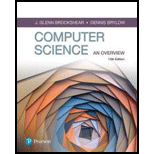

Explanation of Solution
Example of algorithm:
The algorithm is unceremoniously defined as the set of statement or steps being performed to achieve a desired result. Figure 5.1 states: “An algorithm is an ordered set of unambiguous, executable steps that defines a terminating process”.
Take the example of the process for making tea.
- Pour water in vessel.
- Add tea leaves in the vessel.
- Put the vessel to boil.
The above set of statements conform an informal statement that describes the steps to complete a particular task. But these statements have no relation or don’t conform to the formal definition of the algorithm. The above statements are not conforming because there is ambiguity in each step, which means there is no perfect ending point for the particular set of statements.
Want to see more full solutions like this?
Chapter 5 Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Create a BankAccount Test class that contains a main() method that instantiates an object of type BankAccount, with account number of 12345, account holder name of "John Doe", initial balance of $1000 and account type as SAVINGS . Then use the deposit() and withdraw() methods of the object to deposit $500 and withdraw $300. Finally, use the getAccountInfo() method to print the current account information. Use the getAccountInfo() method to verify that the deposit and withdrawal actions are performed correctly and that the account information is updated accordingly.arrow_forwardAdd a new class checkingAccount that inherits from the BankAccount class, and has a double instance variable overdraft Limit in addition to the variables inherited from the superclass. • Create a constructor for the checking Account class that takes in the account number, account holder name, initial balance, account type and overdraft limit as input, and uses the super keyword to call the constructor of the superclass, passing in the account number, account holder name and initial balance, account type. • Re-write the withdraw() method in the checkingAccount class so that it first checks if the withdrawal amount is less than the current balance plus the overdraft limit. If it is, the withdrawal is allowed and the balance is updated. If not, the method should return an error message "Insufficient funds". • Create a new method displayOverdraft Limit() that returns the overdraft limit of the CheckingAccount . • In the BankAccountTest class, create a new object of type Checking Account…arrow_forwardExplain what the rwpos() function does. What is the base case? What values are passed to the recursive call? What value is returned by the original function call?arrow_forward
- Explain what the rs() function does. What value(s) does it return? Is that value always the same? Why or why not?arrow_forwardExplain what the rwsteps() function does. What is the base case? What values are passed to the recursive call? What is printed each time rwsteps() is called? What value is returned by the original function call?arrow_forwardmodule: java Question3: (30 MARKS) Passenger Rail Agency for South Africa Train Scheduling System Problem Statement Design and implement a train scheduling system for Prasa railway network. The system should handle the following functionalities: 1. Scheduling trains: Allow the addition of train schedules, ensuring that no two trains use the same platform at the same time at any station. 2. Dynamic updates: Enable adding new train schedules and canceling existing ones. 3. Real-time simulation: Use multithreading to simulate the operation of trains (e.g., arriving, departing). 4. Data management: Use ArrayList to manage train schedules and platform assignments. Requirements 1. Add Train Schedule, Cancel Scheduled Train, View Train Schedules and Platform Management 2. Concurrency Handling with Multithreading i.e Use threads to simulate train operations, Each…arrow_forward
- java: Question 1: (40 MARKS) E-Hailing Bicycle Management System Case Study:An e-hailing company that rents out bicycles needs a system to manage its bicycles, users, and borrowing process. Each user can borrow up to 2 bicycles at a time, specifically for families with children 18 years or below. The system must track the bicycles (name, make, type, and availability) and users (name, ID, and borrowed bicycles). The company also wants to ensure that the system uses a multidimensional array to store information about the bicycles. Requirements: Add and View Bicycles: Borrow Bicycles: Return Bicycles Display Borrowed Bicycles and Search for a bicycle Create a menu-driven program to implement the above. Sample Output: Add Bicycle View All Bicycles Borrow Bicycle Return Bicycle View Borrowed Bicycles Search Bicycle ExitEnter your choice: Question 2 (30 MARKS) Pentagonal Numbers Problem Statement Create a Java program that will display the first 40 pentagonal…arrow_forwardRequest for Java Programming Expert Assistance - Module: Java 731 Please assign this to