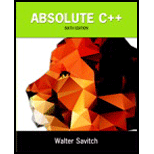
Concept explainers
Define a class named PrimeNumber that stores a prime number. The default constructor should set the prime number to l. Add another constructor that allows the caller to set the prime number. Also, add a function to get the prime number. Finally, overload the prefix and postfix ++ and -- operators so they return a Prime-Number object that is the next largest prime number (for ++) and the next smallest prime number (for For example, if the object’s prime number is set to 13, then invoking ++ should return a PrimeNumber object whose prime number is set to 17. Create an appropriate test program for the class.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Problem Solving with C++ (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Concepts of Programming Languages (11th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with Python (4th Edition)
- In number theory, a value can be categorized as a natural number (a whole number >0, often denoted ℕ), an integer (zero or a positive or negative whole number, including the natural numbers, often denoted ℤ), or a real number (which includes the natural numbers and integers, along with all other positive and negative numbers that are not integers, often denoted ℝ). a) write a definition for a number class that contains: (i) A single field suitable for storing either a natural number, or an integer, or a real number; (ii) Setter and getter methods for manipulating this field; (iii) A constructor that initializes new objects of number to have the value 1 (unity); (iv) a method that determines which kind of number is currently stored (returning 0 if the number is real and an integer and a natural number, 1 if the number is real and integer but not a natural number, and 2 if the number is real but neither an integer nor a natural number) Java code neededarrow_forward2019 AP® COMPUTER SCIENCE A FREE-RESPONSE QUESTIONS 2. This question involves the implementation of a fitness tracking system that is represented by the StepTracker class. A StepTracker object is created with a parameter that defines the minimum number of steps that must be taken for a day to be considered active. The StepTracker class provides a constructor and the following methods. addDailySteps, which accumulates information about steps, in readings taken once per day activeDays, which returns the number of active days averageSteps, which returns the average number of steps per day, calculated by dividing the total number of steps taken by the number of days tracked The following table contains a sample code execution sequence and the corresponding results. Statements and Expressions Value Returned Comment (blank if no value) StepTracker tr StepTracker(10000); Days with at least 10,000 steps are considered active. Assume that the parameter is positive. new tr.activeDays () ; No…arrow_forwardPPM (Portable Pixmap) use three integers to represent a pixel – this means we can have images with RGB colors. You will create a Pixel class in C++ which has three attributes: red: int green: int blue: int You will create a default constructor that initializes those values to 255, and an overloaded constructor that takes user input to assign the values. The class will also have the following functions: changeRGB (): Takes in three integers to update the red, green, and blue attributes. Returns nothing. printRGB (): Takes in nothing. Prints the red, green, and blue attributes in order with a single space in-between each value. Returns nothing. You will then recreate the art program from Assignment 5 with the following changes: Instead of a 2D array of integers, you will create a 2D array of Pixel object. Don’t be scared! This is similar to creating a 2D array of strings. You will prompt for three color values instead of one – red, green, and blue. These must be stored in a Pixel…arrow_forward
- The operator that is required to access members of classes and structs is called: a. Colon operator b. Scope resolution operator c. Operator overloading d. Member access operator e. None of the answers Answer: What is the name of the program that uses and interacts with the class? Answer: By assuming that all libraries are included, replace MYFUNCTION with the proper function name to make the code correct: 001010 111101101 01010101111110 1010101010101 111010101010 0101100 vector> MyVector; int rows= 10, cols = 4; MyVector.MYFUNCTION(rows, vector(cols, 100)); cout << MyVector [0] [1] << endl; for (int i } = 0; i < MyVector.size(); i++) { for (int j 0; j < MyVector[i].size(); j++) cout << MyVector[i][j] << " "; cout << endl;arrow_forwardWrite a Rectangle class that has the following fields: Length (L): a double - Width (W): a double The class should have the following functions: Constructor: accepts the length (L) and the width (W) of a rectangle as arguments. Constructor: does not take any parameters and initializes the length(L) and width(W) to zero. - A function called getArea which returns the area of the rectangle. A function called getPerimeter which returns the perimeter of the rectangle. {note: The area of a rectangle =L*W, and its perimeter = 2*(L+W) } Then, write a program to ask the user to enter the length and width of a rectangle, and then use the above class to calculate and print the area and perimeter of the rectangle.arrow_forwardUse Python Programming Language Write code that defines a class named Animal: Add an attribute for the animal name. Add an eat() method for Animal that prints ``Munch munch.'' A make_noise() method for Animal that prints ``Grrr says [animal name].'' Add a constructor for the Animal class that prints ``An animal has been born.'' A class named Cat: Make Animal the parent. A make_noise() method for Cat that prints ``Meow says [animal name].'' A constructor for Cat that prints ``A cat has been born.'' Modify the constructor so it calls the parent constructor as well. A class named Dog: Make Animal the parent. A make_noise() method for Dog that prints ``Bark says [animal name].'' A constructor for Dog that prints ``A dog has been born.'' Modify the constructor so it calls the parent constructor as well. Create a test program that will: Code that creates a cat, two dogs, and an animal. Sets the name for each animal. Code that calls eat() and make_noise() for each animal. (Don't…arrow_forward
