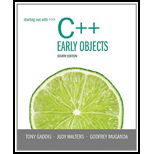
Concept explainers
True or false: The following if / else statements cause the same output to display.
A) if (x > y)
cout << "x is greater than y. \n";
else
cout << "x is not greater than y. \ n" ;
B) if (x <= y)
cout << "x is not greater than y. \n";
else
cout << "x is greater than y\n";

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out with C++: Early Objects
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- (Misc. application) a. Write a program to reverse the digits of a positive integer number. For example, if the number 8735 is entered, the number displayed should be 5378. (Hint: Use a do statement and continuously strip off and display the number’s units digit. If the variable numinitially contains the number entered, the units digit is obtained as (num % 10). After a units digit is displayed, dividing the number by 10 sets up the number for the next iteration. Therefore, (873510)is5and(8735/10)is873. The do statement should continue as long as the remaining number is not 0.) b. Run the program written in Exercise 3a and verify the program by using appropriate test data.arrow_forwardWrite aprogram using nestedif statements that perform the following test: If the variable employed is equal to 'Y' and if worklength is equal or greater than 5, then display the message "Your credit card application is accepted". However, if worklength is less than 5, then display the message "Please provide a guarantor". Otherwise, if the variable employed is equal to 'N', then display the message "Your credit card application is rejected". C.arrow_forwardQUESTION 1 PROBLEM: Write a program that prompts the user to input the amount of money that an ATM machine will dispense and then show the breakdown of how many 1000, 500, and 100 peso bill's are dispensed. The program must display the amount then prompt the user if the transaction will continue or not by choosing between Y (for Yes) and N (for No). If an invalid character is entered, it must display that the user entered an invalid character. The program must also display that the maximum allowable amount is 10,000 and the minimum allowable amount is 100. If the entered amount is greater than 10,000 or less than 100, an error notification must be displayed. Moreover, the entered amount must be exactly divisible by 100. SPECIFICATIONS: - You may only use the codes that we studied under our lectures All lines of codes should have a comment. - Save your cpp file as Surname - PE (Example: Pangaliman - PE.cpp) TEST CASES: If a user inputs an amount less than or equal to 10,000: REMINDER:…arrow_forward
- The monthly payment on a loan may be calculated by the following formula: N Rate * (1 + Rate)* * L/; Payment (1 + Rate)" - 1 Rate is the monthly interest rate, which is the annual interest rate divided by 12. (A 12 percent annual interest would be 1 percent monthly interest.) N is the number of payments and L is the amount of the loan. Write a program that asks for these values and displays a report similar to the following: $ 10000.00 Loan Amount: Monthly Interest Rate: Number of Payments: Monthly Payment: Amount Paid Back: 18 36 $ $ 11957.15 $ 1957.15 332.14 Interest Paid:arrow_forward35. Evaluate: 1+6==7 || 3+2==1. Write T for true and F for false ( ||-or)arrow_forwardQuestion: Write program that asks the user to enter car information for 3 cars. The user will read the car plate number, driven mileage and number of months since last service. The program should display the message ‘Car is due for service’ if one of the following is true: The car drove more than 5000 KM The program should also count and display the number of cars due for service. Input/Output example: Enter car plate number: 600600 Enter the mileage and number of months since last service: 5230 Car is due for service Enter car plate number: 600666 Enter the mileage and number of months since last service: 5002 Car is due for service Enter car plate number: 600222 Enter the mileage and number of months since last service: 1500 The number of cars due is: 2arrow_forward
- Computer Fundamentals and Programming 2 Write a program that determines a student’s grade. The program will accept 3 scores and computes the average score. Determine the grade based on the following rules: - If the average score is equal or greater than 90, the grade is A. - If the average score is greater than or equal to 70 and less than 90, the grade is B. - If the average score is greater than or equal to 50 and less than 70, the grade is C. - If the average score is less than 50, the grade is F. Source Codes and Print Screen of the Outputarrow_forwardif (x y)Z =5*x*x + 3* x / y; else Z =y*y - == 3*x; truecout 20);{Cout>>i; A program to print only 20 integer number * true Falsearrow_forwardPROBLEM: Write a program that prompts the user to input the amount of money that an ATM machine will dispense and then show the breakdown of how many 1000, 500, and 100 peso bill/s are dispensed. The program must display the amount then prompt the user if the transaction will continue or not by choosing between Y (for Yes) and N (for No). If an invalid character is entered, it must display that the user entered an invalid character. The program must also display that the maximum allowable amount is 10,000 and the minimum allowable amount is 100. If the entered amount is greater than 10,000 or less than 100, an error notification must be displayed. Moreover, the entered amount must be exactly divisible by 100. SPECIFICATIONS: - You may only use the codes that we studied under our lectures All lines of codes should have a comment. - Save your cpp file as Surname - PE (Example: Pangaliman - PE.cpp) TEST CASES: If a user inputs an amount less than or equal to 10,000: REMINDER: Maximum…arrow_forward
- PROBLEM:Write a program that prompts the user to input the amount of money that an ATM machine will dispense and then show the breakdown of how many 1000, 500, and 100 peso bill/s are dispensed. The program must display the amount then prompt the user if the transaction will continue or not by choosing between Y (for Yes) and N (for No). If an invalid character is entered, it must display that the user entered an invalid character. The program must also display that the maximum allowable amount is 10,000 and the minimum allowable amount is 100. If the entered amount is greater than 10,000 or less than 100, an error notification must be displayed. Moreover, the entered amount must be exactly divisible by 100. TEST CASES: If a user inputs an amount less than or equal to 10,000: REMINDER: Maximum amount must only be 10,000 and the minimum amount must not be less than 100 Enter Amount to dispense: 4900The Amount of money you entered is 4900.Do you want to continue your transaction?Enter…arrow_forwardQ3: By using Select-Case; write a program to calculate (BMI body mass index) (11 M) weight BMI = hieght And print the result as follows Underweight = 30arrow_forward3.Professor Mysterious uses a special formula to calculate the grade of his students. Write a program that repeatedly does the following: a. Prompt the user to enter the grade of the first test b. Prompt the user to enter the grade of the second test . Prompt the user to enter the grade of the third test. The program should then calculate and displays the student grade based on the following formula: grade-.25*testi+.61 test2+.14 test3 NOTE: Exit the program using sentinel or magic value. SAMPLE PROGRAM RUN Enter Test1 (0 to exit): 100 Enter Test2:90 Enter Test3:87 Grade: 92.08 Enter Test1 (0 to exit): 0 Thank you for using this program.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
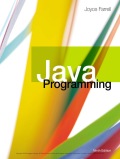
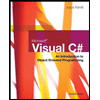
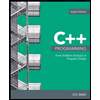
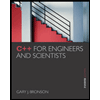