a human expert for detailed Java programming solutions. The AI keeps attempting to answer it, but I need expert-level implementation. Question 1 (40 MARKS) - E-Hailing Bicycle Management System Case Study:An e-hailing company needs a Java system to manage bicycle rentals. Key requirements: Users (families with children ≤18) can borrow up to 2 bicycles. Track bicycles (name, make, type, availability) and users (name, ID, borrowed bikes). Use a multidimensional array for bicycle data. Functionalities: Add/view bicycles. Borrow/return bicycles. Display borrowed bikes and search functionality. Menu-driven program with the following options: Copy 1. Add Bicycle 2. View All Bicycles 3. Borrow Bicycle 4. Return Bicycle 5. View Borrowed Bicycles 6. Search Bicycle 7. Exit Question 2 (30 MARKS) - Pentagonal Numbers Problem Statement:Write a Java program to display the first 40…arrow_forwardmodule , java 731 Question 1: (40 MARKS) E-Hailing Bicycle Management System Case Study:An e-hailing company that rents out bicycles needs a system to manage its bicycles, users, and borrowing process. Each user can borrow up to 2 bicycles at a time, specifically for families with children 18 years or below. The system must track the bicycles (name, make, type, and availability) and users (name, ID, and borrowed bicycles). The company also wants to ensure that the system uses a multidimensional array to store information about the bicycles. Requirements: Add and View Bicycles: Borrow Bicycles: Return Bicycles Display Borrowed Bicycles and Search for a bicycle Create a menu-driven program to implement the above. Sample Output: Add Bicycle View All Bicycles Borrow Bicycle Return Bicycle View Borrowed Bicycles Search Bicycle ExitEnter your choice:arrow_forward
- this module is java 371. please answer all questions correctly , include all comments etc and follow all requirements. Question 1: (40 MARKS) E-Hailing Bicycle Management System Case Study:An e-hailing company that rents out bicycles needs a system to manage its bicycles, users, and borrowing process. Each user can borrow up to 2 bicycles at a time, specifically for families with children 18 years or below. The system must track the bicycles (name, make, type, and availability) and users (name, ID, and borrowed bicycles). The company also wants to ensure that the system uses a multidimensional array to store information about the bicycles. Requirements: Add and View Bicycles: Borrow Bicycles: Return Bicycles Display Borrowed Bicycles and Search for a bicycle Create a menu-driven program to implement the above. Sample Output: Add Bicycle View All Bicycles Borrow Bicycle Return Bicycle View Borrowed Bicycles Search Bicycle ExitEnter your choice: Question 2…arrow_forwardthis module is java 371. please answer all questions correctly , include all comments etc and follow all requirements. Question 1: (40 MARKS) E-Hailing Bicycle Management System Case Study:An e-hailing company that rents out bicycles needs a system to manage its bicycles, users, and borrowing process. Each user can borrow up to 2 bicycles at a time, specifically for families with children 18 years or below. The system must track the bicycles (name, make, type, and availability) and users (name, ID, and borrowed bicycles). The company also wants to ensure that the system uses a multidimensional array to store information about the bicycles. Requirements: Add and View Bicycles: Borrow Bicycles: Return Bicycles Display Borrowed Bicycles and Search for a bicycle Create a menu-driven program to implement the above. Sample Output: Add Bicycle View All Bicycles Borrow Bicycle Return Bicycle View Borrowed Bicycles Search Bicycle ExitEnter your choice: Question 2…arrow_forwardthis module is java 371. please answer all questions correctly , include all comments etc and follow all requirements. Question 1: (40 MARKS) E-Hailing Bicycle Management System Case Study:An e-hailing company that rents out bicycles needs a system to manage its bicycles, users, and borrowing process. Each user can borrow up to 2 bicycles at a time, specifically for families with children 18 years or below. The system must track the bicycles (name, make, type, and availability) and users (name, ID, and borrowed bicycles). The company also wants to ensure that the system uses a multidimensional array to store information about the bicycles. Requirements: Add and View Bicycles: Borrow Bicycles: Return Bicycles Display Borrowed Bicycles and Search for a bicycle Create a menu-driven program to implement the above. Sample Output: Add Bicycle View All Bicycles Borrow Bicycle Return Bicycle View Borrowed Bicycles Search Bicycle ExitEnter your choice: Question 2…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningPrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
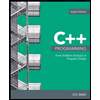
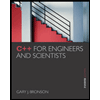
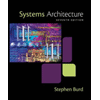
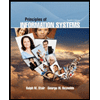
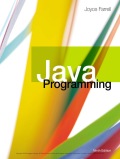