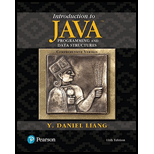
(Geometry: area of a pentagon) Write a
The formula for computing the area of a pentagon is

Geometry: Area of the pentagon
Program Plan:
- Import required packages.
- Declare the main class method “pentagon”.
- In the main method.
- Create an object “in1” for the scanner class.
- Get length from the user.
- Declare the required variables for area of pentagon.
- Calculate the area of the pentagon.
- Display the pentagon.
- In the main method.
The below program reads length and display the area of the pentagon.
Explanation of Solution
Program:
//import the required header files
import java.util.Scanner;
//create a class "pentagon"
public class pentagon
{
//main function
public static void main(String[] args)
{
//Create an object
Scanner in1 = new Scanner(System.in);
//Get length from user
System.out.print("Enter the length from the center to a vertex: ");
//Get the length in "double" type
double r1 = in1.nextDouble();
//Calculate "s1"
double s1 = 2 * r1 * Math.sin(Math.PI / 5);
//Calculate "a1"
double a1 = 5 * s1 * s1 / (4 * Math.tan(Math.PI / 5));
//Display "area"
System.out.println("The area of the pentagon is " +
Math.round(a1 * 100) / 100.0);
}
}
Enter the length from the center to a vertex: 5.5
The area of the pentagon is 71.92
Want to see more full solutions like this?
Chapter 4 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Problem Solving with C++ (10th Edition)
Starting Out with Python (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Concepts Of Programming Languages
Degarmo's Materials And Processes In Manufacturing
Database Concepts (8th Edition)
- (True or False) Seven different positive integers are randomly chosen between 1 and 2022 (including 1 and 2022).There must be a pair of these integers has a difference that is a multiple of 6.arrow_forward(Demonstrate cancellation errors) A cancellation error occurs when you are manipulating a very large number with a very small number. The large number may cancel out the smaller number. For example, the result of 100000000.0 + 0.000000001 is equal to 100000000.0. To avoid cancellation errors and obtain more accurate results, carefully select the order of computation. For example, in computing the following series, you will obtain more accurate results by comput- ing from right to left rather than from left to right: 1 1 1 1+ + 3 + ... 2 п Write a program that compares the results of the summation of the preceding series, computing from left to right and from right to left with n = 50000.arrow_forward(Random Walk Robot) A robot is initially located at position (0, 0) in a grid [−5, 5] × [−5, 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move in and the current position of the robot. Use formatted output to print the direction (Down, Up, Left or Right) in the left. The direction takes 10 characters in total and fill in the field with empty spaces. The statement to print results in such format is given below: cout << setw(10) << left << ‘Down’ << ... ; cout << setw(10) << left << ‘Up’ << ...; If the robot moves back to the original place (0,0), print “Back to the origin!” to the console and stop the program. If it reaches the boundary of the grid, print “Hit the boundary!” to the console and stop the program. A successful run of your code may look like: Due to randomness, your results may have a different trajectory…arrow_forward
- (Random Walk Robot) A robot is initially located at position (0, 0) in a grid [−5, 5] × [−5, 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move in and the current position of the robot. Use formatted output to print the direction (Down, Up, Left or Right) in the left. The direction takes 10 characters in total and fill in the field with empty spaces. The statement to print results in such format is given below: cout << setw(10) << left << ‘Down’ << ... ; cout << setw(10) << left << ‘Up’ << ...; If the robot moves back to the original place (0,0), print “Back to the origin!” to the console and stop the program. If it reaches the boundary of the grid, print “Hit the boundary!” to the console and stop the program. A successful run of your code may look like: Due to randomness, your results may have a different…arrow_forward*Please help in javascript* Summary: Given integer values for red, green, and blue, subtract the gray from each value. Computers represent color by combining the sub-colors red, green, and blue (rgb). Each sub-color's value can range from 0 to 255. Thus (255, 0, 0) is bright red, (130, 0, 130) is a medium purple, (0, 0, 0) is black, (255, 255, 255) is white, and (40, 40, 40) is a dark gray. (130, 50, 130) is a faded purple, due to the (50, 50, 50) gray part. (In other words, equal amounts of red, green, blue yield gray). Given values for red, green, and blue, remove the gray part. Ex: If the input is: 130 50 130 the output is: 80 0 80 import java.util.Scanner; public class LabProgram {public static void main(String[] args) {/* Type your code here. */}}arrow_forward(Bar-Chart Printing Program) One interesting application of computers is drawing graphsand bar charts. Write a program that reads five numbers (each between 1 and 30). For each numberread, your program should print a line containing that number of adjacent asterisks. For example,if your program reads the number seven, it should print *******.arrow_forward
- Find the error(s) in the following code: (6)arrow_forwardPython Programming Part 2 Direction: Use PYTHON to solve this problem. Description: This part tests for two things. The program must identify all resistors in series or in parallel with each other and calculate the equivalent resistance for each group of resistors. Recall that total resistance of resistors in series are added up while the total conductance of those in parallel are added up. Input Format: The first line of the input consists of 1 integer N which is the total number of resistors. The next N lines will consist of 3 strings and an integer R each separated by a space. The first string is the resistor name, the second and third strings are the node names where the resistor terminals are connected, and R is its resistance value. Output Format: One line for each group of resistors in series or parallel. Each line starts with a list of resistors arranged in lexicographical order (see Lexicographic order - Wikipedia) followed by their total resistance rounded off to the nearest…arrow_forwardPlease help answer these two questions in Python. (See attached image) Attach screenshots of your code for clarity.arrow_forward
- (Same-number subsequence) JAVA Class Name: Exercise22_05 Write an O(n) program that prompts the user to enter a sequence of integers ending with 0 and finds the longest subsequence with the same number. Sample Run 1 Enter a series of numbers ending with 0:2 4 4 8 8 8 8 2 4 4 0The longest same number sequence starts at index 3 with 4 values of 8 Sample Run 2 Enter a series of numbers ending with 0: 34 4 5 4 3 5 5 3 2 0 The longest same number sequence starts at index 5 with 2 values of 5arrow_forward(PYTHON) A nutritionist who works for a fitness club helps members by evaluating their diets. As part of her evaluation, she asks members for the number of fat grams and carbohydrate grams that they consumed in a day. Then, she calculates the number of calories that result from the fat, using the following formula: calories from fat = fat grams x 3.9 Next, she calculates the number of calories that result from the carbohydrates, using the following formula: calories from carbs = carb grams x 4 The nutritionist asks you to write a program on *PYTHON* that will make these calculations.arrow_forwarddo in python programming languagearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
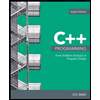