- Write a class Runner having three private data members (distance, minutes, seconds) The class has A constructor which having no parameter – for setting values to zero or null.Getters/setters for data members.Write a function show() which displays the“The player covered “+distance+ “ in “ +minutes +” minutes , and “ + seconds+” seconds”; Write test Application that demonstrates the above class by calling all the methods, creating a Create 3 Runner objects, input values and then display the record of winner.in javaarrow_forwardWrite a code which contains a class CBookList with Private members: CBook books, int ListID. A constructor, which takes the above two arguments as input and assigns them to the class members. The constructor should also cater for default Getter and setter accessor functions for all PrintSome function which cout’s the details of the entire list of books in the particular PrintAll function which cout’s the details of the entire list of books in the entire A destructor. A function to add new nodes to the list, where each node is a CBook type object. Initial insertion should be done at the end of A sort function, that should be able to sort the entire list of books in ascending or descending order of their ID’s. Add and Delete functions to add lists and remove book DeleteAll function to remove all books starting from the head of thearrow_forward1- A complex number has the form a+bi , can be expressed as theordered pair of real numbers (a,b). The class represents the real andimaginary parts as double precision values.Provide a constructor that enables an object of this class to beinitialized when it is instantiated. The constructor should containdefault values.Provide Public member functions for each of the followingarithmetic’s functions (addition – subtraction – multiplication –division), a complex absolute value operation, printing the number inthe form (a,b), printing the real part , printing the imaginary part andfinal overload the == operator to allow comparisons of two complexnumbers.Include any additional operations that you think would be useful fora complex number class.Design, implement, and test your class.arrow_forward
- Write a class named as vehicle which has some attributes this class has three functions.i.e.fuelAmount ()loadingCapacity()applyBrakes()enginePower()Write a class named as Bus, Car and Truck which are inherited from class Vehicle. Also write the setter/getter, default and parameterized constructor in each class. Write a main which create object of derived class and call all inherited function of base class.Note: Each class should have at least two attribute of base class.arrow_forward18. If a class is declared as final, the incorrect statement is (). A. Indicates that this class is final B. Indicates that this class is a root class C. Methods in this class cannot be overridden D. Variables in this class cannot be hidden 19. In the following description of polymorphism, the incorrect one is (). A. Polymorphism refers to "one definition, multiple implementations" B. There are three types of polymorphism: dynamic polymorphism, static polymorphism, and default polymorphism C. Polymorphism is not used to speed up code D. Polymorphism is one of the core characteristics of OOP 20. In Java, two interfaces B and C have been defined. To define a class that implements these two interfaces, the correct statement is ( ). A. interface A extends B,C B. interface A implements B,C C. class A implements B,C D. class A extends B,C 21. Given the following java code, the correct description of the usage of "super" is (). class Student extends Person{ public Student() { super(); } 3 D…arrow_forwardSOLVE IN "C#" Write a program to create a class employee, it consist of ID, name, department and address. All employees belongs to “Computer Science” department and it can never be changed by any means. Employee ID is initialized only once when Employee object is created, any further attempt to change ID should be failed. Class must have a 3 parameterized constructor to set values and two methods: print(): to display all the data of a particular employee totalObjects(): to count and print total number of objects that has been created In Main(), create atleast two objects of employee class, display their records by calling print() function and also print the total number of objects that has been created. Sample Main Method: static void Main(string[] args) { Employee obj1 = new Employee(1, "AMMAD", "Karachi"); obj1.print(); Employee obj2 = new Employee(2, "ALI", "Islamabad"); obj2.print(); Employee.totalObjects(); }arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
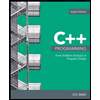
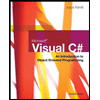