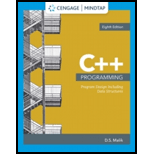
EP MINDTAPV2.0 FOR MALIK'S C++ PROGRAMM
8th Edition
ISBN: 9780357425299
Author: Malik
Publisher: CENGAGE CO
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 8, Problem 9SA
Explanation of Solution
The computations proceed as follows:
list[0] = 1
list[1] = 2
Inside the for loop:
1stiteration:
i = 2
list[2] = list[2-1] * list[2-2] = list[1] * list[0] = 2 * 1 = 2
2nditeration:
i = 3
list[3] = list[3-1] * list[3-2] = list[2] * list[1] = 2 * 2 = 4
3rditeration:
i = 4
list[4] = list[4-1] * list[4-2] = list[3] * list[2] = 4 * 2 = 8
4thiteration:
i = 5
list[5] = list[5-1] * list[5-2] = list[4] * list[3] = 8 * 4...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What is stored in list after the following C++ code executes? int list[8];list[0] = 1;list[1] = 2;
for (int i = 2; i < 8; i++){list[i] = list[i – 1] * list[i – 2];if (i > 5)list[i] = list[i] - list[i - 1];}
C++ ProgrammingActivity: Deque Linked List
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow.
#include "deque.h"
#include "linkedlist.h"
#include <iostream>
using namespace std;
class DLLDeque : public Deque {
DoublyLinkedList* list;
public:
DLLDeque() {
list = new DoublyLinkedList();
}
void addFirst(int e) {
list->addAt(e,1);
}
void addLast(int e) {
list->addAt(e,size()+1);
}
int removeFirst() {
return list->removeAt(1);
}
int removeLast() {
return list->removeAt(size());
}
int size(){
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
// OPTIONAL: a helper method to help you debug
void print() {…
C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow
#include "stack.h"
#include "linkedlist.h"
// SLLStack means Singly Linked List (SLL) Stack
class SLLStack : public Stack {
LinkedList* list;
public:
SLLStack() {
list = new LinkedList();
}
void push(char e) {
list->add(e);
return;
}
char pop() {
char elem;
elem = list->removeTail();
return elem;
}
char top() {
char elem;
elem = list->get(size());
return elem;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
};
Chapter 8 Solutions
EP MINDTAPV2.0 FOR MALIK'S C++ PROGRAMM
Ch. 8 - Mark the following statements as true or false. A...Ch. 8 - Consider the following declaration: (1,2) double...Ch. 8 - Identify error(s), if any, in the following array...Ch. 8 - Determine whether the following array declarations...Ch. 8 - Prob. 5SACh. 8 - Write C+ + statement(s) to do the following: (1,...Ch. 8 - Prob. 7SACh. 8 - Prob. 8SACh. 8 - Prob. 9SACh. 8 - Prob. 10SA
Ch. 8 - Prob. 11SACh. 8 - Correct the following code so that it correctly...Ch. 8 - Prob. 13SACh. 8 - Suppose that points is an array of 10 components...Ch. 8 - Determine whether the following array declarations...Ch. 8 - Prob. 17SACh. 8 - Prob. 19SACh. 8 - Prob. 1PECh. 8 - Prob. 2PECh. 8 - Write a C+ + function, lastLargestIndex that takes...Ch. 8 - Write a program that reads a file consisting of...Ch. 8 - Prob. 6PECh. 8 - Write a program that allows the user to enter the...Ch. 8 - Write a program that uses a two-dimensional array...Ch. 8 - Prob. 12PECh. 8 - Write a program to calculate students average test...
Knowledge Booster
Similar questions
- In c++ pleasearrow_forwardfor c++ please thank you please type the code so i can copy and paste easily thanks. 4, List search Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program. here is my previous programming challange. #include <iostream> using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next =…arrow_forwardstruct insert_at_back_of_dll { // Function takes a constant Book as a parameter, inserts that book at the // back of a doubly linked list, and returns nothing. void operator()(const Book& book) { / // TO-DO (2) |||| // Write the lines of code to insert "book" at the back of "my_dll". // // // END-TO-DO (2) ||| } std::list& my_dll; };arrow_forward
- In OCaml programming language:arrow_forwardc++ program please don't use any builtin functionarrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forward
- c++ program use linked listarrow_forwardstruct remove_from_front_of_dll { // Function takes no parameters, removes the book at the front of a doubly // linked list, and returns nothing. void operator()(const Book& unused) { //// TO-DO (13) |||| // Write the lines of code to remove the book at the front of "my_dll", // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. ///// END-TO-DO (13) //// } std::list& my_dll; };arrow_forwardstruct Node { int data; Node * next; }; Node • head; a. Write a function named addNode that takes in a variable of type int and inserts it at the head of the list. b. Write a function named removeNode that removes a node at the head of the list.arrow_forward
- If you have the following node declaration: struct Node { int number; struct Node * next; }; typedef struct Node node; node *head,*newNode; Write a C program that contains the following functions to manipulate this linked list : First function: Adding the odd numbers to the beginning of the list and even numbers to the end of the list until -1 is entered from keyboardarrow_forwardNote: Code in c++ Consider the following statements: unorderedLinkedList myList; unorderedLinkedList subList; Suppose myList points to the list with elements 34 65 27 89 12 (in this order). The statement: myList.divideMid(subList); divides myList into two sublists: myList points to the list with the elements 34 65 27, and subList points to the sublist with the elements 89 12.arrow_forwardcan you help me with this in c++? thanksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
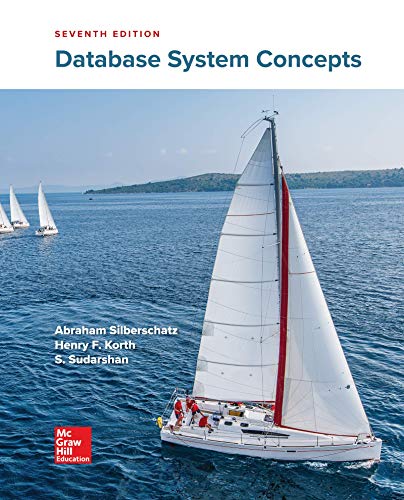
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
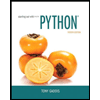
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
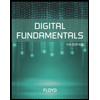
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
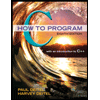
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
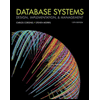
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
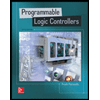
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education