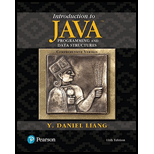
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 30.7, Problem 30.7.1CP
Explanation of Solution
Purpose of given code:
Purpose of given code is to print the resultant values of given code using aggregate “Collectors” and “Comparator”.
Explanation:
- The array values of variable “values” are printed with their occurrence...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Capsim Team PowerPoint Presentations - Slide Title: Key LearningsWhat were the key learnings that you discovered as a team through your Capsim simulation?
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 30 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 30.1 - Prob. 30.1.1CPCh. 30.2 - Prob. 30.2.1CPCh. 30.2 - Prob. 30.2.2CPCh. 30.2 - Prob. 30.2.3CPCh. 30.2 - Prob. 30.2.4CPCh. 30.3 - Prob. 30.3.1CPCh. 30.3 - Prob. 30.3.2CPCh. 30.3 - Prob. 30.3.3CPCh. 30.3 - Prob. 30.3.4CPCh. 30.3 - Given an array names in Listing 30.1, write the...
Ch. 30.4 - Prob. 30.4.1CPCh. 30.4 - How do you create a parallel stream?Ch. 30.4 - Prob. 30.4.3CPCh. 30.4 - Prob. 30.4.4CPCh. 30.4 - Prob. 30.4.5CPCh. 30.4 - Write a statement to obtain an array of 1000...Ch. 30.5 - Prob. 30.5.1CPCh. 30.5 - Prob. 30.5.2CPCh. 30.5 - Prob. 30.5.3CPCh. 30.5 - Prob. 30.5.4CPCh. 30.6 - Prob. 30.6.1CPCh. 30.7 - Prob. 30.7.1CPCh. 30.8 - Can the following code be used to replace line 19...Ch. 30.8 - Prob. 30.8.2CPCh. 30.8 - Prob. 30.8.3CPCh. 30.8 - Prob. 30.8.4CPCh. 30.8 - Write the code to obtain a one-dimensional array...Ch. 30 - Prob. 30.1PECh. 30 - Prob. 30.2PECh. 30 - Prob. 30.3PECh. 30 - (Print distinct numbers) Rewrite Programming...Ch. 30 - Prob. 30.5PECh. 30 - Prob. 30.6PECh. 30 - Prob. 30.7PECh. 30 - Prob. 30.8PECh. 30 - Prob. 30.9PECh. 30 - Prob. 30.10PECh. 30 - Prob. 30.11PECh. 30 - (Sum the digits in an integer) Rewrite Programming...Ch. 30 - (Count the letters in a string) Rewrite...Ch. 30 - Prob. 30.14PECh. 30 - (Display words in ascending alphabetical order)...Ch. 30 - Prob. 30.16PECh. 30 - Prob. 30.17PECh. 30 - (Count the occurrences of words in a text file)...Ch. 30 - (Summary information) Suppose the file test.txt...
Knowledge Booster
Similar questions
- Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.arrow_forward2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forward
- hello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forward
- Hello please look at the attached picture. I need an detailed explanation of the architecturearrow_forwardInformation Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
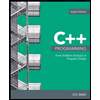
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
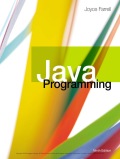
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
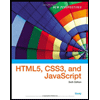
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
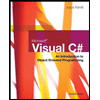
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,