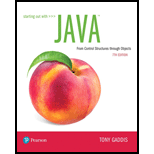
What will the following
public class Checkpoint
{
public static void main (String [] args)
{
int funny = 7, serious = 15;
funny = serious % 2;
if (funny != 1)
{
funny = 0;
serious = 0;
}
else if (funny == 2)
{
funny = 10;
serious = 10;
}
else
{
funny = 1;
serious = 1;
}
System.out.println(funny + ” ” + serious};
}
}

Trending nowThis is a popular solution!
Learn your wayIncludes step-by-step video

Chapter 3 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out With Visual Basic (8th Edition)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Introduction To Programming Using Visual Basic (11th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
- Compare the security services provided by a digital signature (DS) with those of a message authentication code (MAC). Assume that Oscar can observe all messages sent between Rina and Naseem. Oscar has no knowledge of any keys but the public one, in the case of DS. State whether DS and MAC protect against each attack and, if they do, how. The value auth(x) is computed with a DS or a MAC algorithm. In each scenario, assume the message M = x#####auth(x). (Message integrity) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message M, and then sends M in cleartext to Naseem. Oscar intercepts the message and replaces “Mark” with “Oscar.” Will Naseem detect this in the case of either DS or MAC? If yes, how will Naseem detect it? If not, why? (Replay) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message…arrow_forwardI need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forward
- I would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward
- 3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forward
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
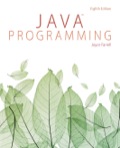
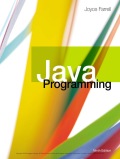
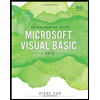
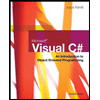
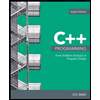