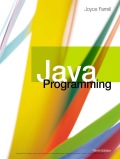
Explanation of Solution
Program:
File name: “QuizScoreStatistics.java”
//Import necessary header files
import java.util.Scanner;
//Define a class named QuizScoreStatistics
public class QuizScoreStatistics
{
//Define main method
public static void main(String[] args)
{
//Create an object for Scanner class
Scanner sc = new Scanner(System.in);
//Declare the variables
int count = 0;
int score = -1;
int min = Integer.MAX_VALUE, max = Integer.MIN_VALUE, sum = 0;
//Prompt the user to enter the scores
System.out.println("Enter the scores: (to stop enter 99) ");
//do..while loop
do
{
//Reads a string of digits
score = sc.nextInt();
/*If the user enters any number of student quiz scores other than 99*/
if (score != 99)
{
//If the score entered is less than 0 or more than 10
if (score < 0 || score > 10)
{
//Print the message
System.out.println("Score must be between 0 and 10");
}
//Else if the score lies between 0 and 10
else
{
//Increment the count
count++;
�...

Want to see the full answer?
Check out a sample textbook solution
- You were given the following negative array. write a program that converts each array element to its positive representation. Then add all these array elements and assign them to the dl register. .data myarr sbyte -5, -6, -7, -4.code ; Write the rest of the program and paste the fully working code in the space below. The dl register should have the value 22 after summing up all elements in the array. Your answer must be in 32-bit MSAM.arrow_forwardImplementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and finished goods inventory using a patchwork of spreadsheets and older software tools. These silos create inconsistent data, errors in stock levels, delivery delays, and customer dissatisfaction. Green Fields plans to implement an Integrated Inventory Management System to centralise production, procurement, inventory, and sales data to address these challenges. This technology aims to provide real-time visibility into stock levels, automate reorder points, and generate analytical dashboards for managers at both operational and strategic levels. Ultimately, the new system will streamline workflows, reduce…arrow_forward. Differentiate between continuous and discrete systems. How does their nature affect the selection of simulation techniques?arrow_forward
- hi, I need help to resolve the case, thank youarrow_forwardThe following table shows the timestamp and actions by two users. Choose the best option that describes the outcome of the actions. Time JohnSara 10:14 select* from hr.employees; 10:15 Update hr.employees set salary= 100 where employee_id= 206; 10:16 Commit: Select* from hr.employees; 10:18 Commit: 10:20 Select* from hr.employees; Commit: John's query willreturn the same results all three times it is executed as they are run in the same session. John's queries run at10:16 and10:20 produce the same result, which is different from the one at 10:14 John's query run at 10:16 waits until 10:18 to produce results, waiting for the commit to happen. John's queries run at 10:14 and 10:16 produce the same result, which is different from the one at 10:20arrow_forwardwhat's the process used to obtain IP configuration using DHCP in Windows Server.arrow_forward
- Consider the following sequential circuit: CLOCK a. Define the diagram circuit variables (5 pts) b. Derive the Flip-Flop input equations) (5 pts) c. Derive the circuit output equation (5 pts) d. Derive the state table of the circuit (5 pts) e. Derive the state diagram for this circuit (5 pts) Clk A D B B' CIK Question 2 (25 pts) A sequential circuit with two D flip-flops A and B, two inputs x and y, and one output z is specified by the following next-state and output equations: A(t + 1) = xy' + xB B(t + 1) = xA + xB' z = A a. Draw the logic diagram of the circuit. (5 pts) b. List the state table for the sequential circuit. (10 pts) c. Draw the corresponding state diagram. (10 pts)arrow_forward5. Word FrequencyWrite a program that reads the contents of a text file. The program should create a dictio-nary in which the keys are the individual words found in the file and the values are the number of times each word appears. For example, if the word “the” appears 128 times, the dictionary would contain an element with 'the' as the key and 128 as the value. The program should either display the frequency of each word or create a second file containing a list of each word and its frequency.arrow_forward3.) File Encryption and DecryptionWrite a program that uses a dictionary to assign “codes” to each letter of the alphabet. For example: codes = { ‘A’ : ‘%’, ‘a’ : ‘9’, ‘B’ : ‘@’, ‘b’ : ‘#’, etc . . .}Using this example, the letter A would be assigned the symbol %, the letter a would be assigned the number 9, the letter B would be assigned the symbol @, and so forth. The program should open a specified text file, read its contents, then use the dictionary to write an encrypted version of the file’s contents to a second file. Each character in the second file should contain the code for the corresponding character in the first file. Write a second program that opens an encrypted file and displays its decrypted contents on the screen.arrow_forward
- Returns an US standard formatted phone number, in the format of (xxx) xxx-xxxx the AreaCode, Prefix and number being each part in order. Testing Hint: We be exact on the format of the number when testing this method. Make sure you think about how to convert 33 to 033 or numbers like that when setting your string format. Reminder the %02d - requires the length to be 2, with 0 padding at the front if a single digit number is passed in.arrow_forwardThe next problem concerns the following C code: /copy input string x to buf */ void foo (char *x) { char buf [8]; strcpy((char *) buf, x); } void callfoo() { } foo("ZYXWVUTSRQPONMLKJIHGFEDCBA"); Here is the corresponding machine code on a Linux/x86 machine: 0000000000400530 : 400530: 48 83 ec 18 sub $0x18,%rsp 400534: 48 89 fe mov %rdi, %rsi 400537: 48 89 e7 mov %rsp,%rdi 40053a: e8 d1 fe ff ff 40053f: 48 83 c4 18 add callq 400410 $0x18,%rsp 400543: c3 retq 0000000000400544 : 400544: 48 83 ec 08 sub $0x8,%rsp 400548: bf 00 06 40 00 mov $0x400600,%edi 40054d: e8 de ff ff ff callq 400530 400552: 48 83 c4 08 add $0x8,%rsp 400556: c3 This problem tests your understanding of the program stack. Here are some notes to help you work the problem: • strcpy(char *dst, char *src) copies the string at address src (including the terminating '\0' character) to address dst. It does not check the size of the destination buffer. You will need to know the hex values of the following characters:arrow_forwardA ROP (Return-Oriented Programming) attack can be used to execute arbitrary instructions by chaining together small pieces of code called "gadgets." Your goal is to create a stack layout for a ROP attack that calls a function located at '0x4018bd3'. Below is the assembly code for the function 'getbuf', which allocates 8 bytes of stack space for a 'char' array. This array is then passed to the 'gets' function. Additionally, you are provided with five useful gadgets and their addresses. Use these gadgets to construct the stack layout. Assembly for getbuf 1 getbuf: 2 sub $8, %rsp 3 mov %rsp, %rdi 4 call gets 56 add $8, %rsp ret #Allocate 8 bytes for buffer #Load buffer address into %rdi #Call gets with buffer #Restore the stack pointer #Return to caller. Stack Layout (fill in Gadgets each 8-byte section) Address Gadget Address Value (8 bytes) 0x4006a7 pop %rdi; ret 0x7fffffffdfc0 Ox4006a9 pop %rsi; ret 0x7fffffffdfb8 0x4006ab pop %rax; ret 0x7fffffffdfb0 0x7fffffffdfa8 Ox4006ad mov %rax,…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
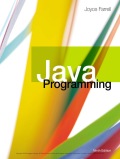
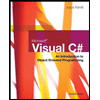
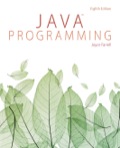