Java Programming
9th Edition
ISBN: 9780357238530
Author: Joyce Farrell
Publisher: Cengage Limited
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 8PE
Program Plan Intro
Automobile application
Program plan:
- In a file “Automobile.java”, create a class “Automobile”,
- Declare the necessary variables.
- Define the constructor,
- Call the method “set_Id()”,
- Call the method “set_Make()”,
- Call the method “set_Model()”,
- Call the method “set_Color()”,
- Call the method “set_Year()”,
- Call the method “set_Mpg()”,
- Define the method “set_Id()”,
- Initialize the final variable.
- Check whether either the id is less than “0” or greater than maximum id,
- If it is true, set the id to “0”.
- Otherwise, set the initial id.
- If it is true, set the id to “0”.
- Define the method “set_Make()” to set the initial make value.
- Define the method “set_Model()” to set the initial model value.
- Define the method “set_Color()” to set the initial color value.
- Define the method “set_Year(),
- Initialize the minimum year and maximum year.
- Check whether either the year is less than the minimum year or greater than the maximum year,
- If it is true, set the year to “0”.
- Otherwise, set the initial year.
- If it is true, set the year to “0”.
- Define the method “set_Mpg()”,
- Initialize the minimum and maximum miles per gallon.
- Check whether either the Miles Per Gallon(MPG) is less than the minimum mpg or greater than the maximum mpg,
- If it is true, set the MPG to “0”.
- Otherwise, set the mpg value.
- If it is true, set the MPG to “0”.
- Define the method “get_Id()” to return the id.
- Define the method “get_Make()” to return the Make.
- Define the method “get_Model()” to return the Model.
- Define the method “get_Color()” to return the Color.
- Define the method “get_Year()” to return the year.
- Define the method “get_Mpg()” to return the MPG.
- In a file “TestAutomobiles.java”, create a class “TestAutomobiles”,
- Define the method “main ()”,
- Call the constructor “Automobile()” that instantiates several job automobile dealers.
- Call the method “display1()” for first automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Make()” for first automobile dealer.
- Call the method “set_Model()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for second automobile dealer.
- Call the method “set_Color()” for second automobile dealer.
- Call the method “set_Year()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Define the method “display1()”,
- Print the output.
- Define the method “main ()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Now unmount the filesystem.
Create a sparse image file, called book.img, of the 2GiB hard drive.
Use a hexdump program to look at book.img and work out the offsets that store the contents of the
book youdownloaded. Record the offsets as a sequence of one or more ranges of hexadecimal
numbers. For example, in the following hexdump the text The rain in Spain falls mainly on the
plain. is stored at 0x10-0x1f, 0x30-0x4b (inclusive).
00000000: 0000 0000 0000 0000 0000 0000 0000 0000 .................00000010: 5468 6520 7261
696e 2069 6e20 5370 6169 The rain in Spai00000020: 0000 0000 0000 0000 0000 0000 0000
0000.................00000030: 6e20 6661 6c6c 7320 6d61 696e 6c79 206f n falls mainly 000000040:
6e20 7468 6520 706c 6169 6e2e 0000 0000 n the plain........
Compress the filesystem using gzip by running:
#gzip book.img
This should leave you with a file book.img.gz. You will use scp to transfer this file in a later task.
Enter your ranges in answers2.json as the answer for question1. To…
Task 4: Examine floppy.img
Mount floppy.img at a suitable mountpoint inside your VM.
Look inside the mountpoint directory. You should see a number of files in the top-level directory.
Look inside your answers2.json at the strings labelled contentA and contentB. Exactly one of those two strings
appears as the content of a file in the floppy disk's filesystem. I.e., there is a file that contains contentA or a file
that contains contentB but not both.
Additionally, the other string has been written into unused space of the floppy disk, so the data is in floppy.img
but cannot be seen inside any of the files at the mountpoint. However it can be seen in a hexdump of floppy.img.
I.e., if there is a file that contains contentA then contentB has been written into unused space.
