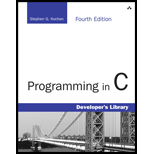
Program 5.10 has several inefficiencies. One inefficiency results from checking even numbers. Because it is obvious that any even number greater than 2 cannot be prime, the program could simply skip all even numbers as possible primes and as possible divisors. The inner f loop is also inefficient because the value of p is always divided by all values of d from 2 through p-1. This inefficiency could be avoided by adding a test for the value of i in the conditions of the f loop. In this manner, the f loop could be set up to continue as long as no divisor was found and the value of d was less than p. Modify Program 5.10 to incorporate these two changes. Then run the program to verify its operation. (Note: In Chapter 6, you discover even more efficient ways of generating prime numbers.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Programming in C
- 12. In .............., the bodies of the two loops are merged together to form a single loop provided that they do not make any references to each other. a. Loop unrolling b. Strength reduction c. Loop concatenation d. Loop jammingarrow_forwardFor Loop in Javaarrow_forwardThe program needs to be modified.arrow_forward
- The pseudocode for implementing the Taylor series expansion is shown below. The pseudocode is designed to return the value of the value of e* correct to two decimal places. This also requires the use of the factorial function created in the previous section. INPUT x, OUTPUT ExpVal % Initial computation n- 0, xold - 0 xnew - xold + (x^n)/nl error = |xnew - xold| xold = xnew n-1 %Successive computations WHILE error 2 0.001 xnew - xold + (x^n)/nl error |xnew - xold xold - xnew n=n+1 END WHILE ExpVal = xnew Question 10: Write a script taylor.m that computes the value of the function e* for a given user input x. Provide La proof that your script works.arrow_forwardConsider the following common task done repeatedly:• Read the next input (x = read())• If the input is not valid (valid(x)), exit the loop• Print the input read (print(x))• Repeat the loopUsing C++ syntax:Write the loop above using a pretest loop, posttest loop and a user-located loop control mechanism. From the three which one is more readable and why?arrow_forwardSelect the suitable answer:1.1 If a do...while structure is used:a. An infinite loop cannot take place.b. Counter-controlled iteration is not possible.c. The body of the loop will execute at least once.d. An off-by-one error cannot occur.arrow_forward
- Write a program in python that takes a sequence of non-negative numbers and performsthe computation suggested by the examples in the picture sent. A negative number is used toterminate the input but is not part of the sequence. USE ONLY WHILE LOOPS! DO NOT USE LANGUAGE OF: "true" or "break" when doing the code.arrow_forwardAssignment for Computer Architecture You are to write a program in MIPS that computes N! using a loop. Remember N! is the product of all the numbers from 1 to N inclusive, that is 1 x 2 x 3 x (N – 1) x N. It is defined as 1 for N = 0 and is undefined for values less than 0. The program first requests the user to input the value of N (display a prompt first so the user knows what to do). If the input value is less than 0, the program is to display “N! undefined for values less than 0” and request input again. If the value input is non-negative, it is to compute N! using a loop. You are to have your name, the assignment number, and a brief description of the program in comments at the top of your program. Since this is an assembly language program, I expect to see comments on almost every line of code in the program. Also make the code neat (line up the commands and comments in nice columns)arrow_forwardThe following function is designed to determine (the hard way) if a number is prime. What definition for stopNum will complete the function? There are multiple answers that will work in the sense that they will produce the right output. For full marks, your answer must be the one which will both work AND cause the loop to run the FEWEST number of times (thus making the function as fast as possible). def checkPrime(num): factors - [] stopNum = ??? for nn in range (2, stopNum): if num % nn == 0: factors.append (nn) if len(factors): print("The number is composite") else: print("The number is prime")arrow_forward
- Consider the following common task done repeatedly:• Read the next input (x = read())• If the input is not valid (valid(x)), exit the loop• Print the input read (print(x))• Repeat the loop Using C++ syntax:Write the loop above using a pretest loop, posttest loop and a user-located loop control mechanisms. Which one is more readable? Why?arrow_forwardSubject: Assembly Language Q: Write a program that displays a string in all possible combinations of foreground and background colors (16 x 16 =256). The colors are numbered from 0 to 15, so you can use a nested loop to generate all possible combinations. Also use a delay of 1s in each foreground color change.arrow_forward(Program) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters, such as zerocount, onecount, twocount, and so forth, to 0. Then generate a large number of pseudorandom integers between 0 and 9. Each time 0 occurs, increment zerocount; when 1 occurs, increment onecount; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of time they occurred.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
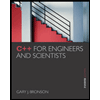
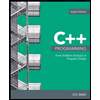