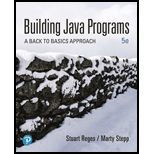
Concept explainers
Interaction with super class
Program plan:
- Create a class “Employee”,
- Define the method “getHours()” to return “40”.
- Define the method “getSalary()” to return “40000.0”.
- Define the method “getVacationDays()” to return “10”.
- Define the method “getVacationForm()” to return “yellow”.
- Create a class “Marketer” that extends the super class “Employee”,
- Define the method “advertise()” ,
- Print the string.
- Define the method “getSalary()”,
- Call the method “getSalary()” of super class and return the updated value.
- Define the method “main()”,
- Create object for “Marketer” class.
- Call the method “advertise()”.
- Print the value returned from “getsalary()”.
- Define the method “getSalary()”,
- Print the string.
- Define the method “advertise()” ,

This program demonstrates the class that accompanies the other law firm classes.
Explanation of Solution
Program:
File name: “Employee.java”
//Create a class
public class Employee
{
//Define the method
public int getHours()
{
//Retunr "40"
return 40;
}
//Define the method
public double getSalary()
{
//Return "40000.0"
return 40000.0;
}
//Define the method
public int getVacationDays()
{
//Return "10"
return 10;
}
//Define the method
public String getVacationForm ()
{
//Return "yellow"
return " yellow";
}
}
File name: “Marketer.java”
//Create a class
public class Marketer extends Employee
{
//Define the method advertise()
public static void advertise()
{
//Print the string
System.out.println("Act now, while supplies last!");
}
//Define the method
public double getSalary()
{
/*Call the method of super class and return the updated value*/
return super.getSalary() + 10000;
}
//Define the main() method
public static void main(String[] args)
{
//Create object
Marketer m=new Marketer();
//Call the method
m.advertise();
//Print the value returned from getSalary()
System.out.println("$"+m.getSalary());
}
}
Output:
Act now, while supplies last!
$50000.0
Want to see more full solutions like this?
Chapter 9 Solutions
MyProgrammingLab with Pearson eText -- Access Code Card -- for Building Java Programs
- Show the correct stereochemistry when needed!! mechanism: mechanism: Show the correct stereochemistry when needed!! Br NaOPh diethyl ether substitutionarrow_forwardIn javaarrow_forwardKeanPerson #keanld:int #keanEmail:String #firstName:String #lastName: String KeanAlumni -yearOfGraduation: int - employmentStatus: String + KeanPerson() + KeanPerson(keanld: int, keanEmail: String, firstName: String, lastName: String) + getKeanld(): int + getKeanEmail(): String +getFirstName(): String + getLastName(): String + setFirstName(firstName: String): void + setLastName(lastName: String): void +toString(): String +getParkingRate(): double + KeanAlumni() + KeanAlumni(keanld: int, keanEmail: String, firstName: String, lastName: String, yearOfGraduation: int, employmentStatus: String) +getYearOfGraduation(): int + setYearOfGraduation(yearOfGraduation: int): void +toString(): String +getParkingRate(): double In this question, write Java code to Create and Test the superclass: Abstract KeanPerson and a subclass of the KeanPerson: KeanAlumni. Task 1: Implement Abstract Class KeanPerson using UML (10 points) • Four data fields • Two constructors (1 default and 1 constructor with all…arrow_forward
- Plz correct answer by best experts...??arrow_forwardQ3) using the following image matrix a- b- 12345 6 7 8 9 10 11 12 13 14 15 1617181920 21 22 23 24 25 Using direct chaotic one dimension method to convert the plain text to stego text (hello ahmed)? Using direct chaotic two-dimension method to convert the plain text to stego text?arrow_forward: The Multithreaded Cook In this lab, we'll practice multithreading. Using Semaphores for synchronization, implement a multithreaded cook that performs the following recipe, with each task being contained in a single Thread: 1. Task 1: Cut onions. a. Waits for none. b. Signals Task 4 2. Task 2: Mince meat. a. Waits for none b. Signals Task 4 3. Task 3: Slice aubergines. a. Waits for none b. Signals Task 6 4. Task 4: Make sauce. a. Waits for Task 1, and 2 b. Signals Task 6 5. Task 5: Finished Bechamel. a. Waits for none b. Signals Task 7 6. Task 6: Layout the layers. a. Waits for Task 3, and 4 b. Signals Task 7 7. Task 7: Put Bechamel and Cheese. a. Waits for Task 5, and 6 b. Signals Task 9 8. Task 8: Turn on oven. a. Waits for none b. Signals Task 9 9. Task 9: Cook. a. Waits for Task 7, and 8 b. Signals none At the start of each task (once all Semaphores have been acquired), print out a string of the task you are starting, sleep for 2-11 seconds, then print out a string saying that you…arrow_forward
- Programming Problems 9.28 Assume that a system has a 32-bit virtual address with a 4-KB page size. Write a C program that is passed a virtual address (in decimal) on the command line and have it output the page number and offset for the given address. As an example, your program would run as follows: ./addresses 19986 Your program would output: The address 19986 contains: page number = 4 offset = 3602 Writing this program will require using the appropriate data type to store 32 bits. We encourage you to use unsigned data types as well. Programming Projects Contiguous Memory Allocation In Section 9.2, we presented different algorithms for contiguous memory allo- cation. This project will involve managing a contiguous region of memory of size MAX where addresses may range from 0 ... MAX - 1. Your program must respond to four different requests: 1. Request for a contiguous block of memory 2. Release of a contiguous block of memory 3. Compact unused holes of memory into one single block 4.…arrow_forwardusing r languagearrow_forwardProgramming Problems 9.28 Assume that a system has a 32-bit virtual address with a 4-KB page size. Write a C program that is passed a virtual address (in decimal) on the command line and have it output the page number and offset for the given address. As an example, your program would run as follows: ./addresses 19986 Your program would output: The address 19986 contains: page number = 4 offset = 3602 Writing this program will require using the appropriate data type to store 32 bits. We encourage you to use unsigned data types as well. Programming Projects Contiguous Memory Allocation In Section 9.2, we presented different algorithms for contiguous memory allo- cation. This project will involve managing a contiguous region of memory of size MAX where addresses may range from 0 ... MAX - 1. Your program must respond to four different requests: 1. Request for a contiguous block of memory 2. Release of a contiguous block of memory 3. Compact unused holes of memory into one single block 4.…arrow_forward
- using r languagearrow_forwardWrite a function to compute a Monte Carlo estimate of the Beta(3, 3) cdf, and use the function to estimate F(x) for x = 0.1,0.2,...,0.9. Compare the estimates with the values returned by the pbeta function in R.arrow_forwardWrite a function to compute a Monte Carlo estimate of the Gamma(r = 3, λ = 2) cdf, and use the function to estimate F(x) for x = 0.2, 0.4, . . . , 2.0. Compare the estimates with the values returned by the pgamma function in R.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
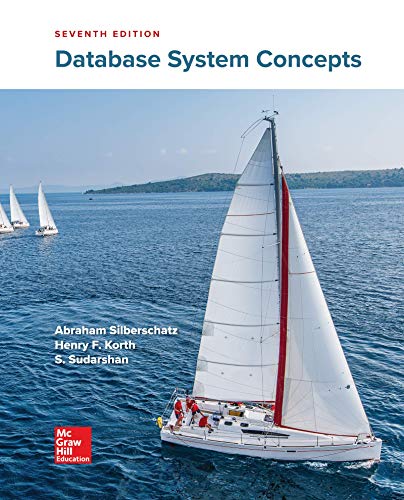
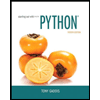
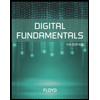
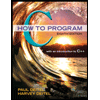
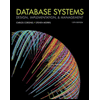
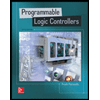