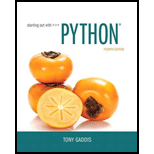
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 4, Problem 9MC
Program Description Answer
The abbreviated form of GIGO is “garbage in, garbage out”.
Hence, the correct answer is option “B”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.
Southern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not).
The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15.
A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots):
The average daily utilization of the aircraft (percentage of total flights that fly)
The…
Why is JAVA OOP is really difficult to study?
Chapter 4 Solutions
Starting Out with Python (4th Edition)
Ch. 4.1 - What is a repetition structure?Ch. 4.1 - What is a condition-controlled loop?Ch. 4.1 - What is a count-controlled loop?Ch. 4.2 - What is a loop iteration?Ch. 4.2 - Does the while loop test its condition before or...Ch. 4.2 - How many times will 'Hello world' be printed in...Ch. 4.2 - What is an infinite loop?Ch. 4.3 - Rewrite the following code so it calls the range...Ch. 4.3 - What will the following code display? For number...Ch. 4.3 - What will the following code display? for number...
Ch. 4.3 - What will the following code display? for number...Ch. 4.3 - What will the following code display? for number...Ch. 4.4 - Prob. 13CPCh. 4.4 - Should an accumulator be initialized to any...Ch. 4.4 - What will the following code display? total - 0...Ch. 4.4 - What will the following code display? number 1 =...Ch. 4.4 - Rewrite the following statements using augmented...Ch. 4.5 - Prob. 18CPCh. 4.5 - Why should you take care to choose a distinctive...Ch. 4.6 - What does the phrase garbage in, garbage out mean?Ch. 4.6 - Give a general description of the input validation...Ch. 4.6 - Describe the steps that are generally taken when...Ch. 4.6 - Prob. 23CPCh. 4.6 - If the input that is read by the priming read is...Ch. 4 - A_________-controlled loop uses a true/false...Ch. 4 - A _____-controlled loop repeats a specific number...Ch. 4 - Each repetition of a loop is known as a(n) a cycle...Ch. 4 - The while loop is a _______ type of loop. a....Ch. 4 - A(n) ______ loop has no way of ending and repeats...Ch. 4 - The -= operator is an example of a(n) _________...Ch. 4 - Prob. 7MCCh. 4 - A(n) ____________ is a special value that signals...Ch. 4 - Prob. 9MCCh. 4 - The integrity of a programs output is only as good...Ch. 4 - The input operation that appears just before a...Ch. 4 - Validation loops are also known as _________. a....Ch. 4 - A condition-controlled loop always repeats a...Ch. 4 - The while loop is a pretest loop.Ch. 4 - The following statement subtracts 1 from x: x = x ...Ch. 4 - It is not necessary to initialize accumulator...Ch. 4 - In a nested loop, the inner loop goes through all...Ch. 4 - To calculate the total number of iterations of a...Ch. 4 - The process of input validation works as follows:...Ch. 4 - What is a condition-controlled loop?Ch. 4 - What is a count-controlled loop?Ch. 4 - What is an infinite loop? Write the code for an...Ch. 4 - Why is it critical that accumulator variables are...Ch. 4 - What is the advantage of using a sentinel?Ch. 4 - Prob. 6SACh. 4 - What does the phrase garbage in, garbage out mean?Ch. 4 - Give a general description of the input validation...Ch. 4 - Write a while loop that lets the user enter a...Ch. 4 - Write a while loop that asks the user to enter two...Ch. 4 - Write a for loop that displays the following set...Ch. 4 - Write a loop that asks the user to enter a number....Ch. 4 - Write a loop that calculates the total of the...Ch. 4 - Rewrite the following statements using augmented...Ch. 4 - Write a set of nested loops that display 10 rows...Ch. 4 - Write code that prompts the user to enter a...Ch. 4 - Write code that prompts the user to enter a number...Ch. 4 - Bug Collector The Bug Collector Problem A bug...Ch. 4 - Calories Burned Running on a particular treadmill...Ch. 4 - Budget Analysis Write a program that asks the user...Ch. 4 - Distance Traveled The distance a vehicle travels...Ch. 4 - Average Rainfall Write a program that uses nested...Ch. 4 - Celsius to Fahrenheit Table Write a program that...Ch. 4 - Pennies for Pay Write a program that calculates...Ch. 4 - Sum of Numbers Write a program with a loop that...Ch. 4 - Ocean Levels Assuming the ocean's level is...Ch. 4 - Tuition Increase At one college, the tuition for a...Ch. 4 - Weight Loss If a moderately active person cuts...Ch. 4 - Calculating the Factorial of a Number In...Ch. 4 - Population Write a program that predicts the...Ch. 4 - Prob. 14PECh. 4 - Prob. 15PECh. 4 - Turtle Graphics: Repeating Squares In this...Ch. 4 - Turtle Graphics: Star Pattern Use a loop with the...Ch. 4 - Turtle Graphics: Hypnotic Pattern Use a loop with...Ch. 4 - Turtle Graphics: STOP Sign In this chapter, you...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forward
- Using XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward
- 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forwardHow would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forward
- Simplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 2. Let ẞ 2, m = 6, emin = -3 and emax = 3. Answer the following questions: Compute the minimum of |x| for General and Normalized form (a) Compute the Machine Epsilon value for the General and Denormalized form. If we change the value of emax to 6 then how will it affect the value of maximum scale invariant error for the case of Normalized form? Explain your answer. show answer in pen a Don't use any Al tool nd paper then take pi ctures and sendarrow_forwardCSE330: Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. Now, let's take, ẞ = 2, m = 3, emin = -2 and emax = 3. Based on these, answer the following: (a) (b) (c) (d) What are the maximum/largest numbers that can be stored in the system by these three forms defined above? (express your answer in decimal values) What are the non-negative minimum/smallest numbers that can be stored in the system by the denormalized form? (express your answer in decimal values) How many numbers (both non-negative and negative) can be represented in the above mentioned system using the general form? Explain your answer. Find all the decimal numbers for e = 3 and e = 2 in denormalized form, plot them on a real line and prove that all the numbers are not equally spaced. Write the equally spaced sets for the number line you drew. show your answer in Don't use any Al tool pen…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
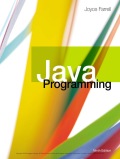
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
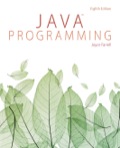
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
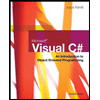
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
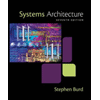
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
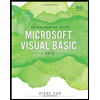
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning