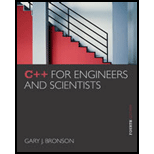
C++ for Engineers and Scientists
4th Edition
ISBN: 9781133187844
Author: Bronson, Gary J.
Publisher: Course Technology Ptr
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 9, Problem 4PP
(Useful utility) Modify the
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
1. (Functions with Loop and Branches)
Write a C program that uses the function, PrintShampoo Instructions(), with int parameter
numCycles, and void return type.
If numCycles is less than 1, print "Too few.".
Else If more than 4, print "Too many.".
Else, print "N: Lather and rinse." for numCycles times, where N is the cycle number,
followed by "Done.". End with a new line. [hint: use "for" loop from 1 to numCycles
times to get desired display]
Example output with input 0: Too few
Example output with input 5: Too many
Example output with input 4:
1: Lather and rinse.
2: Lather and rinse.
3: Lather and rinse.
4: Lather and rinse.
Done.
#include
/* Your user-defined function goes here */
int main(void) {
int user Cycles;
scanf("%d", &userCycles);
PrintShampoo Instructions (user Cycles);
return 0;
}
2. (Functions with C string parameters)
Write a program in C that uses the function, MakeSentenceExcited() to replace any period (full
stop or comma) with an exclamation point.
If the input string is: I told my computer I needed a break. It replied "I am sorry, I'm not
programmed for that function."
The output will be:
I told my computer I needed a break! It replied "I am sorry! I'm not programmed for that
function!"
Assign a string size of 200 or above. Use "fgets()" to take the string input. Don't forget to
include the string header file.
Hint: A very similar example 3 problem is given in the lab slide. Try to follow that example.
• Population of a town today is 10000. The population has decreased steadily at the rate of
1% per year for last 10 years. Write a program to determine the population at the end of each
year in the last decade. (Solve it with C program in Code Block).
Comment/Discussion on the obtained results and discrepancies (if any).
Chapter 9 Solutions
C++ for Engineers and Scientists
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Python)Write a function that receives an integer. The function must return a string containing thehexadecimal representation of the integer.arrow_forward(b) Good Programming practices help in improving programs readability and understandability both for a programmer and for a general user. What changes would you make in the following program, written by a beginner, keeping in view the good programming practices. You are also required to write the output of the program if a user wants to find factorial of number 6. Note: The Program finds/calculates factorial of a number using a user defined recursive function. #include<iostream> using namespace std; int f(int n); int main() {int n; cout << "Enter: "; cin >> n; cout << "Answer = " <<factorial(n); return 0; int f(int n) { } if(n > 1)return n * f(n - 1); else return 1; }arrow_forward(b) Good Programming practices help in improving programs readabilityand understandability both for a programmer and for a general user.What changes would you make in the following program, written by a beginner, keeping in view the good programming practices.You are also required to write the output of the program if a user wants to find factorial of number 6. Note: The Program finds/calculates factorial of a number using a user defined recursive function. #include<iostream> using namespace std; int f(int n); int main() { int n;cout << "Enter: ";cin >> n;cout << "Answer = " <<factorial(n);return 0;}int f(int n){if(n > 1)return n * f(n - 1);elsereturn 1;}arrow_forward
- (b) Good Programming practices help in improving programs readabilityand understandability both for a programmer and for a general user.What changes would you make in the following program, written by a beginner, keeping in view the good programming practices.You are also required to write the output of the program if a user wants to find factorial of number 6.Note: The Program finds/calculates factorial of a number using a user defined recursive function.#include<iostream>using namespace std;int f(int n);int main(){int n;cout << "Enter: ";cin >> n;cout << "Answer = " <<factorial(n);return 0;}int f(int n){if(n > 1)return n * f(n - 1);elsereturn 1;}arrow_forward[THIS IS NOT GRADED] The question mentions the bouncy program; this is the bouncy program I have written that computes the total distance traveled by the ball given initial height, bounciness index, and number of bounces: height = float(input("Enter the height from which the ball is dropped: ")) bounciness = float(input("Enter the bounciness index of the ball: ")) distance = 0 bounces = int(input("Enter the number of times the ball is allowed to continue bouncing: ")) for eachPass in range(bounces): distance += height height *= bounciness distance += height print('\nTotal distance traveled is:', distance, 'units.') I will attatch the pre-existing code that the question provided. Thank you for your help.arrow_forward(Python matplotlib or seaborn) CPU Usage We have the hourly average CPU usage for a worker's computer over the course of a week. Each row of data represents a day of the week starting with Monday. Each column of data is an hour in the day starting with 0 being midnight. Create a chart that shows the CPU usage over the week. You should be able to answer the following questions using the chart: When does the worker typically take lunch? Did the worker do work on the weekend? On which weekday did the worker start working on their computer at the latest hour? cpu_usage = [ [2, 2, 4, 2, 4, 1, 1, 4, 4, 12, 22, 23, 45, 9, 33, 56, 23, 40, 21, 6, 6, 2, 2, 3], # Monday [1, 2, 3, 2, 3, 2, 3, 2, 7, 22, 45, 44, 33, 9, 23, 19, 33, 56, 12, 2, 3, 1, 2, 2], # Tuesday [2, 3, 1, 2, 4, 4, 2, 2, 1, 2, 5, 31, 54, 7, 6, 34, 68, 34, 49, 6, 6, 2, 2, 3], # Wednesday [1, 2, 3, 2, 4, 1, 2, 4, 1, 17, 24, 18, 41, 3, 44, 42, 12, 36, 41, 2, 2, 4, 2, 4], # Thursday [4, 1, 2, 2, 3, 2, 5, 1, 2, 12, 33, 27, 43, 8,…arrow_forward
- (Rounding Numbers) Function floor can be used to round a number to a specific decimal place. The statementy = floor(x * 10 + 0.5) / 10;rounds x to the tenths position (the first position to the right of the decimal point). The statementy = floor(x * 100 + 0.5) / 100;rounds x to the hundredths position (the second position to the right of the decimal point). Write a program that defines fourfunctions to round a number x in various ways:A. roundToInteger(number)B. roundToTenths(number)C. roundToHundredths(number)D. roundToThousandths(number)For each value read, your program should print the original value, the number rounded to the nearest integer, the number rounded to the nearest tenth, the number rounded to the nearest hundredth and the number rounded to the nearest thousandth.arrow_forward(Python) Without giving me the code, can you give me some advice or tips on approaching this?arrow_forwardProblem 4. (Regular Expressions-using Python re or Java regex) Write a regular expression for the following word patterns: 1. Word starts with a 'q'. Word has 'th'. 2. 3. Word has an 'q' or a 'Q'. 4. Word starts with an 'q' or an 'Q'. 5. Word has both 'o' and 'u' in it. 6. Word does not have an 'a'arrow_forward
- (C PROGRAMMING ONLY!) 6. Teaching the Dog To Sitby CodeChum Admin Ever since I was a kid, I've always wanted to see a dog sit because it's so cute ? Let's create a function that lets a dog sit! Instructions: In the code editor, you are provided with the definition of a struct Dog. This struct needs an integer value for its age. Furthermore, in the main, a Dog is already created for you and is passed to a call to a function, sit().Your task is to declare and define the function sit() which has the following details:Return type - voidName - sitParameters - 1 DogDescription - prints the message, "Arf arf! My age is <AGE_OF_DOG> and I know how to sit!"Input 1. The age of the Dog Output Enter age of Dog: 3Arf arf! My age is 3 and I know how to sit!arrow_forward(Q1)This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you.arrow_forwardQ2) (Perfect Numbers) An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 = 1 + 2 + 3. Write a function perfect that determines if parameter number is a perfect number. Use this function in a program that determines and prints all the perfect numbers between 1 and 1000. Print the factors of each perfect number to confirm that the number is indeed perfect. Challenge the power of your computer by testing numbers much larger than 1000.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
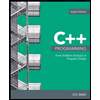
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Literals in Java Programming; Author: Sudhakar Atchala;https://www.youtube.com/watch?v=PuEU4S4B7JQ;License: Standard YouTube License, CC-BY
Type of literals in Python | Python Tutorial -6; Author: Lovejot Bhardwaj;https://www.youtube.com/watch?v=bwer3E9hj8Q;License: Standard Youtube License