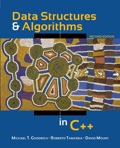
Explanation of Solution
Program code:
//include the required header files
#include <iostream>
#include<time.h>
//use the std namespace
using namespace std;
//Declare n as type of constant
const int n = 10;
//Implementation of prefixAverages1 array
double *prefixAverages1(int X[])
{
//Declare a as integer type
int a;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//assign 0 to a
a = 0;
//Iterate the loop
for (int j = 0; j <= i; j++)
{
//calculate a = a+X[j] value
a = a + X[j];
}
//assign a / (i + 1)
A[i] = a / (i + 1);
}
//return value of the array A
return A;
}
//Implementation of prefixAverages1 array
double *prefixAverages2(int X[])
{
//Declare s and assign 0
int s = 0;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//calculate s = s+X[i] value
s = s + X[i];
//assign s / (i + 1)
A[i] = s / (i + 1);
}
//return A
return A;
}
int main()
{
//Decalre X as type of an array
int X[n];
//Declare differenceValue1, differenceValue2 as type of double
double differenceValue1, differenceValue2;
//Declare timeInseconds1, timeInseconds2 as type of double
double timeInseconds1, timeInseconds2;
//Declare i as type of integer
int i = 0;
//Iterate the loop
while (i <= n)
{
//assign i to X[i]
X[i] = i;
//increment i
i++;
}
//Declare resultimeEntrie1,resultimeEntrie2 as type of double
double *resultimeEntrie1 =0, *resultimeEntrie2 = 0;
// declare clock_t objects as timeEntrie1,timeEntrie2
clock_t timeEntrie1, timeEntrie2;
timeEntrie1 = clock();
//store the return value of prefixAverages1(x)
resultimeEntrie1 = prefixAverages1(X);
//Declare k as integer type and assign 0
int k = 0;
// initialize timeEntrie2
timeEntrie2 = clock();
// get the differenceValueerence of two object values
differenceValue1 = (double)timeEntrie2 - (double)timeEntrie1;
// calculate timeInseconds
timeInseconds1 = differenceValue1 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages1(X) " << endl;
//Display statement
cout<<"For n = " << n << " elements then it take " << timeInseconds1 << " seconds" << endl;
// declare clock_t objects as timeEntrie3,timeEntrie4
clock_t timeEntrie3, timeEntrie4;
timeEntrie3 = clock();
//store the return value of prefixAverages2(x)
resultimeEntrie2 = prefixAverages2(X);
timeEntrie4 = clock();
// calculate the differenceValue of two object values
differenceValue2 = (double)timeEntrie4 - (double)timeEntrie3;
// calculate timeInseconds
timeInseconds2 = differenceValue2 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages2(X)" << endl;
//Display statement
cout<<"\n For n = " << n << " elements then it take " << timeInseconds2 << " seconds" << endl;
return 0;
}
Explanation:
The above program snippet is used to implement “prefixAverages1()” and “prefixAverages2()”. In the code,
- Include the required header files.
- Use the “std” namespace.
- Declare the constant “n”.
- Define the method “prefixAverages1()”.
- Declare the required variables.
- Declare an array “A”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Assign a value “a/(i+1)”.
- Return the value of “A”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Data structures and algorithms in C++
- Question#2: Design and implement a Java program using Abstract Factory and Singleton design patterns. The program displays date and time in one of the following two formats: Format 1: Date: MM/DD/YYYY Time: HH:MM:SS Format 2: Date: DD-MM-YYYY Time: SS,MM,HH The following is how the program works. In the beginning, the program asks the user what display format that she wants. Then the program continuously asks the user to give one of the following commands, and performs the corresponding task. Note that the program gets the current date and time from the system clock (use the appropriate Java date and time operations for this). 'd' display current date 't': display current time 'q': quit the program. • In the program, there should be 2 product hierarchies: "DateObject” and “TimeObject”. Each hierarchy should have format and format2 described above. • Implement the factories as singletons. • Run your code and attach screenshots of the results. • Draw a UML class diagram for the program.arrow_forward#include <linux/module.h> #include <linux/kernel.h> // part 2 #include <linux/sched.h> // part 2 extra #include <linux/hash.h> #include <linux/gcd.h> #include <asm/param.h> #include <linux/jiffies.h> void print_init_PCB(void) { printk(KERN_INFO "init_task pid:%d\n", init_task.pid); printk(KERN_INFO "init_task state:%lu\n", init_task.state); printk(KERN_INFO "init_task flags:%d\n", init_task.flags); printk(KERN_INFO "init_task runtime priority:%d\n", init_task.rt_priority); printk(KERN_INFO "init_task process policy:%d\n", init_task.policy); printk(KERN_INFO "init_task task group id:%d\n", init_task.tgid); } /* This function is called when the module is loaded. */ int simple_init(void) { printk(KERN_INFO "Loading Module\n"); print_init_PCB(); printk(KERN_INFO "Golden Ration Prime = %lu\n", GOLDEN_RATIO_PRIME); printk(KERN_INFO "HZ = %d\n", HZ); printk(KERN_INFO "enter jiffies = %lu\n", jiffies); return 0; } /* This function is called when the…arrow_forwardList at least five Operating Systems you know. What is the difference between the kernel mode and the user mode for the Linux? What is the system-call? Give an example of API in OS that use the system-call. What is cache? Why the CPU has cache? What is the difference between the Static Linking and Dynamic Linking when compiling the code.arrow_forward
