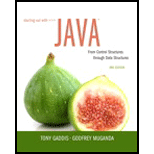
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 9, Problem 5PC
Program Plan Intro
Password Verifier
Program Plan:
The detail of “PasswordDemo” class is shown below:
- In the “main()” method,
- Declare the required variable.
- Read the password input from user.
- Check whether the password is valid or not. If yes,
- Display the error message to user.
- Otherwise, display the valid password message to user.
- Exit the system.
The detail of “PasswordVerifier” class is shown below:
- Declare and initialize the minimum password length.
- In the isValid() method,
- Declare the variable.
- Check whether the password is valid or not. If yes,
- Set the status as “true”.
- Otherwise, set the status as “false”.
- Return the status.
- In the hasUpperCase() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string has at least one uppercase character. If yes, set the status as “true”.
- Return the status.
- In the hasLowerCase() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string has at least one lowercase character. If yes, set the status as “true”.
- Return the status.
- In the hasDigit() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string contains numeric digit. If yes, set the status as “true”.
- Return the status.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - Prob. 13MCCh. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
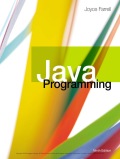
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
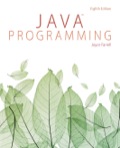
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage