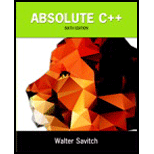
Define a class for a type called CounterType. An object of this type is used to count things, so it records a count that is a nonnegative whole number. Include a mutator function that sets the counter to a count given as an argument. Include member functions to increase the count by one and to decrease the count by one. Be sure that no member function allows the value of the counter to become negative. Also, include a member function that returns the current count value and one that outputs the count. Embed your class definition in a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Vector Mechanics for Engineers: Statics and Dynamics
- Define a class for a type called Counter . An object of this type is used to count things. Include a default constructor that sets the counter to zero and a constructor with one argument that sets the counter to the value specified by its argument. Write member functions to increase the value by one (called increment ) and decrease the value by one (called decrement ), don’t let the value go below 0. Write a member function ( print ) that prints out the value of the counter.*The first photo is the driver program that you should include to test your class ****The second is what the program should look like****arrow_forward18. If a class is declared as final, the incorrect statement is (). A. Indicates that this class is final B. Indicates that this class is a root class C. Methods in this class cannot be overridden D. Variables in this class cannot be hidden 19. In the following description of polymorphism, the incorrect one is (). A. Polymorphism refers to "one definition, multiple implementations" B. There are three types of polymorphism: dynamic polymorphism, static polymorphism, and default polymorphism C. Polymorphism is not used to speed up code D. Polymorphism is one of the core characteristics of OOP 20. In Java, two interfaces B and C have been defined. To define a class that implements these two interfaces, the correct statement is ( ). A. interface A extends B,C B. interface A implements B,C C. class A implements B,C D. class A extends B,C 21. Given the following java code, the correct description of the usage of "super" is (). class Student extends Person{ public Student() { super(); } 3 D…arrow_forwardWrite a full class definition for a class named Counter , and containing the following members: A data member counter of type int . A constructor that takes one int argument and assigns its value to counter . A function called increment that accepts no parameters and returns no value. increment adds one to the counter data member. A function called decrement that accepts no parameters and returns no value. decrement subtracts one to the counter data member. A function called getValue that accepts no parameters. It returns the value of the instance variable counter .arrow_forward
- Object passing checklist1. Go over each member function and add the const keyword after their declaration ifthey do not modify any of the class' member variables.2. When accepting objects as parameters, prefer to pass them by reference. Add reference declarator (& before the identifier name of an object parameter.3. If the member function does not modify the parameter, make the parameter constantby using the const keyword. apply the Object Passing Checklist. Modify food.h and volunteer.h to useobject references. Add const and & keywords in the appropriate places of the code.arrow_forward1- A complex number has the form a+bi , can be expressed as theordered pair of real numbers (a,b). The class represents the real andimaginary parts as double precision values.Provide a constructor that enables an object of this class to beinitialized when it is instantiated. The constructor should containdefault values.Provide Public member functions for each of the followingarithmetic’s functions (addition – subtraction – multiplication –division), a complex absolute value operation, printing the number inthe form (a,b), printing the real part , printing the imaginary part andfinal overload the == operator to allow comparisons of two complexnumbers.Include any additional operations that you think would be useful fora complex number class.Design, implement, and test your class.arrow_forwardC++ Friend function concepts Do not use operator overloading Create a class User with private member variables "id". In the class define a function increment_user_id() which will increment the id and return it as well. Create another method "void show_Id()" to show the id for a particular object. Create a constructor of User class where increment the Id by calling the increment_user_id() method for every new object . Finally create two objects of the class and print their corresponding id's on console.arrow_forward
- Object passing checklist1. Go over each member function and add the const keyword after their declaration ifthey do not modify any of the class' member variables.2. When accepting objects as parameters, prefer to pass them by reference. Add areference declarator (&) before the identifier name of an object parameter.3. If the member function does not modify the parameter, make the parameter constantby using the const keyword. apply the Object Passing Checklist. Modify food.h and volunteer.h to useobject references. Add const and & keywords in the appropriate places of the code.arrow_forwardDesign a class bookType that defines a book as a class. a. Each object of the class bookType will hold the following information about a book: title number of authors up to four authors publisher year published ISBN 13 (with dashes) price number of copies in stock (may be 0) b. Include the member functions to perform the various operations on objects of type bookType: Include individual get and set functions for all member variables. c. Add a member function to update the number of copies in stock. d. Add the appropriate constructors and a destructor (if one is needed). Ensure a default constructor is coded and the constructor initializes all data members. Remember, an array of classes uses a default constructor only. e. ALL member variables must be private and accessed through member functions. The main client program CANNOT access the variables directly. f. Ensure the class declaration and class implementation files are in separate header and code (.cpp) files. This assignment will…arrow_forwardIn the Member class, complete the function definition for SetNumPoints() that takes in the integer parameter newNumPoints. Ex: If the input is 4.5 62, then the output is: Height: 4.5 Number of points: 62 3 4 class Member { 5 6 7 8 9 10 11 12 17 } 1's public: void SetHeight (double newHeight); void SetNumPoints (int newNumPoints); 13 20 21 } double GetHeight() const; int GetNumPoints() const; private: 13 }; 14 15 void Member::SetHeight (double newHeight) { height= newHeight; 16 double height; int numPoints; ce es erabyCamScanner ur numPoints = newNumPoints;arrow_forward
- One use of the this pointer is to access a data member when there is a local variable with the same name. For example, if a member function has a local variable x and a data member x, then you can refer to the data member as this->x. Use this fact to complete the constructor implementation of this Point class: class Point { public: Point(int x, int y); int get_x() const; int get_y() const; private: int x; int y; }; Not all lines are useful.arrow_forwardPlease solution in c++arrow_forwardc++ please The Critter class is a base class with some basic functionality for running simulations of different kinds of critters. Critters can move, act, and remember their history. A typical simulation contains a number of critters of different types. In each step of the simulation, the act member function will be called on each critter. Define a class Sloth derived from Critter that simulates a sloth. Sloths alternate between eating and sleeping. Add the word "eat" or "sleep" to the history each time the act function is called. The implementation of the Critter class is not shown. Complete the following file: sloth_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A sloth eats and sleeps.*/class Sloth : public Critter{public:Sloth();. . .private:. . .}; Sloth::Sloth(){. . .} void Sloth::act(){if (...){add_history("eat");...}else{add_history("sleep");...}} int main(){Sloth sloth;sloth.act();cout << sloth.get_history() << endl;cout <<…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
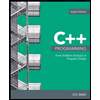
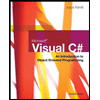
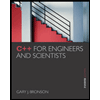