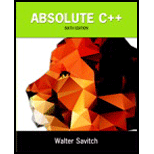
Concept explainers
Create a Temperature class that internally stores a temperature in degrees Kelvin. Create functions named setTempKe1vin, setTempFahrenheit, and setTempCe1sius that take an input temperature in the specified temperature scale, convert the temperature to Kelvin, and store that temperature in the class member variable. Also, create functions that return the stored temperature in degrees Kelvin, Fahrenheit, or Celsius. Write a main function to test your class. Use the equations shown next to convert between the three temperature scales:

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Absolute C++
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
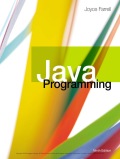
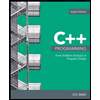
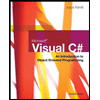
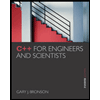
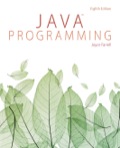