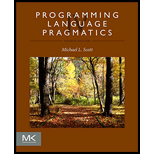
Explanation of Solution
C++ program for preorder iterator:
- The Example 6.69 explains how C++ use iterator () function different from Java.
- The program explains the use of pointers, references, inner classes and operator overloading.
- The tree node take the input of integer datatype.
//Defining the header files
#include <stack>
#include <iostream.h>
//Using namespace std
using namespace std;
//Defining the class tree_node
class tree_node
{
//Defining the left node variable
tree_node* left;
//Defining the right node variable
tree_node* right;
//Defining the public variable val
public:int val;
//Defining the constructor
tree_node(tree_node *l, tree_node *r, int v)
{
//assigning the value for left and right node
left = l; right = r; val = v;
}
//Defining another constructor with one parameter
tree_node(int v)
{
//Assigning value to tree node and val
left = 0; right = 0; val = v;
}
//Defining the inner class
class iterator
{
//Defining the variable with reference
stack<tree_node*> *s;
tree_node *cur;
//Defining the constructor for iterator as public
public:iterator(tree_node& n)
{
//Defining a stack to store the tree
s = new stack<tree_node*>();
//Assigning current value to n
cur = &n;
//Checking current position
if (cur)
{
//If current position in right side then push the element to the stack
if (cur->right) s->push(cur->right);
//If current position in left side then push the element to the stack
if (cur->left) s->push(cur->left);
}
}
//Destructor for iterator
~iterator()
{
//Deleting the stack
delete s;
}
//Checking current position with pointer
bool operator!=(void *p) const
{
//Return statement if current!=0
return (cur != 0);
}
//Use of reference
iterator& operator++()
{
//if condition for checking the stack is empty or not
if (s->empty())
{
//if stack is empty then it assign the current position to 0
cur = 0;
}
//If stack is not empty
else
{
//The current position value points to top of the stack
cur = s->top();
//use of pop() method
s->pop();
if (cur)
{
//Pushing the right element to stack
if (cur->right) s->push(cur->right);
//Pushing the right element to stack
if (cur->left) s->push(cur->left);
}
}
//returning the object
return *this;
}
//operator method using reference
int& operator*() const
{
//it return the current position as val
return cur->val;
}
//operator method pointers
int* operator->() const
{
//it return the current position as val
return &(cur->val);
}
};
//Defining the begin method of iterator which uses reference method
iterator& begin()
{
//Creating a new pointer i
iterator *i = new iterator(*this);
//Returing the pointer i
return *i;
}
//End method
void *end()
{
//which return 0
return 0;
}
};
Explanation:
- The program creates a class “tree_node” which is used to get the left and right nodes of the tree.
- The “tree_node” class used constructors to create the tree.
- Then the program creates an inner class with the name of “iterator”, which incorporates the reference, operator overloading, and pointers method.
- It creates a stack and pushing the elements to the stack based on current position of the variable.
Want to see more full solutions like this?
Chapter 6 Solutions
Programming Language Pragmatics, Fourth Edition
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- 1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forward
- using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardusing r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
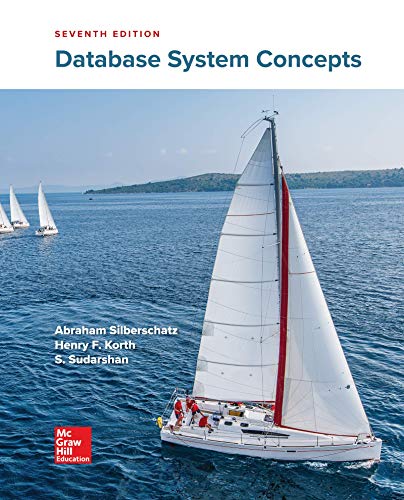
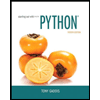
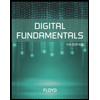
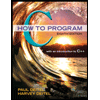
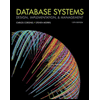
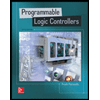