You must search through your mountpoint directory to find out which of contentA and contentB is stored in a file
of the filesystem and which is stored in unused space. Suppose that contentA is stored in a file, and…
Download your personalized assignment files, answers2.json and floppy.img, into your Kali Linux Vm. Use
wget as before. For example, you'd use "wget
Chapter 5 Solutions
Java Programming
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- ▼ description: answer: question2: ▼ description: answer: "What are the offset ranges for the book file content within book.img?" "YOUR ANSWER GOES HERE" "What are the offset ranges for the content in unused space within floppy.img?" "YOUR ANSWER GOES HERE"arrow_forwardTask 3: Creating a Sample Hard Drive Image book.img Add a new (virtual) hard drive of capacity 2GiB to your Kali Linux VM. Create a FAT filesystem on the hard drive using mkfs. fat. Be careful to use your new 2GiB drive! It would be a good idea to use 1sblk to verify the block device name before proceeding. Mount the new hard drive at a mount point of your choice. Find a book from Project Gutenberg by an author whose last name begins with the same letter as your last name (look under "Browsing Options" and then "Authors" A-Z). Download the plain text UTF-8 and ensure it is saved into your mounted drive (it should be saved within the mount point directory). For example, using The Royal Guide to Wax Flower Modelling by Emma Peachey (a cracking read, no doubt) we can download the plain text file using:arrow_forward- Is FD A a partial or transitive dependency?- Is FD B a partial or transitive dependency?- Is FD C a partial or transitive dependency?- In what normal form is the relation Parking Tables?- Convert the relation Parking Tables to a set of 3NF relations.arrow_forward
- What happens to MAC addresses as frames travel from one node (device) to another? What happens to MAC addresses as frames travel from one network to another? What happens to IP addresses as packets travel from one node (device) to another? What happens to IP addresses as packets travel from one network to another?arrow_forwardCan you check if my explation is correct: Challenge: Assume that the assigned network addresses are correct. Can you deduce (guess) what the network subnet masks are? Explain while providing subnet mask bits for each subnet mask. [Hint: Look at the addresses in binary and consider the host ids] To assume that the network addresses are correct, we take a look at the network ID and IP addresses. Take 20.0.0.0/8, we have an /8 prefix which is Class A, that provide us with 255.0.0.0. This means that there are 24 host bits while the network bits are 8. When compared to the IP address 20.255.0.7, we can perform an additional operation with the subnet mask giving us 20.0.0.0. The same happened with 20.0.0.89, which we also got 20.0.0.0. Therefore, these two IP addresses fall within the respective /8 subnet. For 200.100.10.0/30, the /30 is Class C with a subnet mask of 255.255.255.252, meaning 30 network bits and only two host bits. When applying the subnet mask and the IP addresses…arrow_forwardIt is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forward
- It is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardIt is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardIt is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forward
- It is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardI need help: Challenge: Assume that the assigned network addresses are correct. Can you deduce (guess) what the network subnet masks are? Explain while providing subnet mask bits for each subnet mask. [Hint: Look at the addresses in binary and consider the host ids]arrow_forwardI would like to know if my answer statment is correct? My answer: The main difference is how routes are created and maintained across different networks. Static routing establishes router connections to different networks from the far left and far right. The Dynamic routing focus emphasizes immediate connection within the router while ignoring the other connections from different networks. Furthermore, the static routing uses the subnet mask to define networks such as 25.0.0.0/8, 129.60.0.0/16, and 200.100.10.0/30, which correspond to 255.0.0.0, 255.255.0.0, and 255.255.255.252. On the other hand, dynamic routing uses the wildcard mask to inverse the subnet mask, where network bits become 0 and host bits become 1, giving us 0.0.0.255, 0.0.255.255, and 0.0.0.3. Most importantly, the CLI commands used for Static and Dynamic routing are also different. For static routing, the “IP route” corresponds with the network, subnet mask, and next-hop IP address. In contrast, dynamic routing uses…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
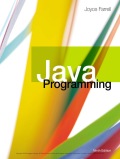
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
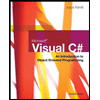
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
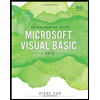
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
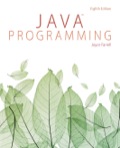
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY