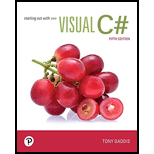
Form design:
- Open Microsoft Visual Studio 2010.
- Select C# and click Windows Forms Application.
- Name the project as Encryption.
- Store the file in desire location.
- The created project is now display with a form in the name of Form1.
- Select the Form1 and add the necessary components.
- In property window, change the Form name and add changes for Form elements properties.
- Click the File menu->Save All.
View of the form design in the IDE:
The form control properties in the properties window are as follows:
Object | Property | Setting |
Form1 | Text | Encryption |
goButton | Text | Encrypt File |
- Add a button control to start the encryption process.
Form design:
- Open Microsoft Visual Studio 2010.
- Select C# and click Windows Forms Application.
- Name the project as Decryption.
- Store the file in desire location.
- The created project is now display with a form in the name of Form1.
- Select the Form1 and add the necessary components.
- In property window, change the Form name and add changes for Form elements properties.
- Click the File menu->Save All.
View of the form design in the IDE:
The form control properties in the properties window are as follows:
Object | Property | Setting |
Form1 | Text | Decryption |
goButton | Text | Decrypt File |
outputListBox | Items | (Empty) |
- Add a button control to start the decryption process.
- Add a list box control to show the decrypted text.
Explanation of Solution
- Add the following code in Form1.cs.
//Importing the required header files
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Decryption
{
public partial class Form1 : Form
{
// Declaring the dictionary to store characters and keys
Dictionary<char, char> codes = new Dictionary<char,
char>()
{
{ 'A', '!' }, { 'a', 'q' }, { 'B', 'a' }, { 'b', 'z'
}, { 'C', '@' }, { 'c', 'w' },{ 'D', 's' }, { 'd',
'x' }, { 'E', '#' }, { 'e', 'c' }, { 'F', 'd' }, {
'f', 'e' }, { 'G', '$' }, { 'g', 'r' }, { 'H', 'f'
}, { 'h', 'v' }, { 'I', '%' }, { 'i', 't' }, { 'J',
'g' }, { 'j', 'b' }, { 'K', '^' }, { 'k', 'y' }, {
'L', 'h' }, { 'l', 'n' }, { 'M', '&' }, { 'm', 'u'
}, { 'N', 'j' }, { 'n', 'm' }, { 'O', '*' }, { 'o',
'i' },{ 'P', 'k' }, { 'p', '<' }, { 'Q', '(' }, {
'q', 'o' }, { 'R', 'l' }, { 'r', '>' },{ 'S', ')' },
{ 's', 'p' }, { 'T', ':' }, { 't', '/' }, { 'U', '-'
}, { 'u', '[' },{ 'V', '"' }, { 'v', '+' }, { 'W',
']' }, { 'w', '\\'}, { 'X', ';' }, { 'x', '.'},{ 'Y',
',' }, { 'y', '|' }, { 'Z', '=' }, { 'z', '_' }, { '
', '~' }, { ',', 'X' },{ '.', '2' }, { '?', '3' }, {
'\n', '\t' }, {'\t', ' ' }};
public Form1()
{
InitializeComponent();
}
//GetInputFileName() opens the OpenFileDialog to select
the file and returns the file name or returns an empty
string if cancel button is clicked
private string GetInputFileName()
{
string infileName = "";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
infileName = openFileDialog.FileName;
}
return infileName;
}
//Defining the DecryptFile() that accepts input file
from the user
private void DecryptFile(string infileName)
{
try
{
// Opening the file
StreamReader infile =
File.OpenText(infileName);
// Encrypting the input file
while (!infile.EndOfStream)
{
// Reading the lines from the input file...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
EBK STARTING OUT WITH VISUAL C#
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
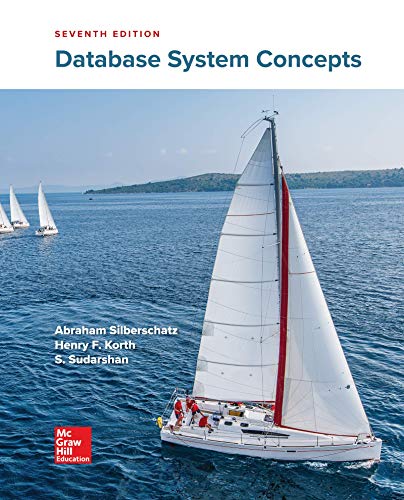
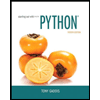
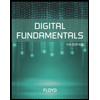
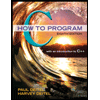
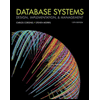
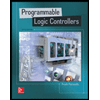