Exam 1 Study Guide STUDENT COPY
docx
keyboard_arrow_up
School
Liberty University *
*We aren’t endorsed by this school
Course
111
Subject
Computer Science
Date
Feb 20, 2024
Type
docx
Pages
6
Uploaded by ChiefSnow8810
Exam 1 Topics to Review
Chapter 6
Know your predefined functions (Table 6-1 pg 350) how to use them and what header is needed
Know how the static cast type conversion works (page 50 in your book, review from 111)
o
Used to explicitly convert one data type to another data type
Know how to calculate the result of a function call within a function call o
Using similar syntax as you would normally
Function Prototypes
o
Know the differences between a function prototype, function call, and function implementation (header) hint: one is a declaration, one is a definition
o
If you need a refresher, watch this video: Buckys C++ Programming Tutorials – 59 https://www.youtube.com/watch?v=SeleR7PDs5Q&list=PLAE85DE8440AA6B83&index=60
o
Know the syntax of a valid function prototype
How do you pass ifstream and ofstream variables to functions? (see section 6-7)
o
Have the separate functions that return each or use
Return {x,y}; within a function
What should you do when you need a function to return more than one value? o
Have the separate functions that return each or use
Return {x,y}; within a function
What are the rules for function overloading? (see section 6-13)
o
Does not include the return type of the function
o
If the number of formal parameters is the same, but the data type of the formal parameters differ in at least one position
Function Call Stack and Activation Records
o
Know the definitions of both. This link will be a good reference for that!
Call stack: a stack data structure that stores information about the active subroutines of a computer program
Activation record: data structure that is created when a procedure/ function is invoked
o
https://flylib.com/books/en/2.253.1/function_call_stack_and_activation_records.html
o
When is an activation record popped off of the call stack?
Whenever a functions returns to its caller
o
When are they pushed onto the stack?
Whenever a function call occurs then a new activation record is created and I will be pushed onto the top of the stack
o
What is included in the function’s activation record?
Return values, param list, control links, access links, saved machine status, local data etc.
You need to know the rules of default arguments (see section 6-14)
o
What can be used as a default argument?
A value provided in a function declaration that is automatically assigned by the compiler if the calling function doesn’t provide a value fir the argument
o
What happens when arguments are omitted in a call to a function with defaults? What are the requirements for this to be valid?
They are unassigned a value
They need to have a value
Chapter 13-5: Function Templates o
When is the ideal situation to use a function template?
When you need to define a function that performs the same logic on multiple different data types
o
When are specializations generated for a function template? Chapter 13-6: Random number Generation
You need to know several things about the rand() function o
What data type does the rand function return? o
Terminology: shifting value = offset, scaling value = interval
Chapter 15 - Recursion
o
What are the pros/cons of recursion vs. iteration? o
What causes recursion to stop? What causes iteration to stop? o
Why do we consider recursion ‘memory-intensive’?
o
Be able to trace through recursive functions to find the answer/output
o
You should also be able to identify the base statement in a recursive function o
What is the difference between direct and indirect recursion? o
You should understand the basic math to calculate a Fibonacci number Chapter 10: Classes o
What is the difference between a class and a struct? What is the benefit of each? o
Constructors
Know what the naming rules are (how do you create a constructor?)
How do you define a default constructor? How many parameters does it have?
What type does a constructor return?
Will the constructor ever call other functions?
How do you overload a constructor?
When does C++ implicitly create a default constructor?
Is a default implicit when a constructor w/ arguments is created?
Understand how default arguments can be used in a constructor and valid ways to then initialize objects using that constructor o
What is a destructor? How do you create one? How many destructors can a class have?
o
Class Functions
What is the definition of a utility function? Why do we need utility functions?
What is the definition of an access function?
How do you call a function in reference to an object?
Get member functions (getters, accessors)
Set member functions (setters, mutators)
Know what the attributes of a class are called (aka data members) and the difference between them and local variables
How do you create an object of a class?
What is the difference between automatic, static, const, or global objects? o
Header and Implementation Files
What is the purpose of a header file? (i.e. what is it used for by the source file)
What phase of the compilation process is the implementation file attached to the header file?
We have not discussed this much so you should review this article! https://www.cs.fsu.edu/~myers/c++/notes/compilation.html
When #including a header file, how does the <> differ from “”?
How do you connect implementation cpp files to the prototypes in the header file? What is that symbol called? o
Constants
What causes a syntax error in terms of having a const function or object?
Scope
o
Scope resolution operator (see section 6-9)
How do you call a base class function from within a derived class? o
Know how to determine where a variable is in scope: block vs. class level
Chapter 16-2: Vector Type Class
o
How to initialize a vector using another vector and how to call the copy constructor
o
Know your basic vector syntax! How do you create a vector? o
Is there a limit to the types of data that can be stored in a vector? No
o
What expression inserts a copy of an element into a vector at the end? o
Can a vector be resized? o
What is the difference between using the at function and the square brackets to access a member of a vector? Which one provides bounds checking? Chapter 8-2: Searching and sorting
o
Know how many comparisons are needed in a linear (sequential search) – know worse case as well as average case
What types of arrays can a linear search be used on?
Any types of arrays (integers, floats, char, strings)
What type of value does the seq. search use to track when an item is found?
It uses a search key. This is a set value of an item being searched for before the search begins
How much of a list (on average) does a sequential search review?
About half of the list
o
Know the algorithms for the bubble sort and the insertion sorts
How many iterations are needed? What do each compare first?
Bubble must scan each as it uses a nested loop to repeatedly swap adjacent elements
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Iteration uses half by picking them up and inserting each in the correct place in the sub array
Know how the bubble sort algorithm and insertion sort algorithm works. You should be able to identify which algorithm is being used based on given code
How many key comparisons are needed for each?
All (bubble)
Half (insertion)
How many item assignments are needed for both?
Must assign all items
o
What is the formula for finding the index of the middle element of an array?
Use the a command to find the length then pull the middle
o
Binary search algorithm – know how it works! List MUST be sorted
Continual incremental division o
Do arrays “need to” be sorted?
No, they can stay as they are. However, it may be useful to sort the contents of arrays
Chapter 11: Composition (HAS-A RELATIONSHIP)
o
When do member objects get constructed? When do host objects get constructed? (Know the order that objects and hosts are constructed in composition!)
Member objects are constructed before the host object
o
When are default constructors *required*?
When the host object needs to be constructed without any argument or initialization
Chapter 11: Inheritance (IS-A RELATIONSHIP)
o
What are the advantages of inheritance compared to a “copy-and-paste” approach
o
What is the separating your header and source code files in a base class? o
You should be able to identify from a list what out of a list would most likely be a base class. You should also be able to identify what types of data would be a good fit for inheritance and what would not be o
When to use inheritance vs. composition, what should be put in base class
o
How do you define a derived class? (syntax)
o
How do you define a multi-argument constructor of a derived class to call the constructor of a base class? (How do you use the member initializer list in the constructor of a derived class?)
o
What is NOT inherited by derived classes? (constructors, destructors, assignment operators – copy constructors) o
Derived classes: What is the dif. between redefining
and overloading
class functions?
o
Know what the member access specifier means and the difference between public, private, and protected
o
Is multiple inheritance possible in C++?
o
Know the definition of: Encapsulation, Code reusability, Inheritance, and Composition
CSIS 112 EXAM 2 STUDY GUIDE
CHAPTER 12
Definitions:
Pointer, Address of operator, dereferencing operator, de-constructor, member-wise initialization, copy constructor, indirection operator, memory leak, static variables, member access operator arrow, member initialization list
Code Output problems
There are LOTS of possible output problems with pointers. Given a value, a pointer, and an address for that pointer, can you find the final output? You need to know what happens when you print a pointer value vs. an address of pointer vs. a dereferenced pointer (like the example we did in class) Other Topics
Initialization: Know how to initialize a pointer variable
Dynamic variables: Know how to create and destroy dynamic variables
What operations are allowed on pointers what two values can be assigned to one?
When you increment a pointer, how much does the value increase by?
Deep vs. Shallow Copy: Know the difference!
Copy Constructor: Know the appropriate syntax
What happens when you dereference a null pointer/an uninitialized pointer?
Understand the ‘Declaring Pointer Variables’ slide from the PowerPoint
How do you properly use the member access operator arrow?
Know the difference between a source code file and object file, where they’re used CHAPTER 8-4
Know how to use the strcpy function and the strncpy function on character arrays
C-Strings: know the definition of aggregate operations
How do the functions of the character-handling library manipulate characters?
Know the strtok_s function, what it does, what it returns, what its’ parameters are CHAPTER 13
Know how to appropriately overload the operators discussed in class
Know the definition of an implicit overload
Know the definition of the this pointer and how to use it
Difference between pre-increment and post-increment overloading
Know the various rules for operator overloading as discussed in class
Rules for which operators can be overloaded as a member vs. as a non-member function. What operators can’t be overloaded? Why do we need friend functions?
What is required for an overloaded operator that takes two different types to be commutative?
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
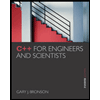
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
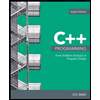
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
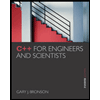
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
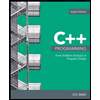
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning