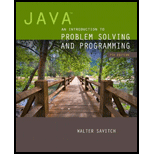
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8.4, Problem 27STQ
Program Plan Intro
Overriding method:
- • If the subclass inherits a method from its superclass, the overriding of method is possible. The overriding method is used to define a methods or behavior that’s specific to the superclass type.
- • Based on the requirement, subclass can be implemented on a superclass method.
- • Generally, the overriding method overrides the functionality of a current method.
- • The private method cannot be override, because this method is not accessible outside the class.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Which one is NOT an example of software reuse?
A I have an abstract class Game, I will define Chess, Backgammon, and Checkers as subclasses of class Game.
B I have a LinkedList implementation, I will use it to implement a Set class through delegation.
C I need to develop a structure that has recursive composition, I will make use of composite design pattern.
D I have already written a code segment that scans a list and finds duplicates, I will copy and paste that code segment into another program that I am developing.
E I have an implementation of List class which contains elements of type integer, I will use it to develop a list that contains elements of type Car.
Programming Exercise 8 asks you to redefine the class to implement the nodes of a linked list so that the instance variables are private. Therefore, the class linkedListType and its derived classes unorderedLinkedList and orderedLinkedList can no longer directly access the instance variables of the class nodeType. Rewrite the definitions of these classes so that these classes use the member functions of the class nodeType to access the info and link fields of a node. Also write programs to test various operations of the classes unorderedLinkedList and orderedLinkedList.
template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type>…
Provide me complete and correct solution thanks 3
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is the difference between what you can do in...Ch. 8.5 - Prob. 36STQCh. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Prob. 8PPCh. 8 - Prob. 9PPCh. 8 - Prob. 10PP
Knowledge Booster
Similar questions
- In C++, How can I use and apply using templates in my programming assignments? Such as how and when to using templates? Because, I know how to implement them, and theoratically to why we use them, but I am not sure how can I use this in code that involves in polymorphism, such as for example lets say we have a class called player and another class called Roles, and lets say that class Roles is to inherit from Player. How would I use templates in this case? Or even in some cases what is the mindset or logical thinking should I have when developing code involving templates?arrow_forwardC++ programming design a class to implement a sorted circular linked list. The usual operations on a circular list are:Initialize the list (to an empty state). Determine if the list is empty. Destroy the list. Print the list. Find the length of the list. Search the list for a given item. Insert an item in the list. Delete an item from the list. Copy the list. Write the definitions of the class circularLinkedList and its member functions (You may assume that the elements of the circular linked list are in ascending order). Also, write a program to test the operations of your circularLinkedList class. NOTE: The nodes for your list can be defined in either a struct or class. Each node shall store int values.arrow_forwardDesign and implement a Java class named Book with two data members: title and price. The class should have suitable constructors, get/set methods, and the toString method. Design and implement another Java class named BookShelf which has an ArrayList data member named bookList to store books. The class should have suitable constructors, get/set methods, and the toString method, as well as methods for people to add a book (prototype: addBook(Book book)), remove a book (prototype: removeBook(Book book)), and search for a book (prototype: findBook(Book book)). Test the two classes by creating a Bookshelf object and five Book objects. Add the books to the bookshelf. Display the contents of the bookshelf. Test the removeBook and findBook methods as well. Take screenshots of your tests and submit them with your Java code (not the whole project)arrow_forward
- Java Code: In this assignment, we are going to start working on the parser. The lexer’s job is to make “words”, the parser’s job is to make sure that those tokens are in an order that makes sense and create a data structure in memory that represents the program. The Tokens are in a 1-dimensional structure (array or list). The parser’s output will be a 2-dimensional tree. The parser works using recursive descent. We are encoding the rules into the structure of the program. There are a few simple rules that we will follow in writing our parser: Each function represents some “phrase” in our language, like “if statement” or “assignment statement”. Each function must either succeed or fail: On success, remove Tokens from the list and output a tree node On failure, leave the list unchanged and return null. When there are alternatives, the function must call each alternative’s function until it finds one that is not null. Create a Parser class (does not derive from anything). It must…arrow_forwarduse java (ArrayList, inheritance, polymorphism, File I/O and basic Java) Student class: First, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they…arrow_forwardplease in c++ im really struggling and would appreciate the help ill give like, down below i will leave the ADT LinkedSorterLists.h and .cpp c Question: Write an implementation using the ADT LinkedSorterList (textbook code) defining a list of runner objects. Create a Runner class with two attributes one is the time (hh:mm:ss) of the Time datatype and the other is the runner's name. The Time Class has three integer attributes and stores the time in military time format (Ex. 13:45:34). Your program determines the places of the runners. 16. Running the Race Write a program that asks for the names of three runners and the time it took each of them to finish a race. The program should display who came in first, second, and third place. Input Validation: Only accept positive numbers for the times. (Gaddis T. 2018)arrow_forward
- Write simple C++ code as I am a beginnerarrow_forwardPlease help answer this Java multiple choice question. Assume you are a developer working on a class as part of a software package for running artificial neural networks. The network is made of Nodes (objects that implement a Node interface) and Connections (objects that implement a Connection interface). To create a new connection you call the constructor for a concrete class that implements the Connection interface, passing it three parameters: Node origin, Node target, and double weight. The weight is a double that is greater than or equal to 0, and represents how strongly the target node should weigh the input from the origin node. So with a weight of 0.1 the target node will only slightly weight the input from the origin, but with a weight of 725.67 the target node will strongly weight the input. The method throws an IllegalArgumentException. Which of the following are true? A. All of the above are true. B. The code:Connection c = new ConvLayerConnection(origin, target,…arrow_forwardIn C++, Write the implementation for the methods of the Node class including the constructor. The constructor should set value to the passed in parameter, and set the value of the next pointer to NULL. getNext() should return the value of the next pointer. setNext() should set the value of the next pointer to the parameter that is passed in. In the main() function below (where it says // Step 2 code here), write the code to make a list of 10 Node objects. To do this, make a root pointer which is a pointer to a Node object, and then use a loop to create new Nodes, hooking up the list using setNext() and getNext() for each node. Set the value of each new Node to be the even numbers 2 through 18 (in addition to the root node which has value 0). Make sure that your nodes are linked together! Write code to step through your linked list (where it says // Step 3 code here), printing the value of each node. Write code to clean up the list (where it says // Step 4 code here). Print a…arrow_forward
- Suppose that you are required to create a C++ class with additional method, instead of a struct in a linked list. You are required to use a class as a node.Write the definition of the node as a class, so that nodeType is a class and member variables are private, with its proper methods and properties. The node should be a template.arrow_forwardpresentation: Show how to use the diamond operator when creating instances of generic classes in javaarrow_forwardWrite a COMPLETE C++ code by using only #include library, Includes an explanation of the code and its working details completely. It must contain the pictures of each and every functions results. Write a menu driven program to implement the linked list that has the following operations: Insert(data) Search(key) Delete(key) Your Linked List must accommodate Trader type of data. A bloom filter that acts as a membership data structure giving a very quick heads up on search of an item. Data is key/value fields for a Trader type of data tradernumber is the key or identifying field and other data fields name and department are value fields. All these fields combine to form the data and for Search and Delete all we need from the user is the key field.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
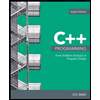
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning