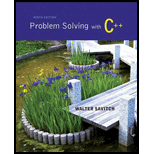
Concept explainers
Write a function that computes the average and standard deviation of four scores. The standard deviation is defined to be the square root of the average of the four values: (Si –a)2, where a is average of the four scores S1, S2, S3, and S4. The function will have six parameters and will call two other functions. Embed the function in a driver

Creation of program to compute average and standard deviation
Program Plan:
- Define the method “clc()” to calculate value of standard deviation.
- The variables are been declared initially.
- The difference of value with average is been computed.
- The square of resultant value is been computed.
- The final value is been returned.
- Define the method “stdMean()” to compute value of standard deviation as well as mean of values.
- The variables are been declared initially.
- The value of average is been calculated by summing values and dividing it by count of elements.
- Compute deviations for each value by calling “clc()” method.
- Compute overall value of deviation and return square root of resultant value.
- Define main method.
- Declare variables values of scores.
- Get each value from user.
- Store values in different variables.
- Call “stdMean()”to compute standard deviation as well as mean of values.
- Display standard deviation and mean of values.
Program Description:
The following C++ program describes about creation of program to compute mean as well as standard deviation of values entered by user.
Explanation of Solution
Program:
//Include libraries
#include <iostream>
#include <math.h>
//Define function prototypes
double stdMean(double,double,double,double,int, double&);
double clc(double, double);
//Use namespace
using namespace std;
//Define main method
int main()
{
//Declare variables
double s1,s2,s3,s4,avg,sd;
//Get value from user
cout<<"Enter 1st value ";
//Store value
cin>>s1;
//Get value from user
cout<<"Enter 2nd value ";
//Store value
cin>>s2;
//Get value from user
cout<<"Enter 3rd value ";
//Store value
cin>>s3;
//Get value from user
cout<<"Enter 4th value ";
//Store value
cin>>s4;
//Call method
sd=stdMean(s1,s2,s3,s4,4,avg);
//Display message
cout<<"The average is "<<avg<<"\n";
//Display message
cout<<"The standard deviation is "<<sd<<"\n";
//Pause console window
system("pause");
//Return
return 0;
}
//Define method clc()
double clc(double x,double avg)
{
//Declare variable
double tmp;
//Compute value
tmp=x-avg;
//Return value
return tmp*tmp;
}
//Define method stdMean()
double stdMean(double lX1, double lX2,double lX3, double lX4,
int n, double &avg)
{
//Declare variables
double d1,d2,d3,d4,dev;
//Compute value
avg=(lX1+lX2+lX3+lX4)/n;
//Call method
d1=clc(lX1,avg);
//Call method
d2=clc(lX2,avg);
//Call method
d3=clc(lX3,avg);
//Call method
d4=clc(lX4,avg);
//Compute value
dev=(d1+d2+d3+d4)/n;
//Return value
return sqrt(dev);
}
Enter 1st value 8
Enter 2nd value 3
Enter 3rd value 5
Enter 4th value 4
The average is 5
The standard deviation is 1.87083
Press any key to continue . . .
Want to see more full solutions like this?
Chapter 5 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Starting Out With Visual Basic (7th Edition)
- Using python programming a screenshot will be helpful #2arrow_forwardWrite a program that calculates the average of a group of test scores. You will ask the user to input each test score individually. When calculating the average, the lowest score in the group is dropped. Your program should use the following functions: void getScore() should ask the user for a test score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five scores to be entered. void calcAverage() should calculate and display the average of the four highest scores. This function should be called just once by main and should be passed the five scores. int findLowest) should find and return the lowest of the five scores passed to it. It should be called by calcAverage(), which uses the function to determine which of the five scores to drop. Void displayAverage() should display the average of the four highest scores. This function should be called by calcAverage(). Input Validation: Do not accept test scores lower…arrow_forwardIt is estimated that a restaurant's tip box accumulates twice as much as the previous day. Write the function with the main () function that takes the number of days as a parameter and returns the total tip amount on the day entered in the restaurant with a 10-TL tip on the first day. Sample Work: Enter the number of days: 4 --------------------------------- Total estimated stake in the box: 150.00 -TL in carrow_forward
- If a year has the digit 7 OR the digit 4 in it, then it is called a “Beautiful year”. For example, 2017, 2014, and 2072 are all beautiful years. If the year has BOTH 4 and 7 in it, then it's an “Amazing year”. For example, the years 2047 and 2074 are amazing years. All other years are “Ugly years”. You need to write a function that takes a year as an argument and return it's type. Then, finally print the returned values in the function call. ================================================ Sample Function Call 1: function_name(2470) Sample Output 1: Amazing year ================================================ Sample Function Call 2: function_name(2170) Sample Output 2: Beautiful year ================================================ Sample Function Call 3: function_name(2021) Sample Output 3: Ugly yeararrow_forwardLewis' invention can determine if the weather is too hot (104°F and above) or too cold (50°F and below) once the unit of measure of the temperature is in Fahrenheit. Which is why, he added another function that converts the temperature from Celsius to Fahrenheit to make his invention work. Create a program that contains the function: HotOrCold(). HotOrCold() function will accept as parameter a temperature type and the temperature and will do the following: Convert the given temperature in Celsius to Fahrenheit. a. [F = C + 32] a. Display if depending on the temperature value. Weather is too hot" or "Weather is too cold" , OF THE MADE4Learners NSTITUTE FRAMEWORK VERSITY UNI MULTIPLE APPROACHES TO DISTANCE EDUCATION FOR TECHNOLOGIAN LEARNERS : O Google Slides CHNOLOGarrow_forwardIf a value for a parameter is not passed when the function is called, the default given value is used, but if a value is specified, this default value is ignored and the passed value is used instead. Write a function called sum, that calculates the total value of a and b, where a and b are integers, a= 100 and b = 200 in the main function. Your main function will declare your variables, call the function to add values and then call the function again. When you compile your program it has to give you total result of sum (a, b) from the main function, and the result for sum (a,b) from the function sum. There is a default value of b which is part of the function list, with the value 20. Your function sum, will calculate the result of a and b, and then return the result.arrow_forward
- Write a function in python code for checking the speed of drivers. This function should have one parameter: speed. If speed is less than 70, it should print “Ok”. Otherwise, for every 5km above the speed limit (70), it should give the driver one demerit point and print the total number of demerit points. For example, if the speed is 80, it should print: “Points: 2”. If the driver gets more than 12 points, the function should print: “License suspended”.arrow_forwardDo it with Programmingarrow_forwardChange this question to work using a function. decide what the name of the function of each should be, how many parameters are required and what value needs to be returned. You're no longer required to solve the problem - try to re-manage your code to be a function. Write a program that uses input to prompt a user for their name and then welcomes them. Enter your name: Chuck Hello Chuckarrow_forward
- Use Python You are planning to add bookcases to a wall in your living room, but you don't know how many bookcases to buy. You decide to write a Python function, get_num_bookcases, to help. Your function needs to do the following: Take parameters for the width of the wall (an integer number of feet) and the width of a bookcase (an integer number of inches). (Your function does not read input from the keyboard; it gets its input through named parameters.) Calculate how many whole bookcases will fit on the wall using modular arithmetic (use div and/or mod, represented by the operators // and %, as needed to solve the problem). Return the number of bookcases. For example: Test Result print(get_num_bookcases(20, 50)) 4 print(get_num_bookcases(8, 15)) 6 print(get_num_bookcases(3, 40)) 0arrow_forwardOver the years, people have created a few nicknames for me. One that sticks without me telling me to call me that name is "Denck." It works great with anyone whose name ends with an "er". We are going to create a function called "nickName" which takes in one parameter. This parameter stores the name you want to create the nickname for. If the name ends with an er, return the name with the er removed. If the name does not have an er then return the full name back to the program.arrow_forwardWhich of the following statements about testing are always true. A. If a test fails, there must necessarily be a defect in the executed code. B. If a test passes, there cannot be a defect in any code executed by the test, but there could be a defect elsewhere in the function. C. If a test is unsound, that test passing or failing doesn't tell us whether or not a defect exists. D. All of the above are truearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
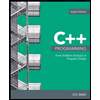
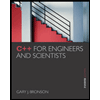