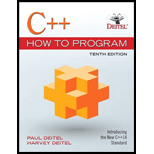
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 7.14E
Program Plan Intro
1.int a[10] used as a input array
2.
3. noduplicates function used to remove the duplicate elements in an array.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Create a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also move too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show the movements of poachers.
Create a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also moves too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show to movements
I have to develop an efficient parallel numerical integration program on a 2-D mesh but I'm struggling. And it has to be in Cstar
Chapter 7 Solutions
C++ How to Program (10th Edition)
Ch. 7 - Exercises 7.6(Fill in the Blanks) Fill in the...Ch. 7 - (True or False) Determine whether each of the...Ch. 7 - (Write C++ Statements) Write C++ statements to...Ch. 7 - (Two-Dimensional array Questions) Consider a...Ch. 7 - (Salesperson Salary Ranges) Use a one-dimensional...Ch. 7 - (One-Dimensional array Questions) Write statements...Ch. 7 - (Find the Errors) Find the error(s) in each of the...Ch. 7 - (Duplicate Elimination with array) Use a...Ch. 7 - Prob. 7.14ECh. 7 - Prob. 7.15E
Ch. 7 - (Dice Rolling) Write a program that simulates...Ch. 7 - ( What Does This Code Do?) What does the following...Ch. 7 - (Craps Game Modification) Modify the program of...Ch. 7 - (Converting vector Example of Section 7.10 to...Ch. 7 - Prob. 7.20ECh. 7 - (Sales Summary) Use a two-dimensional array to...Ch. 7 - (Knight's Tour) One of the more interesting...Ch. 7 - (Knight's Tour: Brute Forty Approaches ) In...Ch. 7 - (Eight Queens) Another puzzler for chess buffs is...Ch. 7 - (Eight Queens: Brute Force Approaches) In this...Ch. 7 - Prob. 7.26ECh. 7 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 7 - Prob. 7.28RECh. 7 - (Eight Queens) Modify the Eight Queens program you...Ch. 7 - (Print an array) Write a recursive function...Ch. 7 - Prob. 7.31RECh. 7 - Prob. 7.32RECh. 7 - (Maze Traversal) The grid of hashes (#) and dots...Ch. 7 - Prob. 7.34RECh. 7 - Making a Difference 7.35 (Polling) The Internet...
Knowledge Booster
Similar questions
- An employee is departing from the company you work for. Explain why it could be best practice not to delete their user account but to lock it instead.arrow_forwardthe nagle algorithm, built into most tcp implementations, requires the sender to hold a partial segment's worth of data (even if pushed) until either a full segment accumulates or the most recent outstanding ack arrives. (a) suppose the letters abcdefghi are sent, one per second, over a tcp connection with an rtt of 4.1 seconds. draw a timeline indicating when each packet is sent and what it contains.arrow_forwardJust need some assistance with number 3 please, in C#arrow_forward
- How do we find the possible final values of variable x in the following program. Int x=0; sem s1=1, s2 =0; CO P(s2); P(s1); x=x*2; V(s1); // P(s1); x=x*x; V(s1); // P(s1); x=x+3; V(s2); V(s1); Ocarrow_forwardLab 07: Java Graphics (Bonus lab) In this lab, we'll be practicing what we learned about GUIs, and Mouse events. You will need to implement the following: ➤ A GUI with a drawing panel. We can click in this panel, and you will capture those clicks as a Point (see java.awt.Point) in a PointCollection class (you need to build this). о The points need to be represented by circles. Below the drawing panel, you will need 5 buttons: о An input button to register your mouse to the drawing panel. ○ о о A show button to paint the points in your collection on the drawing panel. A button to shift all the points to the left by 50 pixels. The x position of the points is not allowed to go below zero. Another button to shift all the points to the right 50 pixels. The x position of the points cannot go further than the You can implement this GUI in any way you choose. I suggest using the BorderLayout for a panel containing the buttons, and a GridLayout to hold the drawing panel and button panels.…arrow_forwardIf a UDP datagram is sent from host A, port P to host B, port Q, but at host B there is no process listening to port Q, then B is to send back an ICMP Port Unreachable message to A. Like all ICMP messages, this is addressed to A as a whole, not to port P on A. (a) Give an example of when an application might want to receive such ICMP messages. (b) Find out what an application has to do, on the operating system of your choice, to receive such messages. (c) Why might it not be a good idea to send such messages directly back to the originating port P on A?arrow_forward
- Discuss how business intelligence and data visualization work together to help decision-makers and data users. Provide 2 specific use cases.arrow_forwardThis week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forward
- In completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
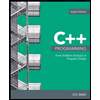
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
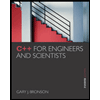
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
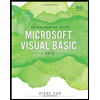
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
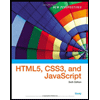
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
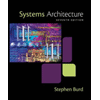
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
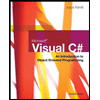
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,