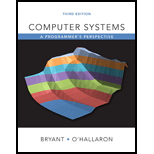
Computer Systems: A Programmer's Perspective (3rd Edition)
3rd Edition
ISBN: 9780134092669
Author: Bryant, Randal E. Bryant, David R. O'Hallaron, David R., Randal E.; O'Hallaron, Bryant/O'hallaron
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 8.17HW
Program Plan Intro
Given code:
//Include necessary header files
#include <stdio.h>
#include "csapp.h"
//Define a main function
int main()
{
//Variable declaration
int status;
pid_t pid;
//Print the statement
printf("Hello\n");
//Call the method fork() and assign it to the variable pid
pid = fork();
//Print the statement
printf("%d\n",!pid);
//Check, pid is not equal to zero
if (pid != 0)
{
//True, check the condition
if (waitpid(-1, &status, 0) > 0)
{
//True, check the condition
if (WIFEXITED(status) != 0)
//True, print the statement
printf("%d\n" , WEXITSTATUS(status));
}
}
//Otherwise, print the statement
printf("Bye\n");
//Exit
exit(2);
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(a) A ssume that five generation unıts with third order cost
function (F, (P) = A; P+ B;P+C; P; + D;) are in the circuit.
Write a computer program using any arbitrary programming
language (MATLAB, C++, C#, Python,.) to calculate economic
load dispatch (ELD) using first order gradient method.
Note that all parameters and variables should be defined
inside the
program
(at tirst limes) such that units' charactenistics
and demand can be changed easily. Neglect grid losses.
O using lambda - iteration method.
Problem No.1
Given: Eight (8) Queens and 8 x 8 chess board
Goal: Arrange the 8 Queens in the 8 x 8 chess board as given in the layout above such that only one (1)
Queen will be placed in any column, row, and diagonal/s. That is, no two or more Queens are placed in
any column, row, and diagonal/s.
Solve the problem set by the giving the solution, which is the sequence of steps, that will satisfy the
goal.
NOTE: The respective Queens are move in their column location only. For example, QA is move along
column A, QB column B, and so on. However, you need to give the remaining moves to attain the
required goal. Set the column labels from left to right A, B, C, D, E, F, G, and H, respectively. Set the row
labels from top to bottom 8, 7, 6, 5, 4, 3, 2, and 1, respectively.
Let f(x)=√√x, g(x) = x + 12 and h(x)
=
Compute the following function value.
(#)(4)
Chapter 8 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Ch. 8.2 - Prob. 8.1PPCh. 8.4 - Prob. 8.2PPCh. 8.4 - Prob. 8.3PPCh. 8.4 - Prob. 8.4PPCh. 8.4 - Practice Problem 8.5 (solution page 797) Write a...Ch. 8.4 - Practice Problem 8.6 (solution page 797) Write a...Ch. 8.5 - Practice Problem 8.7 (solution page 798) Write a...Ch. 8.5 - Prob. 8.8PPCh. 8 - Prob. 8.9HWCh. 8 - In this chapter, we have introduced some functions...
Ch. 8 - How many hello output lines does this program...Ch. 8 - How many "hello' output lines does this program...Ch. 8 - Prob. 8.13HWCh. 8 - How many hello output lines does this program...Ch. 8 - How many "hello lines does this program print?Ch. 8 - Prob. 8.16HWCh. 8 - Prob. 8.17HWCh. 8 - Consider the following program: Determine which of...Ch. 8 - How many lines of output does the following...Ch. 8 - Use execve to write a program called my1s whose...Ch. 8 - What are the possible output sequences from the...Ch. 8 - Write your own version of the Unix system function...Ch. 8 - One of your colleagues is thinking of using...Ch. 8 - Modify the program in Figure 8.18 so that the...Ch. 8 - Write a version of the fgets function, called...
Knowledge Booster
Similar questions
- PROBLEM 21 - 0533: Write a BASIC program to solve Laplace's equation (a²V/Əx?) + (a²V/ay²) = 0. Consider a rectangular 8 by 8 grid %3D with boundary conditions such that 3 sides are assumed to be at a potential of 100. The remaining side is at zero potential as shown. Solution: We use the replacement of partial derivatives by central differencesarrow_forwardPLEASE WRİTE A PARAMETİC PROGRAM TO SOLVE !!! ( PYTHON)arrow_forward(7) Discrete structure: Please solve it on urgent basis: Question # 7: Show that at least ten of any 64 days chosen must fall on the same day of the week.arrow_forward
- 4. Look up the Pythagorean theorem if you are not already familiar with it. Use the following formula to solve for c in the formula: c = √a2 + b2. Use the proper functions from the cmath header file. Be sure to output the result..arrow_forwardIn matlab code Find the velocity of mars, earth, venus. In the descent phase of an extraterrestrial space mission, a spacecraft free falls through the planet's atmosphere. As it falls, it will reach a constant or terminal velocity when the air resistance force balances the gravitational attraction force. The terminal velocity is given by V₁ = where m is the spacecraft's mass [m], g is the acceleration due to gravity on the planet, p is the atmosphere's density [kg/m³], Cp is the spacecraft's drag coefficient, and A is the spacecraft's cross-sectional area [m²]. a) Write a function named terminalVelocity which calculates the terminal velocity an object. The function should . 2mg pCDA input m, g, p, CD, and A output the terminal velocity b) Write a program named q03.m which calculates the terminal velocity of a spacecraft at 10 km above the surface of various planets. The spacecraft's properties are m = 240 [kg], A= 15 [m²], and Cp = 0.5. The program should use the gravity and terminal…arrow_forward3. The diagram below shows the main land routes for vehicular traffic between points A and G in a city. The figures in the arcs represent the cost of traveling between each pair of nodes. a) Manually apply Dijkstra's algorithm to find the cheapest route between A and G (visited nodes and total distance). b) Formulate a linear programming problem in extended form, to determine the shortest route to travel from A to G. Do not use subscripts, name 14 variables, for example XFE would be the variable that indicates that the arc from F to E is used. c) If there is a fixed cost for visiting each node, modify the formulation of the problem to include said fixed cost in the objective function, and the variables and restrictions that are required. NODE A B C D E F G FIXED COST 25 18 32 20 28 18 34arrow_forward
- ENGINEERING • COMPUTER-ENGINEERING 2 - calculate the first four iterations for the approximate value of the root of the function f(x) = x*log(x) - 1 using the method below: a) Bissection Method. Use as interval for the root the values [2,3].arrow_forwardUse c programming to solve and explain clearlyarrow_forwardCode in python pleasearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
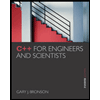
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr