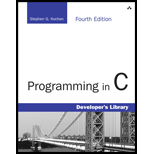
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 14E
7.10
Program Plan Intro
Modified version of the exercise 7.10 with the global variables:
Program Plan:
- Include required header files.
- Give the function prototype.
- Declare the variable “n” and “val” as global variable
- Define the main function,
- Get the number from the user.
- Call the function “prime()”.
- Check whether “val” equals to “1”.
- Print the corresponding statement.
- Otherwise, if the condition fails,
- Print the corresponding statement.
- Definition for the function “prime()”,
- Declare the variable “i”.
- Use for loop to check the condition.
- Check whether the remainder equals to 0.
- Return the value “0”.
- Return the value “1”.
- Check whether the remainder equals to 0.
- Use for loop to check the condition.
- Declare the variable “i”.
a)
Program Plan Intro
Modified version of the exercise 7.12(a) with the global variables:
Program Plan:
- Include required header files
- Declare and initialize the array values globally
- Declare the array “Arr_2” globally.
- Definition for the function “isTrans(int A[4][5], int B[5][4])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “4”
- For loop to check whether “j” is less than “5”
- Condition to check if both the elements in the array are not equal.
- Return the value “0”.
- Return the value “1”.
- Condition to check if both the elements in the array are not equal.
- Definition for the function “transposeMatrix(int A[4][5], int B[5][4])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “4”
- For loop to check whether “j” is less than “5”
- Condition to check if both the elements in the array are equal.
- Return the value “0”.
- Condition to check if both the elements in the array are equal.
- Check whether “isTrans(A,B)” equals to 1.
- For loop to check whether “k” is less than “5”
- For loop to check whether “l” is less than “4”
- Print the element.
- Print new line.
- For loop to check whether “l” is less than “4”
- Otherwise, print the corresponding statement inside “else” clause.
- For loop to check whether “k” is less than “5”
- Define the main function
- Call the function “transpose()” by passing the array.
- Return the value “0”.
b)
Program Plan Intro
Modified version of the exercise 7.12(b) with the global variables:
Program Plan:
- Include required header files
- Definition for the function “isTrans(int A[row][col], int B[row][col])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “row”
- For loop to check whether “j” is less than “col”
- Condition to check if both the elements in the array are not equal.
- Return the value “0”.
- Return the value “1”.
- Condition to check if both the elements in the array are not equal.
- Definition for the function “t(int A[row][col], int B[row][col])”
- Declare the variable “k” and “l”.
- For loop to check whether “k” is less than “row”
- For loop to check whether “j” is less than “col”
- Condition to check if both the elements in the array are equal.
- Return the value “0”.
- Condition to check if both the elements in the array are equal.
- Check whether “isTrans(A,B)” equals to 1.
- For loop to check whether “k” is less than “col”
- For loop to check whether “l” is less than “row”
- Print the element.
- Print new line.
- For loop to check whether “l” is less than “row”
- Otherwise, print the corresponding statement inside “else” clause.
- For loop to check whether “k” is less than “col”
- Define the main function
- Declare and initialize the array values.
- Declare the array “Arr_2”.
- Call the function “transpose()” by passing the array.
- Return the value “0”.
7.13
Program Plan Intro
Modified version of the exercise 7.13 with the global variables:
Program Plan:
- Include required header files.
- Declare and initialize the array as global.
- Declare the variable “n” as global variable.
- Definition for the function “sort()”.
- Declare the variables.
- Condition to check the “i<n-1”.
- Condition to check the “j<n”.
- Check whether to sort in increasing order.
- Assign “a[i] ” to “temp”.
- Assign “a[j] ” to “a[i]”.
- Assign “a[j]” to “temp”.
- Check whether to sort in decreasing order.
- Assign “a[i]” to “temp”.
- Assign “a[j] ” to “a[i]”.
- Assign “a[j]” to “temp”
- Check whether to sort in increasing order.
- Condition to check the “j<n”.
- Define the main function
- Print the statement.
- Loop to traverse the array.
- Print the element.
- Call the function “sort()”.
- Loop to traverse the array.
- Print the element.
- Print new line
- Return the value “0”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
reminder it an exercice not a grading work
GETTING STARTED
Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website.
Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”.
If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically.
With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet.
If cell B6 does not display your name, delete the file and download a new copy from the SAM website.
Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…
Need help completing this algorithm here in coding! 2
Whats wrong the pseudocode here??
Chapter 7 Solutions
Programming in C
Ch. 7 - Type in and run the 17 programs presented in this...Ch. 7 - Prob. 2ECh. 7 - Modify Program 7.8 so that the value of e is...Ch. 7 - Modify Program 7.8 so that the value of g is...Ch. 7 - Prob. 6ECh. 7 - Write a function that raises an integer to a...Ch. 7 - Prob. 8ECh. 7 - The least common multiple (1cm) of two positive...Ch. 7 - Prob. 10ECh. 7 - Write a function called a that takes two...
Knowledge Booster
Similar questions
- Help! how do I fix my python coding question for this? (my code also provided)arrow_forwardNeed help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forwardI'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
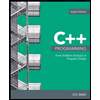
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
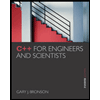
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
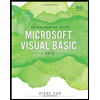
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
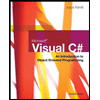
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
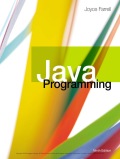
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage