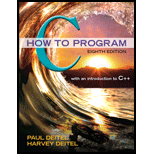
(Recursive Selection Sort) A selection sort searches an array looking for the smallest element in the array. When that element is found, it’s swapped with the first element of the array. The process is then repeated for the subarray, beginning with the second element. Each pass of the array results in one element being placed in its proper location. This sort requires processing capabilities similar to those of the bubble sort—for an array of n elements,

Program Plan-
- Define Header files.
- Define selectionSort()function.
- Define local variables.
- Checking the condition using for() loop till the iteration i is less than 6.
- Display result.
- Checking the condition using if-else() loop.
- Checking the second element with first element.
- Declare main() function.
- Declare local variable.
- Checking the condition using for() loop till the iteration i is less than 6
- Calling selectionSort() function.
- Return successfully to main().
Summary Introduction- (Recursive Selection Sort)A selection sort searches an array looking for the smallest element in the array. When the element is found, it is swapped with the first element of the array. The process is repeated for the sub-array beginning with the second element of the array. Each pass of the array results in one element being placed in its proper location. This sort requires processing capabilities similar to those of bubble sort.
Program Description- The purpose of the program is to implement the recursive selection sort.
Explanation of Solution
Modified program:
/* *This program to implement the recursive selection sort. */ #include<stdio.h> //recursive definition for selcetion sort void selectionSort ( int a[], int size, int i, int j) { //declare local variable int temp; //checking the condtion using if-else loop if(i==size) { //iteration gets executed till value of i is less than 6 for(i=0; i<6; i++) //Displaying result printf("%d ",a[i]); return; //return successfully } else { //checking the condition using if-else() loop if(j<size) { //checking the second element with first //element if(a[i]>a[j]) { temp=a[i]; a[i]=a[j]; a[j]=temp; } //function call //calling function with parameters selectionSort(a,size,i,j+1); } else { //function call selectionSort(a,size,i+1,i+1); } } } //Define main() function int main(int argc, char *argv[]) { //Declare local variables int arr[6],i,min,j,temp; printf("Enter the elements of array\n"); //Asking user to enter elements //Executing for() loop for (i=0; i<6; i++) { printf("Element %d: ",i+1); scanf("%d",&arr[i]); } //sorting the value selectionSort(arr,6,0,1); return 0; //return successfully }
Sample Output-
Sample Output:
Enter the element of array
Element 1:37
Element 2:2
Element 3:6
Element 4:4
Element 5:89
Element 6:8
2 4 6 8 37 89
Want to see more full solutions like this?
Chapter D Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Software Engineering (10th Edition)
Starting Out with Python (4th Edition)
Java How To Program (Early Objects)
- Sorting is a basic issue with arrays in which the goal is to find the target element in the array. Choose from the following options: Falsearrow_forwardARRAY RANDOMIZER Create a program that shuffels all the elements present in an array. To shuffle an array, you need to do at least 500 random swaps of any elements in the array given below. Print the shuffled array in the output. {12, 54, 22, 100, -3, 5, 10, -33, 78, 90, 29, -45, 77, -9, 19, 21} Language: CPParrow_forwardJava programarrow_forward
- What are the advantages and disadvantages of using an unordered array as opposed to an ordered one?arrow_forwardThe given array is arr = {3,4,5,2,1}. The number of iterations in bubble sort and selection sort respectively are, a) 5 and 4 b) 4 and 5 c) 2 and 4 d) 2 and 5arrow_forwardjavaarrow_forward
- Interpolation search By using interpolation search algorithm check if the given element exists in the integer one dimensional array return the index of it ( data structure in java)arrow_forwardRecursivle function to sort two arrays into one in cppExample : Array1[ 1,4,5,2,7,9] , Array2[ 10,3,6] , SortedArray[1,2,3,4,5,6,7,9,10]arrow_forwardNote : Don't Explain .arrow_forward
- arl = np.array([10,20,30,40]) ar2 = np.array([15,6,7]) np.vstack ( (arl,ar2)) The np.stack() function above will O a. Give error b. Stack the two arrays ar1 and ar2 horizontally c. Stack the two arrays ar1 and ar2 vertically O d. Sums the two arrays art and ar2arrow_forwardMultiply left and right array sum. Basic Accuracy: 54.29% Submissions: 12630 Points: 1 Pitsy needs help in the given task by her teacher. The task is to divide a array into two sub array (left and right) containing n/2 elements each and do the sum of the subarrays and then multiply both the subarrays. Example 1: â€arrow_forwardSelect only the correct answers * right wrong An array is a series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding an index to a unique identifier. When working with nested loops, the inner loop changes only after the outer loop is completely finishedarrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
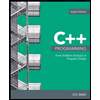
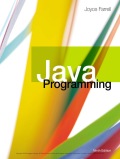
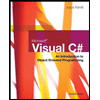
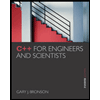