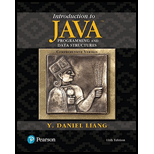
(Algebra: solve quadratic equations) The two roots of a quadratic equation ax2 + bx + c = 0 can be obtained using the following formula:
b2 − 4ac is called the discriminant of the quadratic equation. If it is positive, the equation has two real roots. If it is zero, the equation has one root. If it is negative, the equation has no real roots.
Write a
Note you can use Math. pow(x, 0.5) to compute

Algebra: solve quadratic equation
Program Plan:
- Import the required packages.
- Create a class Exercise
- Define the main method.
- Define the scanner input and prompt user to enter the value of a,b,c.
- Get the input.
- Calculate the discriminant value.
- Condition to validate the discriminant value.
- After validation the value gets of the root gets calculated based on the condition.
- Display the result of roots.
The below program is used to solve the quadratic equation:
Explanation of Solution
Program:
//import the required packages
import java.util.Scanner;
//define the class exercise
public class Exercise
{
public static void main(String[] args)
{
//scanner input
Scanner input = new Scanner(System.in);
/*prompt user to enter the value of the a,b and c*/
System.out.print("Enter a, b, c: ");
//get value of a
double a = input.nextDouble();
//get value of b
double b = input.nextDouble();
//get value of c
double c = input.nextDouble();
//equation to calculate the discriminant
double discriminant = b * b - 4 * a * c;
//condition to validate the discriminant value
if (discriminant < 0)
{
//display result
System.out.println("The equation has no real roots");
}
//condition to validate the discriminant value
else if (discriminant == 0)
{
//equation to calculate the root value
double r1 = -b / (2 * a);
//display result
System.out.println("The equation has one root " + r1);
}
//condition to validate the discriminant value
else
{
// (discriminant > 0)
//equation to calculate the root value
double r1 = (-b + Math.pow(discriminant, 0.5)) / (2 * a);
//equation to calculate the root value
double r2 = (-b - Math.pow(discriminant, 0.5)) / (2 * a);
//display result
System.out.println("The equation has two roots " + r1 + " and " + r2);
}
}
}
Enter a, b, c: 1
2.0
1
The equation has one root -1.0
Additional Output 1:
Enter a, b, c: 1.0
3
1
The equation has two roots -0.3819660112501051 and -2.618033988749895
Additional Output 2:
Enter a, b, c: 1
2
3
The equation has no real roots
Want to see more full solutions like this?
Chapter 3 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (8th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Concepts Of Programming Languages
Problem Solving with C++ (10th Edition)
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
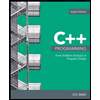
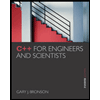
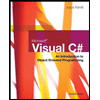
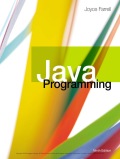