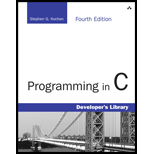
Concept explainers
Write a
( – –) – –)

Explanation of Solution
Program:
The following program will evaluate the given expression.
//include the header file
#include <stdio.h>
//definition of main method
int main (void)
{
//declare the variable
float ans;
//calculate the ans
ans = (3.31e-8f * 2.01e-7f) / (7.16e-6f + 2.01e-8f);
//display the result
printf ("The answer is %e\n", ans);
//return statement
return 0;
}
Explanation:
In the above program, declare the required header file. Inside the main method, declare the variable. Then calculate the “ans” value and print the result on the output screen.
The answer is 9.266027e-10
Want to see more full solutions like this?
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with C++: Early Objects
Starting Out With Visual Basic (7th Edition)
- Please take screenshots of the stops. (Science: calculating energy) Write a program that calculates the energy needed to heat water from an initial temperature to a final temperature. Your program should prompt the user to enter the amount of water in kilograms and the initial and final temperatures of the water. The formula to compute the energy is Q = M * (finalTemperature – initialTemperature) * 4184 where M is the weight of water in kilograms, temperatures are in degrees Celsius, and energy Q is measured in joules.arrow_forwardCreate a program which gets an integer as input and reverses it. Use only arithmetic operations. For example: 12345 -> 54321. (in C language)arrow_forward(Python) Please write a program that creates steps. You are expected to take in a single positive integer which will be used as the number of steps in your staircase. The program only accepts integers greater than 0 and less than 500. If 0 is entered a message stating "Your staircase has no steps." and if the user enters a value greater than or equal to 500, a message stating "I can't build a staircase that tall." For all other values not within the valid range or not integers an "Invalid staircase size provided." will be displayed to the user. The program will always run only once for each user input. One thing to note, the messages should be the return string from your printSteps() function and printed from the calling function. Additional Requirements: The very first step in the staircase will be left-aligned and for each subsequent level, the step will move above and to the right of the prior step. There are no spaces after the right of any of the steps. The bottom-most row ends…arrow_forward
- 3. Write a Java program that uses a for iteration statement in order to generate and print the sum of numbers from 1 to 1,000 to the output. (2 points)arrow_forward===========Answer question below:=========== - Write program in Java. (Convert infix to postfix) Note: Postfix notation is a way of writing expression without using parentheses. For example, the expression ( 11 + 12 ) * 13 would be written as 11 12 + 13 * Assume that ALWAYS there is a space between operands and operators in the input expression. Use two stacks, one to store the operands and one to store the operators. Your program only accpets following operators : ( ) + - / * Write a method to converts an infix expression into a postfix expression using the following method: String infixToPostfix(String expression) For example, the method should convert the infix expression ( 13 + 25 ) * 34 to 13 25 + 34 * and 20 * ( 10 + 30 ) to 20 10 30 + *. - solution.java: import java.util.*; import java.lang.*; import java.io.*; class InfixToPostfix { public String infixToPostfix(String expression) { } } class DriverMain { public static void main(String args[]) { Scanner input…arrow_forwarddo it fastarrow_forward
- Use C# (:Write an app that reads in a 5 digit number and determines if it is a palindrome. A palindrome is a number that reads the same backward as it does forward, for instance: 12321, 44444 or 22722. If the number inputted is not 5 digits long, display an error message and allow the user to input another number.arrow_forward(Display a pattern) Write a program that displays the following pattern:JJ aaa v vaaaJ J aa v v a aJ aaaa v aaaaarrow_forwardprovide answer with full explanation ( only type ) java pleasearrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
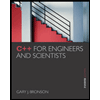
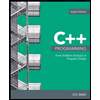