use the d2 format
specifier to get the leading zero.
“
6:05 PM
” if TIME_FORMAT
is set to TimeFormat
.HOUR12.
Again, use the d2 format specifier to get the leading zero.
public
static void
SetTimeFormat(
TimeFormat
time_format)
– This a class method that is public. It takes a single TimeFormat
argument and
assigns it to the static TIME_FORMAT
field. Page 19
of 21
Programming II
Account: private constructor, const, readonly, static Test Harness
Insert the following code statements in your Program.cs file:
//create a list to store the objects
List
<
Time
> times = new
List
<
Time
>() { new
Time
(9, 35),
new
Time
(18, 5),
new
Time
(20, 500),
new
Time
(10),
new
Time
()
};
//display all the objects
TimeFormat
format = TimeFormat
.Hour12;
Console
.WriteLine(
$"\n\nTime format is {format}
"
);
foreach
(
Time
t in
times)
{
Console
.WriteLine(t);
}
//change the format of the output
format = TimeFormat
.Mil;
Console
.WriteLine(
$"\n\nSetting time format to {format}
"
);
//SetTimeFormat(TimeFormat) is a class member, so you need the type to access it
Time
.SetTimeFormat(format);
//again display all the objects
foreach
(
Time
t in
times)
{
Console
.WriteLine(t);
}
//change the format of the output
format = TimeFormat
.Hour24;
Console
.WriteLine(
$"\n\nSetting time format to {format}
"
);
//SetTimeFormat(TimeFormat) is a class member, so you need the type to access it
Time
.SetTimeFormat(format);
foreach
(
Time
t in
times)
{ Console
.WriteLine(t);
}
Page 20
of 21
Programming II
Account: private constructor, const, readonly, static Your output will look like below:
Time format is Hour12
9:35 AM
6:05 PM
8:00 PM
10:00 AM
0:00 AM
Setting time format to Mil
0935
1805
2000
1000
0000
Setting time format to Hour24
09:35
18:05
20:00
10:00
00:00
Page 21
of 21
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
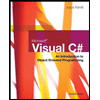
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
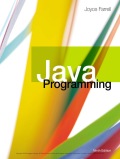
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
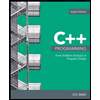
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
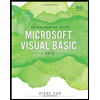
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
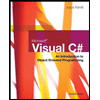
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
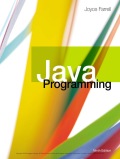
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
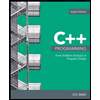
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
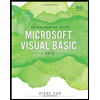
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning