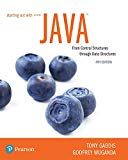
Deposit and Withdrawal Files
Use Notepad or another text editor to create a text file named Deposits.txt. The file should contain the following numbers, one per line:
100.00
124.00
78.92
37.55
Next, create a text file named Withdrawals.txt. The file should contain the following numbers, one per line:
29.88
110.00
27.52
50.00
12.90
The numbers in the Deposits.txt file are the amounts of deposits that were made to a savings account during the month, and the numbers in the Withdrawals.txt file are the amounts of withdrawals that were made during the month. Write a program that creates an instance of the SavingsAccount class that you wrote in

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Electric Circuits. (11th Edition)
Database Concepts (8th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- 1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forward
- using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r languagearrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
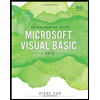
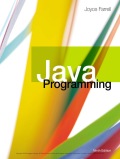
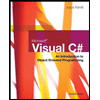