- In the GoF book, List interface is defined as follows: interface List { int count(); //return the current number of elements in the list Object get(int index); //return the object at the index in the list Object first(); //return the first object in the list Object last(); //return the last object in the list boolean include(Object obj); //return true is the object in the list void append(Object obj); //append the object to the end of the list void prepend(Object obj); //insert the object to the front of the list void delete(Object obj); //remove the object from the list void deleteLast(); //remove the last element of the list void deleteFirst(); //remove the first element of the list void deleteAll(); //remove all elements of the list (a) Write a class adapter to adapt Java ArrayList to GoF List interface. (b) Write a main program to test your adapters through List interface. (c) Same requirement as (a) and (b), but write an object adapter to adapt Java ArrayList to GoF List…arrow_forwardIn modern packet-switched networks, including the Internet, the source host segments long, application-layer messages (for example, an image or a music file) into smaller packets and sends the packets into the network. The receiver then reassembles the packets back into the original message. We refer to this process as message segmentation. Figure 1.27 (attached) illustrates the end-to-end transport of a message with and without message segmentation. Consider a message that is 106 bits long that is to be sent from source to destination in Figure 1.27. Suppose each link in the figure is 5 Mbps. Ignore propagation, queuing, and processing delays. a. Consider sending the message from source to destination without message segmentation. How long does it take to move the message from the source host to the first packet switch? Keeping in mind that each switch uses store-and-forward packet switching, what is the total time to move the message from source host to destination host? b. Now…arrow_forwardConsider a packet of length L that begins at end system A and travels over three links to a destination end system. These three links are connected by two packet switches. Let di, si, and Ri denote the length, propagation speed, and the transmission rate of link i, for i = 1, 2, 3. The packet switch delays each packet by dproc. Assuming no queuing delays, in terms of di, si, Ri, (i = 1, 2, 3), and L, what is the total end-to-end delay for the packet? Suppose now the packet is 1,500 bytes, the propagation speed on all three links is 2.5 * 10^8 m/s, the transmission rates of all three links are 2.5 Mbps, the packet switch processing delay is 3 msec, the length of the first link is 5,000 km, the length of the second link is 4,000 km, and the length of the last link is 1,000 km. For these values, what is the end-to-end delay?arrow_forward
- how to know the weight to data and data to weight also weight by infomraion gain in rapid miner , between this flow diagram retrieve then selecte attrbuite then set role and split data and decision tree and apply model and peformance ,please show how the operators should be connected:arrow_forwardusing rapid miner how to creat decison trea for all attribute and another one with delete one or more of them also how i know the weight of each attribute and what that mean in impact the resultarrow_forwardQ.1. Architecture performance [10 marks] Answer A certain microprocessor requires either 2, 4, or 6 machine cycles to perform various operations. ⚫ (40+g+f)% require 2 machine cycles, ⚫ (30-g) % require 4 machine cycles, and ⚫ (30-f)% require 6 machine cycles. (a) What is the average number of machine cycles per instruction for this microprocessor? Answer (b) What is the clock rate (machine cycles per second) required for this microprocessor to be a "1000 MIPS" processor? Answer (c) Suppose that 35% of the instructions require retrieving an operand from memory which needs an extra 8 machine cycles. What is the average number of machine cycles per instruction, including the instructions that fetch operands from memory?arrow_forward
- Q.2. Architecture performance [25 marks] Consider two different implementations, M1 and M2, of the same instruction set. M1 has a clock rate of 2 GHz and M2 has a clock rate of 3.3 GHz. There are two classes of instructions with the following CPIs: Class A CPI for M1 CPI for M2 2.f 1.g B 5 3 C 6 4 Note that the dots in 2 fand 1.g indicate decimal points and not multiplication. a) What are the peak MIPS performances for both machines? b) Which implementation is faster, if half the instructions executed in a certain program are from class A, while the rest are divided equally among classes B and C. c) What speedup factor for the execution of class-A instructions would lead to 20% overall speedup? d) What is the maximum possible speedup that can be achieved by only improving the execution of class-A instructions? Explain why. e) What is the clock rate required for microprocessor M1 to be a "1000 MIPS" (not peak MIPS) processor?arrow_forwardPLEASE SOLVE STEP BY STEP WITHOUT ARTIFICIAL INTELLIGENCE OR CHATGPT I don't understand why you use chatgpt, if I wanted to I would do it myself, I need to learn from you, not from being a d amn robot. SOLVE STEP BY STEP I WANT THE DIAGRAM PERFECTLY IN SIMULINKarrow_forwardI need to develop and run a program that prompts the user to enter a positive integer n, and then calculate the value of n factorial n! = multiplication of all integers between 1 and n, and print the value n! on the screen. This is for C*.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
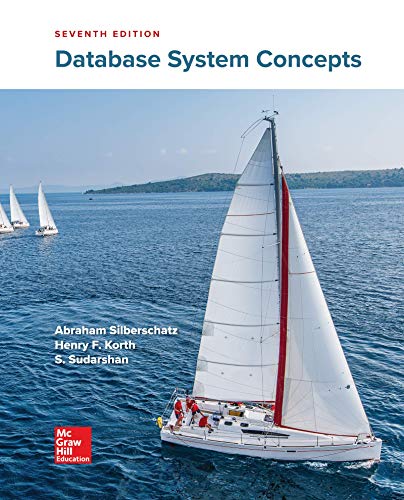
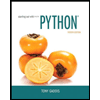
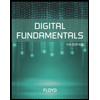
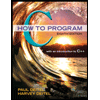
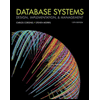
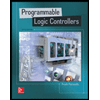