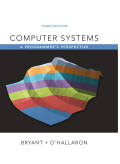
Explanation of Solution
Implementation of “find_fit()” function with First-fit search:
In the “Section 9.9.12 (mm.c)”, add the below “find_fit()” function. The function “find_fit()” is as follows:
// Definition of find_fit() function to find a block fit with size bytes
static void *find_fit(size_t asize)
{
// First-fit search
// Declare the pointer
void *bp;
// For loop to find the fit for first block
for (bp = heap_listp; GET_SIZE(HDRP(bp)) > 0; bp = NEXT_BLKP(bp)) {
// Check the allocation and size
if (!GET_ALLOC(HDRP(bp)) && (asize <= GET_SIZE(HDRP(bp)))) {
// Return the point
return bp;
}
}
// Return null if no fit is available
return NULL;
}
Explanation:
The “find_fit()” function is to find a block fit with size bytes.
- Declare a pointer “bp” to represent which place the block is allocated.
- “for” loop to search the place to fit the first block.
- “if” statement to check the place and size to fit the block.
- Return the pointer.
- Otherwise, return “NULL” if no fit is available.
- “if” statement to check the place and size to fit the block.
The “find_fit()” function is used to implement other simple implicit-list allocator same as first-fit search and to handle and traverse blocks.
Filename: main.c
// Include libraries
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
// Include required header files
#include "csapp.h"
#include "memlib.h"
#include "mm.h"
#include "memlib.c"
#include "mm...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
EBK COMPUTER SYSTEMS
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
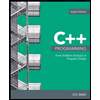
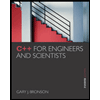