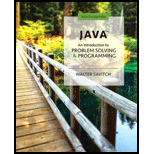
Concept explainers
Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Problem Solving with C++ (9th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
C How to Program (8th Edition)
Absolute Java (6th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- The local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license exam. The exam has 20 multiple choice questions. Here are the correct answers: B D A A C A B A C D B C D A D C C B D A A student must correctly answer 15 of the 20 questions to pass the exam. Write a class named DriverExam that holds the correct answers to the exam in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: • passed. Returns true if the student passed the exam, or false if the student failed • totalCorrect. Returns the total number of correctly answered questions • totalIncorrect. Returns the total number of incorrectly answered questions • questionsMissed. An int array containing the question numbers of the questions that the student missed Demonstrate the class in a complete program that asks the user to enter a student’s answers, and then displays the…arrow_forwardUse Java programming language Write a program that asks the user to enter 5 test grades (use an array to store them). Output the grades entered, the lowest and highest grade, the average grade, how many grades are above the average and how many are below and the letter grade for the average grade. Create a method that returns the lowest grade. Create a method that returns the highest grade. Create a method that returns the average grade. Create a method that returns how many grades were above the average. Create a method that returns how many grades were below the average. Create a method that returns the letter grade of the average (90-100 – A, 80-89 – B, 70-79 – C, < 70 – F)arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as atwo-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit…arrow_forward
- A positive integer greater than 1 is said to be prime if it has no divisors other than 1 and itself. Write a program that asks the user to input an integer greater than 1, then display all of the prime numbers that less than or equal to the number entered. The program should work as follows: • Once the user has entered a number, the program should display an array with all of the integers from 2 up through the value entered. • The program should then use a loop to step through the array. The loop should pass each element to a method that displays the element whether it is prime number. Your result should look like, for example: Enter an integer greater than 1: 9 2, 3, 4, 5, 6, 7, 8, 9 2 is prime 3 is prime 4 is not prime 5 is prime 6 is not prime 7 is prime 8 is not prime 9 is not prime Using java programmingarrow_forwardIn programming language Java, make a programm that scans an array of numbers and then checks which numbers have ONLY one digit 7. For example: we input numbers 23, 143, 98, 2373, 77, 97, 7001. And it displays these numbers: 2373, 97, 7001. (Doesn't display 77 because it has 2 sevens).arrow_forwardThis code is in java.You are walking along a hiking trail. On this hiking trail, there is elevation marker at every kilometer. The elevation information is represented in an array of integers. For example, if the elevation array is [100, 50, 20, 30, 50, 40], that means at kilometer 0, the elevation is 100 meters; at kilometer 1, the elevation is 50 meters; at kilometer 2, the elevation is 20 meters; at kilometer 3, the elevation is 30 meters; at kilometer 4, the elevation is 50 meters; at kilometer 5, the elevation is 40 meters. b) Write a method called longestUphill that returns the elevation change in meters of the longest uphill section. In the example above, the method should return 30 because the longest uphill section is from kilometer 2 with 20 meters to kilometer 4 with 50 meters. The elevation change is 50 - 20 = 30 meters. Note: A flat section with no elevation change is not considered part of an uphill.arrow_forward
- Write a program that displays the number of goals scored by players during the next World Cup in Brazil. • The program should prompt the user to enter the number of players they wish to display. If an invalid entry is inputNTED, the program should re-prompt for the number. • Create an array of strings to store the appropriate number of names as entered by the user • Create an array to store each players tally of goals The user is prompted to enter the name of the player and the player’s goal tally (re-prompt in case of an exception, and also if the player has a goal tally of less than zero) • These values are stored respectively into the array of names and goals for each player. • These details are then displayed on the screenarrow_forwardcomputer programmingarrow_forwardBlackjack is a card game where the goal is to reach a score of 21. Create a java version of this game with the following requirements. Extend the JPanel class and create an array of 52 cards. Add four sets of numbers from 1 to 10. Use J for Jack, Q for queen, K for king, and A for ace. Jack through king will have a value of 10, and the ace will have a value of 11. Deal two cards to the user and two cards to the computer. Do not show the value of the computer's first two cards to the user. Add buttons Hit, Stay, and Reset. If the user selects the "Hit" button, randomly select one of the cards from the array, and give it to the user. (After selecting a card, do not reuse this index during the rest of the game.) If the dealer has less than 21, "hit" the dealer, too. When the user selects the "Stay" button, add up the card values. The winner is the person who is closest to 21 without going over. Name the class Blackjack.java.arrow_forward
- 1. Write a program in a class EvensOddsAvg that counts the integers that are below the average. Read fifteen integers from the keyboard and place them in an array. Compute the sum and count of the evens and the odds separately. Find the average of the integers, and count and display the integers which are below average. Please Java Codearrow_forwardWrite a program that simulates rolling two dice. Prompt the user to enter the number of dice rolls. Use a loop to repeatedly call a method that simulates a dice roll and returns the total of the two dice to main. Keep track of the rolls in an array in main, and end the program by showing the results of the rolls.arrow_forwardCreate a class containing the main method. In the main method, create an integer array and initialize it with the numbers: 1,3,5,7,9,11,13,15,17,19 Pass the array as an argument to a method. Use a loop to add 1 to each element of the array and return the array to the main method. In the main method, use a loop to add the array elements and display the result. Note: In the loops, use the array field that holds the length of the array and do not use a number for array length.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
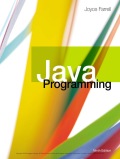
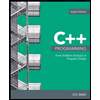