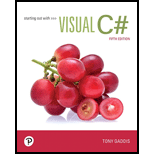
Form design:
- Open Microsoft Visual Studio 2010.
- Select C# and click Windows Forms Application.
- Name the project as Encryption.
- Store the file in desire location.
- The created project is now display with a form in the name of Form1.
- Select the Form1 and add the necessary components.
- In property window, change the Form name and add changes for Form elements properties.
- Click the File menu->Save All.
View of the form design in the IDE:
The form control properties in the properties window are as follows:
Object | Property | Setting |
Form1 | Text | Encryption |
goButton | Text | Encrypt File |
- Add a button control to start the encryption process.
Form design:
- Open Microsoft Visual Studio 2010.
- Select C# and click Windows Forms Application.
- Name the project as Decryption.
- Store the file in desire location.
- The created project is now display with a form in the name of Form1.
- Select the Form1 and add the necessary components.
- In property window, change the Form name and add changes for Form elements properties.
- Click the File menu->Save All.
View of the form design in the IDE:
The form control properties in the properties window are as follows:
Object | Property | Setting |
Form1 | Text | Decryption |
goButton | Text | Decrypt File |
outputListBox | Items | (Empty) |
- Add a button control to start the decryption process.
- Add a list box control to show the decrypted text.
Explanation of Solution
- Add the following code in Form1.cs.
//Importing the required header files
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Decryption
{
public partial class Form1 : Form
{
// Declaring the dictionary to store characters and keys
Dictionary<char, char> codes = new Dictionary<char,
char>()
{
{ 'A', '!' }, { 'a', 'q' }, { 'B', 'a' }, { 'b', 'z'
}, { 'C', '@' }, { 'c', 'w' },{ 'D', 's' }, { 'd',
'x' }, { 'E', '#' }, { 'e', 'c' }, { 'F', 'd' }, {
'f', 'e' }, { 'G', '$' }, { 'g', 'r' }, { 'H', 'f'
}, { 'h', 'v' }, { 'I', '%' }, { 'i', 't' }, { 'J',
'g' }, { 'j', 'b' }, { 'K', '^' }, { 'k', 'y' }, {
'L', 'h' }, { 'l', 'n' }, { 'M', '&' }, { 'm', 'u'
}, { 'N', 'j' }, { 'n', 'm' }, { 'O', '*' }, { 'o',
'i' },{ 'P', 'k' }, { 'p', '<' }, { 'Q', '(' }, {
'q', 'o' }, { 'R', 'l' }, { 'r', '>' },{ 'S', ')' },
{ 's', 'p' }, { 'T', ':' }, { 't', '/' }, { 'U', '-'
}, { 'u', '[' },{ 'V', '"' }, { 'v', '+' }, { 'W',
']' }, { 'w', '\\'}, { 'X', ';' }, { 'x', '.'},{ 'Y',
',' }, { 'y', '|' }, { 'Z', '=' }, { 'z', '_' }, { '
', '~' }, { ',', 'X' },{ '.', '2' }, { '?', '3' }, {
'\n', '\t' }, {'\t', ' ' }};
public Form1()
{
InitializeComponent();
}
//GetInputFileName() opens the OpenFileDialog to select
the file and returns the file name or returns an empty
string if cancel button is clicked
private string GetInputFileName()
{
string infileName = "";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
infileName = openFileDialog.FileName;
}
return infileName;
}
//Defining the DecryptFile() that accepts input file
from the user
private void DecryptFile(string infileName)
{
try
{
// Opening the file
StreamReader infile =
File.OpenText(infileName);
// Encrypting the input file
while (!infile.EndOfStream)
{
// Reading the lines from the input file...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Pearson eText for Starting out with Visual C# -- Instant Access (Pearson+)
- Consider the following relational schema. An employee can work in more than one department; the pct_time field of the Works relation shows the percentage of time that a given employee works in a given department. Emp(eid: integer, ename: string, age: integer, salary: real) Works(eid: integer, did: integer, pct_time: integer) Dept(did: integer, budget: real, managerid: integer) Write the following queries in SQL: a. Print the name of each employee whose salary exceeds the budget of all of the departments that he or she works in. b. Find the enames of managers who manage only departments with budgets larger than $1 million, but at least one department with budget less than $5 million.arrow_forwardConsider the following schema: Suppliers(sid: integer, sname: string, address: string) Parts(pid: integer, pname: string, color: string) Catalog(sid: integer, pid: integer, cost: real) The Catalog relation lists the prices charged for parts by suppliers. Write the following queries in SQL: a. Find the sids of suppliers who charge more for some part than the average cost of that part (averaged over all the suppliers who supply that part). b. Find the sids of suppliers who supply a red part or a green part. c. For every supplier that supplies a green part and a red part, print the name and price of the most expensive part that she supplies.arrow_forwardThe following relations keep track of airline flight information: Flights(flno: integer, from: string, to: string, distance: integer, departs: time, arrives: time, price: integer) Aircraft(aid: integer, aname: string, cruisingrange: integer) Certified(eid: integer, aid: integer) Employees(eid: integer, ename: string, salary: integer) Note that the Employees relation describes pilots and other kinds of employees as well; every pilot is certified for some aircraft, and only pilots are certified to fly. Write each of the following queries in SQL.(Additional queries using the same schema are listed in the exercises for Chapter 4) a. Identify the routes that can be piloted by every pilot who makes more than $100,000. b. Print the name and salary of every nonpilot whose salary is more than the average salary for pilots. c. Print the names of employees who are certified only on aircrafts with cruising range longer than 1000 miles and who are certified on some Boeing…arrow_forward
- Need help making python code for this!arrow_forward2.7 LAB: Smallest of two numbers Instructor note: Note: this section of your textbook contains activities that you will complete for points. To ensure your work is scored, please access this page from the assignment link provided in the CTU Virtual Campus. If you did not access this page via the CTU Virtual Campus, please do so now.arrow_forwardI help understanding this question d'y + 4dy +3y = a, Initial Conditions: y(0) = 5 & y'(0)=0 Where a = 10 a) Find y(t) =yh(t) +yp(t) in time domainIs the system over-damped, under-damped, or critical? b) Find y(t) using Laplace Transformsarrow_forward
- Given f(t)=a sin(ßt) a = 10 & ß = 23 Find the Laplace Transform using the definition F(s) = ∫f(t)e-stdtarrow_forwardPlease do not use any AI tools to solve this question. I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor. No AI-generated responses, please.arrow_forwardObtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).arrow_forward
- I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ? Cisco Packet Tracer 1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardusing r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
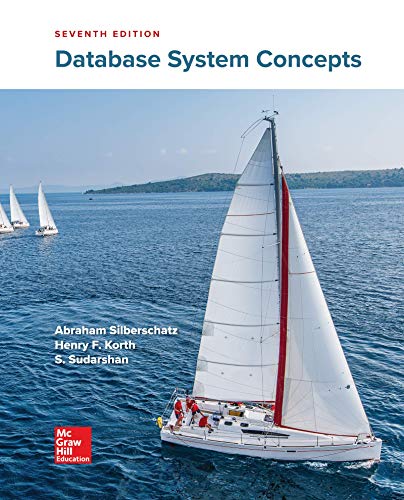
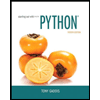
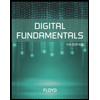
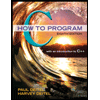
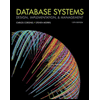
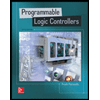