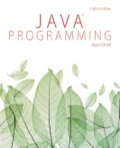
EBK JAVA PROGRAMMING
8th Edition
ISBN: 9781305480537
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 1GZ
Program Plan Intro
Generate random numbers and find out which one is greater
Program plan:
Filename: “Die.java”
- Define the “Die” class
- Declare the required variables.
- Define the constructor
- Set the values
- Define the “generateRandom” method
- Determine the random value and return it
- Define the “getValue” method
- Return the value.
Filename: “TwoDice.java”
- Define the “TwoDice” class
- Define the main method.
- Create the objects for “Die” class
- Declare the variables and call the “getValue” method with the different “Die” objects.
- Display the first and second dice values
- Check “x” is greater than “y” or not.
- If the condition is true, then display “x” is greater.
- Check “x” is less than “y” or not.
- If the condition is true, then display “x” is lesser.
- Otherwise, display both the values are equal.
- Define the main method.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Hands-On Assignments Part II
Assignment 1-5: Querying the DoGood Donor Database
Review the DoGood Donor data by writing and running SQL statements to perform the following
tasks:
1. List each donor who has made a pledge and indicated a single lump sum payment. Include
first name, last name, pledge date, and pledge amount.
2. List each donor who has made a pledge and indicated monthly payments over one year.
Include first name, last name, pledge date, and pledge amount. Also, display the monthly
payment amount. (Equal monthly payments are made for all pledges paid in monthly
payments.)
3. Display an unduplicated list of projects (ID and name) that have pledges committed. Don't
display all projects defined; list only those that have pledges assigned.
4. Display the number of pledges made by each donor. Include the donor ID, first name, last
name, and number of pledges.
5. Display all pledges made before March 8, 2012. Include all column data from the
DD PLEDGE table.
Write a FancyCar class to support basic operations such as drive, add gas, honk horn, and start engine. FancyCar.java is provided with method stubs. Follow each step to gradually complete all methods.
Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main() method includes basic method calls. Add statements in main() as methods are completed to support development mode testing.
Step 0. Declare private fields for miles driven as shown on the odometer (int), gallons of gas in tank (double), miles per gallon or MPG (double), driving capacity (double), and car model (String). Note the provided final variable indicates the gas tank capacity of 14.0 gallons.
Step 1 (2 pts). 1) Complete the default constructor by initializing the odometer to five miles, tank is full of gas, miles per gallon is 24.0, and the model is "Old Clunker". 2)…
Find the error:
daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0]
days_of_week = ['Sunday', 'Monday', 'Tuesday',
'Wednesday', 'Thursday', 'Friday',
'Saturday']
for i in range(7):
daily_sales[i] = float(input('Enter the sales for ' \
+ day_of_week[i] + ': ')
Chapter 4 Solutions
EBK JAVA PROGRAMMING
Ch. 4 - Prob. 1RQCh. 4 - Prob. 2RQCh. 4 - Prob. 3RQCh. 4 - Prob. 4RQCh. 4 - Prob. 5RQCh. 4 - Prob. 6RQCh. 4 - Prob. 7RQCh. 4 - Prob. 8RQCh. 4 - Prob. 9RQCh. 4 - Prob. 10RQ
Ch. 4 - Prob. 11RQCh. 4 - Prob. 12RQCh. 4 - Prob. 13RQCh. 4 - Prob. 14RQCh. 4 - Prob. 15RQCh. 4 - Prob. 16RQCh. 4 - Prob. 17RQCh. 4 - Prob. 18RQCh. 4 - Prob. 19RQCh. 4 - Prob. 20RQCh. 4 - Prob. 1PECh. 4 - Prob. 2PECh. 4 - Prob. 3PECh. 4 - Prob. 4PECh. 4 - Prob. 5PECh. 4 - Prob. 6PECh. 4 - Prob. 7PECh. 4 - Prob. 8PECh. 4 - Prob. 9PECh. 4 - Prob. 10PECh. 4 - Prob. 11PECh. 4 - Prob. 12PECh. 4 - Prob. 1GZCh. 4 - Prob. 2GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Find the error: daily_sales = [0.0, 0,0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': ')arrow_forwardFind the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(6): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': '))arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it and normalize it? Give two references with your answer.arrow_forward
- What are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Consider that the database offline is not allowed since people are connected to it and personal data might be bridged and not secured. Provide three refernces with you answer.arrow_forwardShould software manufacturers should be tolerant of the practice of software piracy in third-world countries to allow these countries an opportunity to move more quickly into the information age? Why or why not?arrow_forwardI would like to know about the features of Advanced Threat Protection (ATP), AMD-V, and domain name space (DNS).arrow_forward
- I need help to resolve the following activityarrow_forwardModern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
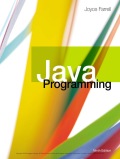
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
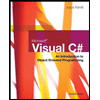
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
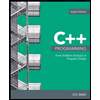
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
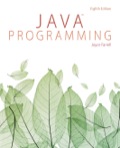
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
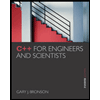
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY